How to Invert an Image Using OpenCV Module in Python
- Inverting Images
-
Invert Images Using
bitwise_not()
Method in Python -
Invert Images Using
numpy.invert()
Method in Python
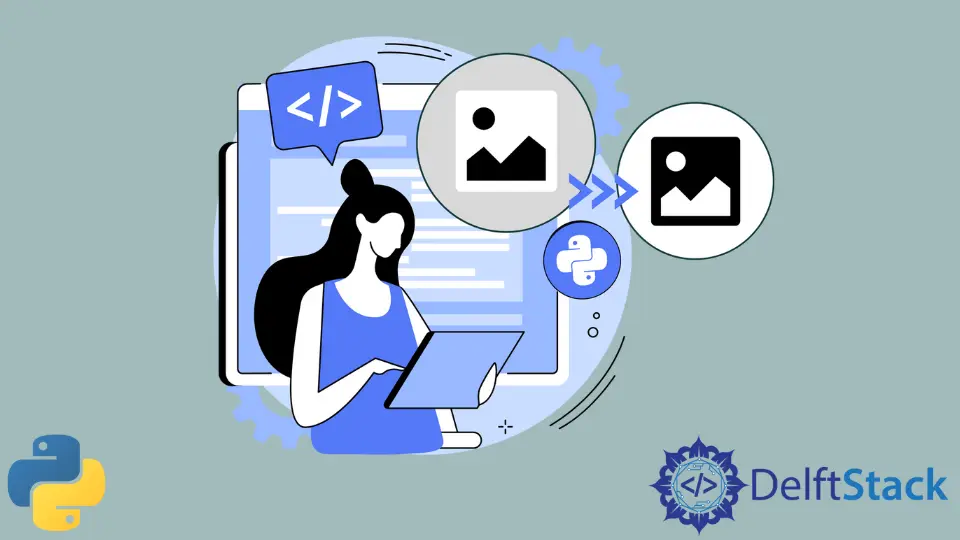
OpenCV or Open Source Computer Vision Library is a real-time computer vision library used for image processing and machine learning. It is written in C/C++ and is available for many programming languages such as C++, Python, and Java. In Python, generally, OpenCV is used along with NumPy, a Python-based library for matrices, multi-dimensional arrays, and mathematical computation.
Images are represented using NumPy multi-dimensional arrays and are processed using OpenCV. Using the OpenCV module, one can perform many operations over images such as flipping, scaling, rotating, mirroring, changing colors, inverting colors, etc. In this article, we will learn how to invert images using the OpenCV module.
Inverting Images
Images are represented using RGB or Red Green Blue values. Each can take up an integer value between 0
and 255
(both included). For example, a red color is represent using (255, 0, 0)
, white with (255, 255, 255)
, black with (0, 0, 0)
, etc.
Inverting an image means reversing the colors on the image. For example, the inverted color for red color will be (0, 255, 255)
. Note that 0
became 255
and 255
became 0
. This means that inverting an image is essentially subtracting the old RGB values from 255
.
New_Value = 255 - Old_Value
Original image:
Inverted image:
Invert Images Using bitwise_not()
Method in Python
OpenCV has a bitwise_not()
method which performs bit-wise NOT operation. We can use this function to invert an image. Refer to the following code. It considers that you have an image by the name of image.png
in your working directory.
import cv2
image = cv2.imread("image.png", 0)
inverted_image = cv2.bitwise_not(image)
cv2.imwrite("inverted.jpg", inverted)
cv2.imshow("Original Image", image)
cv2.imshow("Inverted Image", inverted_image)
This program will first load an image, invert it and save it in the working directory. After that, it will show both the original and the inverted images.
Invert Images Using numpy.invert()
Method in Python
NumPy has an invert()
method, which performs bit-wise inversion or bit-wise NOT operation. The following code shows how we can use this method to perform the inversion.
import cv2
import numpy as np
image = cv2.imread("image.png", 0)
inverted_image = np.invert(image)
cv2.imwrite("inverted.jpg", inverted)
cv2.imshow("Original Image", image)
cv2.imshow("Inverted Image", inverted_image)
To learn more about the
invert()
method, refer here