OpenCV Histogram Equalization
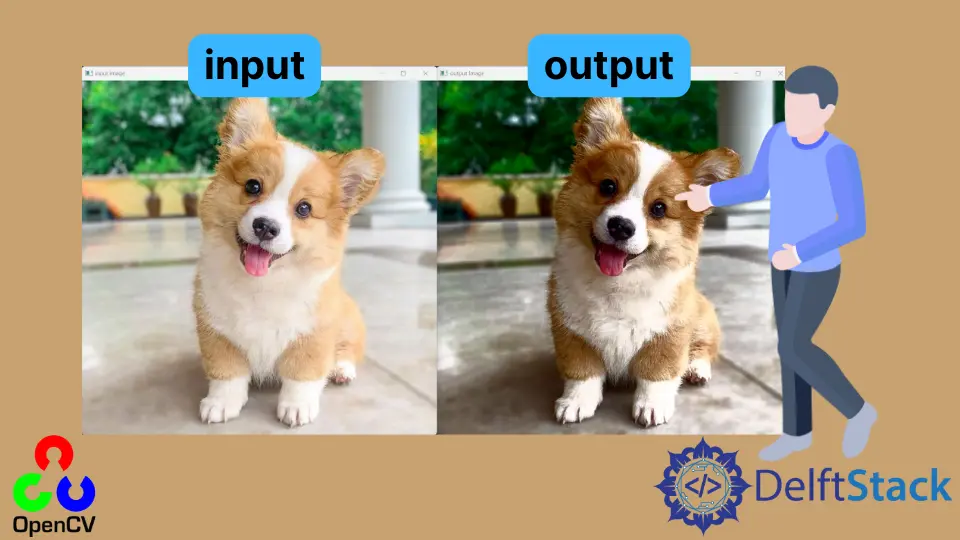
This tutorial will discuss finding the histogram equalization of a colored image using the equalizeHist()
function of OpenCV.
Use equalizeHist()
Function of OpenCV to Find the Histogram Equalization of Colored Images
Histogram equalization is used to increase the intensity level or contrast of images. Histogram equalization will make the bright areas brighter and the dark areas darker.
A grayscale image only contains the brightness value so that we can pass it directly in the equalizeHist()
function to find the histogram equalization of the image.
A color image is mostly in RGB color space, representing the intensity value of red, green, and blue colors instead of brightness value.
So we cannot directly pass the colored image inside the equalizeHist()
function to find the histogram equalization of the image.
We have to convert the image to another color space in which there is a separate channel for the brightness value like HSV
, YCbCr
, and YUV
.
After changing the image’s color space, we can pass the channel which contains the brightness value inside the equalizeHist()
function to find the histogram equalization of the given image.
To get the desired result, we have to replace the brightness value channel with the output from the equalizeHist()
function. After that, we can convert the image back to the original color space.
We can change the color space of an image using the cvtColor()
function of OpenCV.
For example, let’s find the histogram equalization of a colored image.
See the code below.
import cv2
import numpy as np
img = cv2.imread("test.png")
img_yuv = cv2.cvtColor(img, cv2.COLOR_BGR2YUV)
img_yuv[:, :, 0] = cv2.equalizeHist(img_yuv[:, :, 0])
img_output = cv2.cvtColor(img_yuv, cv2.COLOR_YUV2BGR)
cv2.imshow("input image", img)
cv2.imshow("output Image", img_output)
cv2.waitKey(0)
Output:
In the above code, we only find the histogram equalization of the Y channel of the YUV color space image.
We can also use the HSV
and YCbCr
color space to find the histogram equalization.