Python Tutorial - For Loop
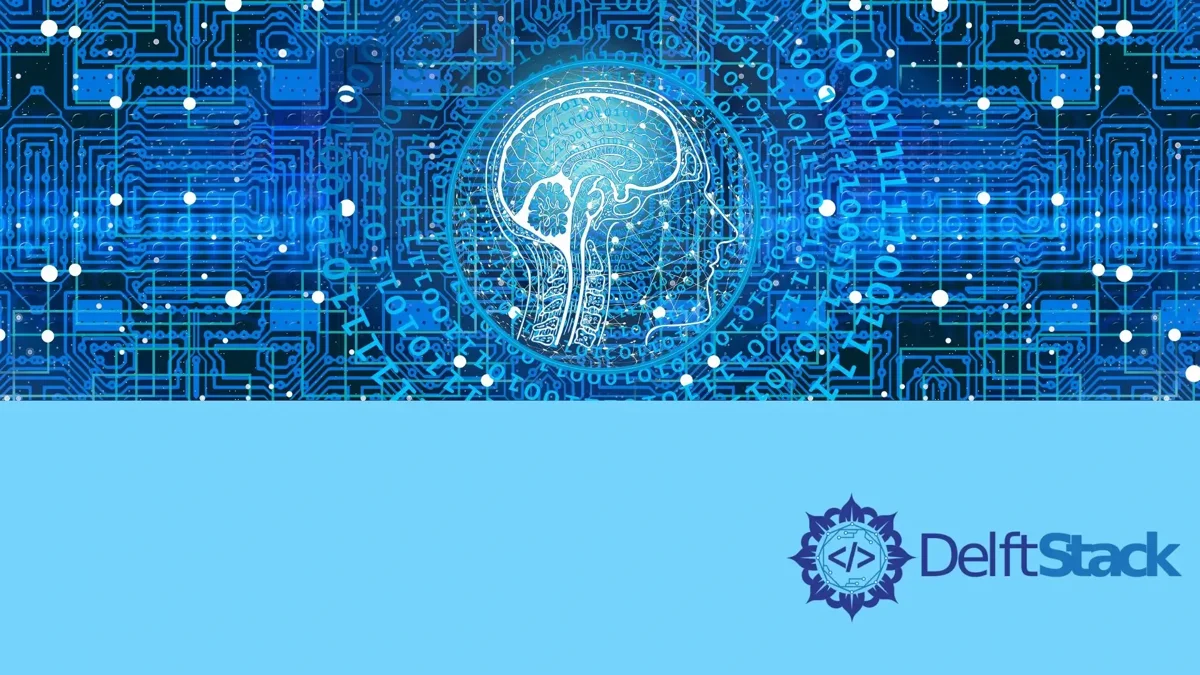
The Python for
loop is used to iterate over the elements of a sequence or other iterable objects.
Python for
Loop
Below is the syntax of for loop in Python:
for val in sequence:
block of statements
Here val
is the variable that is the value of the elements or items of the sequence in each iteration. The loop is terminated after val
reaches the last item of the sequence.
The body of the for
loop is indicated by indentation and not curly braces { }
. The first unindented line will be the end of the for
loop.
for
Loop Example
x = {1, 2, 3, 4, 5, 6, 7, 8, 9}
sum = 0
for i in x:
sum = sum + i
print("Sum of elements of x =", sum)
Sum of elements of x = 45
the Range()
Functions
A sequence of numbers can be generated using the range()
function. The range starts from 0 if no start number is assigned. When you write range(10)
, it will generate numbers from 0 to 9.
The following is the syntax of range()
function:
range(start, stop, step size)
start
and stop
are the starting and ending points and step size
describes the interval between each item.
The range()
function actually defines a range and not tells individual items. If you want to see each item in a range you can use list()
function:
print(range(10)) # OUTPUT: range(0, 10)
print(list(range(10))) # OUTPUT: [0, 1, 2, 3, 4, 5, 6, 7, 8, 9]
Range()
With for
Loop
In the following program, range()
function iterates through a sequence using for
loop and through indexing that is by using the len()
function.
l = ["Python", "Java", "C", "Kotlin"]
for i in range(len(l)):
print("Programming Language is:", l[i])
Programming Language is: Python
Programming Language is: Java
Programming Language is: C
Programming Languages is: Kotlin
for
Loop With else
You can use else
with for
loop to break through the loop if there is no break
statement. else
part will be executed after the items in a sequence are ended. else
contains a body in which you can perform tasks which will be executed when the sequence has no items left.
l = [1, 2, 3, 4, 5]
for i in l:
print("Items in list:", i)
else:
print("List is ended")
Items in list: 1
Items in list: 2
Items in list: 3
Items in list: 4
Items in list: 5
List is ended
When there are no items left in list l
, the else
part will be executed and the message is printed.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook