How to Use the imshow() Function From OpenCV in Python
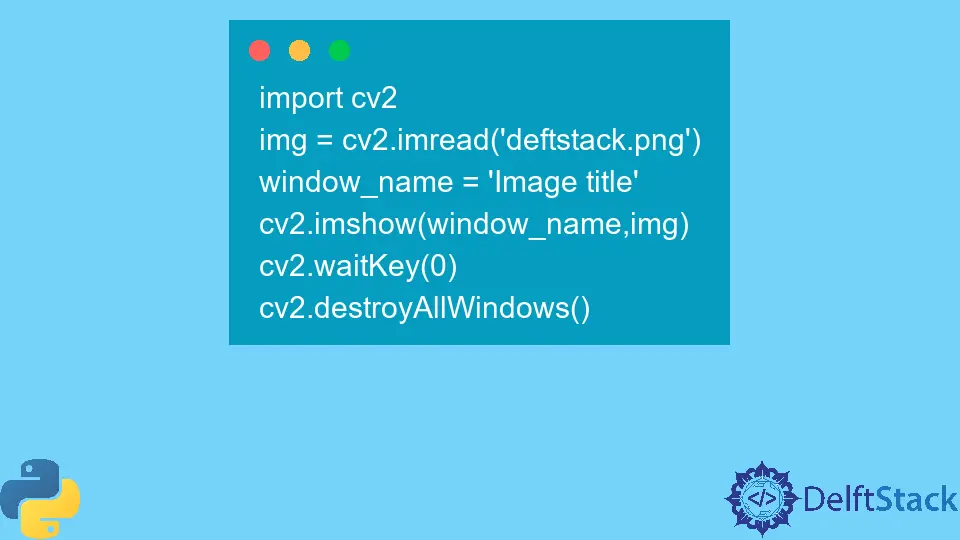
In Python, the OpenCV library is an open-source library that provides functionalities and objects to process images for computer vision in AI. It offers a set of functions to read and process images efficiently.
This tutorial will demonstrate using this library’s imshow()
function.
Use the imshow()
Function From the OpenCV Library in Python
The cv2.imshow()
function can display an image in a new window. The created window will adjust automatically to fit the image.
The image to be displayed needs to be provided within the function and has to be a numpy.ndarray
object. Such objects can be created while readings images using the cv2.imread()
function.
In the following example, we will display an image using the cv2.imshow()
function.
Example code:
import cv2
img = cv2.imread("deftstack.png")
window_name = "Image title"
cv2.imshow(window_name, img)
cv2.waitKey(0)
cv2.destroyAllWindows()
Output:
In the above example code, we first read an image using the cv2.imread()
function and stored it in an object named img
. This object is passed to the cv2.imshow()
, and the image gets displayed in a window.
A parameter is used within the function called window_name
; this is optional. This provides a title to the window in which the image is displayed.
We also used a few other functions from the OpenCV library.
The cv2.waitKey()
function prevents the window from closing; the Python interpreter will close the window automatically if this function is not used. We wait for the user to press any key before closing with this function.
We also use the cv2.destroyAllWindows()
at the end, closing all open windows as the program ends.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn