How to Count Occurrences of a Character in a String in Python
-
How to Count the Occurrences of a Character in a String Using the
collections
ModuleCounter
Class - How to Count the Occurrences of a Character in a String Using the NumPy Library
-
How to Count the Occurrences of a Character in a String Using a
for
Loop -
How to Count the Occurrences of a Character in a String Using
str.count
- How to Count the Occurrences of a Character in a String Using List Comprehension
- How to Count the Occurrences of a Character in a String Using Regular Expressions
- Faq
- Conclusion
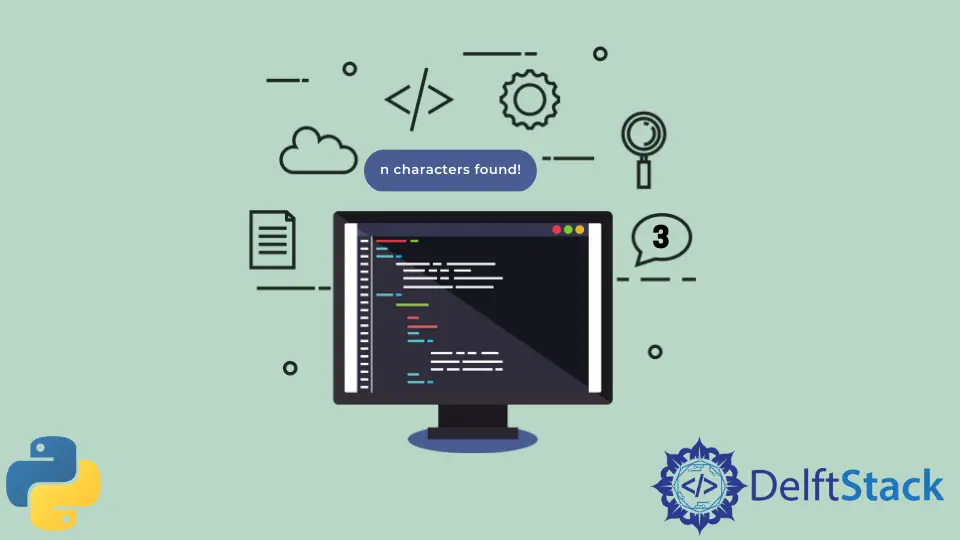
In Python programming, mastering the art of manipulating strings is a fundamental skill. One common task is tallying the occurrences of a specific character within a given string—an essential operation with diverse applications.
This guide delves into the intricacies of counting characters in a Python string, offering a concise yet comprehensive exploration. Whether you’re a beginner seeking to enhance your foundational Python skills or an experienced developer aiming to refine your string manipulation expertise, understanding how to systematically count occurrences provides a valuable tool in your programming arsenal.
How to Count the Occurrences of a Character in a String Using the collections
Module Counter
Class
The collections
module in Python provides specialized data structures that are alternatives to the built-in types like lists, tuples, sets, and dictionaries. These data structures are designed to be efficient and provide additional functionality beyond the basic data types.
A key component of this module is the Counter
class. This class can be used to count the occurrences of elements in a collection, including characters present in a string.
Syntax:
from collections import Counter
counter_obj = collections.Counter(iterable or mapping)
Parameters:
iterable or mapping
(optional): You can initialize aCounter
object either with aniterable
(e.g., a list or a string) or amapping
(e.g., a dictionary). If you don’t provide any argument, an empty counter is created.
Below is a sample Python program of how you can use the Counter
class to count the occurrences of a character in a Python string.
Code Input:
from collections import Counter
def count_characters(input_string, target_character):
# Use Counter to count occurrences of each character in the string
char_count = Counter(input_string)
# Get the count of the target character
target_count = char_count[target_character]
return target_count
# Example usage
input_string = "hello, world!"
target_character = "l"
print(f"Input String: {input_string}")
result = count_characters(input_string, target_character)
print(f"The character '{target_character}' appears {result} times in the string.")
In this example, we created a Counter
object by passing the string to it. The Counter
will automatically count the occurrences of each character in the input_string
.
The result is stored in the char_count
dictionary, where keys are characters and values are their respective counts. The count of the target_character
is then retrieved and printed.
To get a better understanding of this method, adjust the input_string
and target_character
variables to suit your specific use case.
Code Output:
The output of the code successfully indicates how many times the specified target character (l
) appears in the input string (hello, world!
).
How to Count the Occurrences of a Character in a String Using the NumPy Library
NumPy, a powerful library in Python for numerical computing, offers a variety of functions for array manipulation and data analysis.
While its primary focus is on numerical operations, NumPy can also be ingeniously employed to count the occurrences of a character in a Python string. Let’s see how we can utilize NumPy to efficiently count characters in a Python string.
Before getting started, ensure that you have NumPy installed. If you haven’t already installed it, you can do so using the following command:
pip install numpy
Use the unique
Function in NumPy
The NumPy library provides a unique
function that can be used to find unique elements in an array. Note that it is not directly designed to count characters. Still, you can use it along with additional functions to achieve the desired result.
Code Input:
import numpy as np
def count_characters_numpy_unique(input_string, target_character):
# Convert the string to a numpy array of characters
char_array = np.array(list(input_string))
# Use NumPy's unique function to get unique elements and their counts
unique_elements, counts = np.unique(char_array, return_counts=True)
# Find the index of the target character in unique_elements
target_index = np.where(unique_elements == target_character)[0]
# If the target character is present, get its count; otherwise, the count is 0
target_count = counts[target_index] if target_index.size > 0 else 0
return target_count
# Example usage
input_string = "hello, world!"
target_character = "l"
result = count_characters_numpy_unique(input_string, target_character)
print(f"The character '{target_character}' appears {result} times in the string.")
In this example, the np.unique
function is used to get unique elements (unique_elements
) and their counts (counts
) from the char_array
.
Then, np.where
is used to find the index of the target_character
in the unique_elements
array. Finally, the total count of the target character is obtained from the corresponding index in the counts
array.
While this approach works, it’s important to note that using the collections
module, as shown in the first example, is generally more straightforward and efficient for counting occurrences of elements in a collection like a string. The Numpy library is more commonly used for numerical operations on arrays.
Code Output:
The code output indicates how many times the specified target character (l
) appears in the input string (hello, world!
) using the NumPy and unique
approach.
Use the count_nonzero
Function in NumPy
The NumPy library also provides the count_nonzero
function, which counts the number of non-zero elements in an array. While this function is not specifically designed for counting occurrences of characters, it can be utilized along with Boolean indexing to achieve the desired result.
Code Input:
import numpy as np
def count_characters_numpy_nonzero(input_string, target_character):
# Convert the string to a numpy array of characters
char_array = np.array(list(input_string))
# Use NumPy's count_nonzero function to count occurrences of the target character
target_count = np.count_nonzero(char_array == target_character)
return target_count
# Example usage
input_string = "hello, world!"
target_character = "l"
result = count_characters_numpy_nonzero(input_string, target_character)
print(f"The character '{target_character}' appears {result} times in the string.")
In this example, the np.count_nonzero
function is used with Boolean indexing (char_array == target_character
) to count the occurrences of the target_character
. The result is then printed.
Note that this approach is more concise compared to the previous np.unique
method, but it relies on the fact that the target character is not equal to zero (assuming a character with a count of zero would not be present in the string). The collections
module, as shown in the first example, remains a more straightforward and efficient choice for counting occurrences of elements in a collection like a string.
Code Output:
The output indicates how many times the specified target character (l
) appears in the input string (hello, world!
) using NumPy’s count_nonzero
approach. In this case, the result is 3
, as l
appears three times in the string.
How to Count the Occurrences of a Character in a String Using a for
Loop
We can also use a for
loop to iterate through each character in the input string and count the occurrences of a specified character (target_character
). The count is incremented each time the loop encounters the specified character.
Syntax:
def count_characters_with_loop(input_string, target_character):
count = 0
for char in input_string:
if char == target_character:
count += 1
return count
Parameters:
input_string
: The input string in which you want to count occurrences.target_character
: The character whose occurrences you want to count.
Code Input:
def count_characters_with_loop(input_string, target_character):
count = 0
for char in input_string:
if char == target_character:
count += 1
return count
input_string = "hello, world!"
target_character = "l"
result = count_characters_with_loop(input_string, target_character)
print(f"String Input: {input_string}")
print(f"The character '{target_character}' appears {result} times in the string.")
Here, a function named count_characters_with_loop
is defined with two parameters: input_string
(representing the string in which occurrences are to be counted) and target_character
(representing the character whose occurrences are being counted).
Inside the function, a counter, count
variable, is initialized to zero. The function then employs a for
loop to iterate through each character in the input string (input_string
).
Within the loop, there is an if
statement that checks whether the current character (char
) is equal to the specified target character (target_character
). If this condition is true, indicating a match, the count
variable is incremented by 1.
This process continues for each character in the input string and returns the total count of occurrences.
In the subsequent lines outside the function, the script provides an example usage of hello, world!
and sets the target_character
variable to l
. It then calls the count_characters_with_loop
function with these parameters and stores the result in the result
variable.
Code Output:
The output indicates that the character l
appears three times in the input string hello, world!
.
How to Count the Occurrences of a Character in a String Using str.count
To count characters in a Python string using the str.count
method, you can directly call this method on the string. The str.count
method takes one argument, either a character or a substring, and returns the number of non-overlapping occurrences of a substring in the given string.
Syntax:
result = input_string.count(target_character)
Parameters:
input_string
: The string in which you want to count occurrencestarget_character
: The character you want to count.
Below is a code example using the str.count
method to count the occurrences of the character in a string.
Code Input:
input_string = "hello, world!"
target_character = "l"
result = input_string.count(target_character)
print(f"String Input: {input_string}")
print(f"The character '{target_character}' appears {result} times in the string.")
The input_string.count(target_character)
calls the count
method on the input_string
and counts the occurrences of the target_character
(l
). The print(f"The character '{target_character}' appears {result} times in the string.")
prints the result, indicating how many times the specified target character (l
) appears in the input string.
Code Output:
In this example, the str.count
method provides a concise way to count occurrences without the need for a loop or additional functions.
How to Count the Occurrences of a Character in a String Using List Comprehension
To count the occurrences of a character in a string using list comprehension, you can create a list containing only the occurrences of the target character and then find the length of that list. To give you a better understanding, below is an example using the same string hello, world!
and counting the occurrences of the character l
.
Code Input:
input_string = "hello, world!"
target_character = "l"
occurrences = [char for char in input_string if char == target_character]
result = len(occurrences)
print(f"String Input: {input_string}")
print(f"The character '{target_character}' appears {result} times in the string.")
[char for char in input_string if char == target_character]
is a list comprehension that creates a list containing only the characters that match the target character (l
).
The result = len(occurrences)
line calculates the length of the list, which represents the number of occurrences of the target character.
Then, the print(f"The character '{target_character}' appears {result} times in the string.")
prints the result, indicating how many times the specified target character (l
) appears in the input string.
Code Output:
List comprehension provides a concise way to filter elements based on a condition, and in this case, it is used to count occurrences of a specific character in a string.
How to Count the Occurrences of a Character in a String Using Regular Expressions
Regular expressions, commonly known as regex, are sequences of characters that define a search pattern. In Python, the re
module provides support for regular expressions.
The basic syntax for counting occurrences of a character involves using the re.findall()
function along with the character or pattern to search for.
import re
def count_occurrences(string, char):
occurrences = re.findall(char, string)
return len(occurrences)
In this snippet, re.findall(char, string)
searches for all occurrences of the specified character (char
) in the given string (string
). The result is a list of matches, and len(occurrences)
returns the count of these matches.
Code Input:
import re
def count_occurrences(string, char):
occurrences = re.findall(char, string)
return len(occurrences)
# Example Usage
string = "hello world"
char_to_count = "l"
result = count_occurrences(string, char_to_count)
print(f"The character '{char_to_count}' appears {result} times in the string.")
In the provided code, we start by importing the re
module. The count_occurrences
function takes two parameters: string
(the input string) and char
(the character we want to count).
Inside the function, re.findall(char, string)
searches for all the occurrences of the specified character in the string. The result is stored in the occurrences
variable, which is a list of matches. The total count of occurrences in the entire string is then obtained using len(occurrences)
.
The example usage section demonstrates counting all the occurrences of the character l
in the string "hello world"
and prints the result.
Code Output:
This output confirms that the regular expression-based approach successfully counted the occurrences of the specified character in the given string. Regular expressions provide a flexible and powerful solution for various string manipulation tasks, including counting characters of a string or a specific substring.
Faq
Q1: How Do I Count the Occurrences of a Specific Character in a String Using Built-In Methods in Python?
A1: You can use the count
method of a string to directly count the occurrences of a specific character. For example: result = input_string.count(target_character)
.
Q2: What’s an Alternative Approach to Counting Character Occurrences Without Using the count
Method?
A2: You can use list comprehension to create a list of occurrences and then find its length. Example: occurrences = [char for char in input_string if char == target_character]; result = len(occurrences)
.
Q3: Is There a Module in Python That Provides a Specialized Tool for Counting Occurrences of Elements in a Collection, Including Characters in a String?
A3: Yes, the collections
module provides the Counter
class, which is specifically designed for counting occurrences of elements in a collection like a string.
Q4: What’s a Common Method for Counting Occurrences if I Want More Detailed Statistics About Characters in a String?
A4: The collections
module is often used for more advanced statistics. For instance, using Counter(input_string).most_common()
will provide a list of tuples, each containing a character and its count, sorted in descending order of frequency.
Conclusion
In conclusion, Python provides several efficient methods to count the number of occurrences of a character in a string. From the straightforward use of the collections
module’s Counter
class to the versatility of NumPy, the simplicity of a for
loop, the built-in convenience of the str.count
method, the conciseness of list comprehension, to the flexibility of regular expressions, you have an array of tools at your disposal.
When deciding which method to employ, it’s essential to consider the specific requirements of your task. For straightforward character counting, the str.count
method and collections.Counter
provide elegant solutions.
If you’re working with numerical data or larger datasets, NumPy offers a robust alternative. List comprehension and regular expressions cater to those who prefer concise and flexible solutions.
Ultimately, the choice between these methods depends on factors such as readability, simplicity, performance, and the specific characteristics of the data being processed. To further improve your Python skills, try this tutorial about the different methods to find a string in a file in Python.