Python Tutorial - While Loop
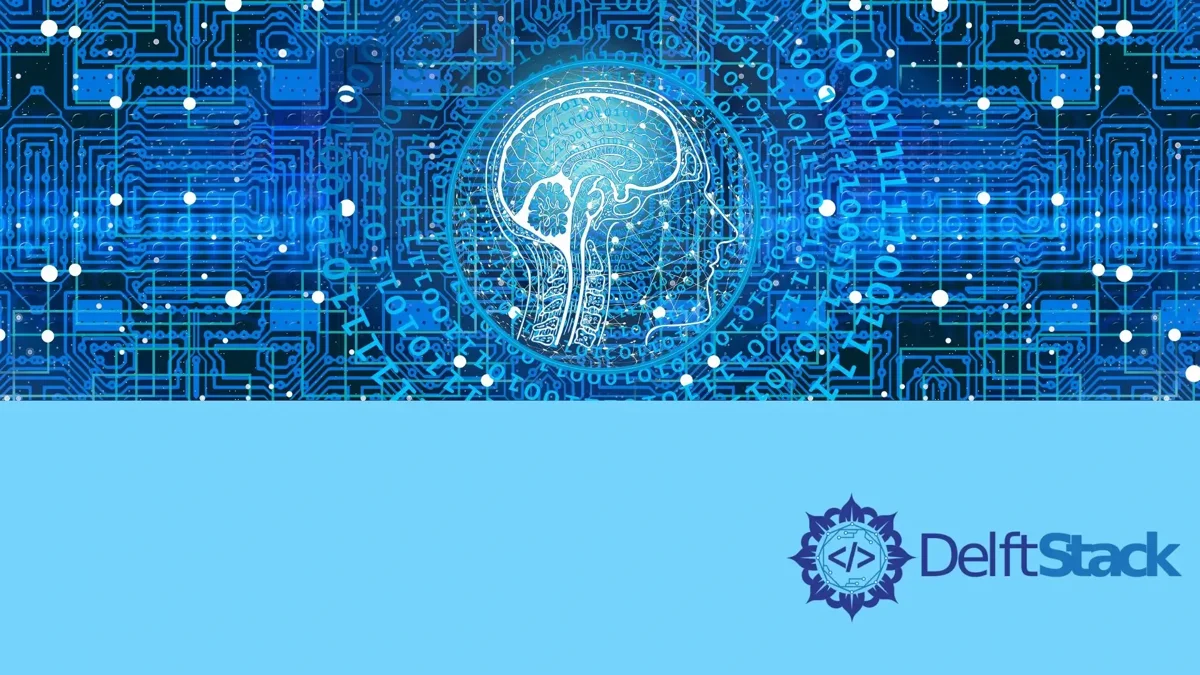
In this section, we will walk you through while
loop that executes a number of statements for a specified number of times.
In a while
loop, if the condition is True
, control enters into the body of while
and statements inside are executed. This process continues until the condition becomes False
.
while
loop is mostly used when you don’t know how many times the statements are going to be executed (total number of iterations).
The following is the syntax of Python while
loop:
while condition:
block of statements
Here, if the condition
is True
, control enters the body of while
and the block of statements is executed. When the condition becomes False
, the iteration will be stopped and the loop is terminated.
while
Loop Example
The following program calculates the sum of the first five even numbers:
sum = 0
i = 0 # initializing counter variable at 0
while i <= 10:
sum = sum + i
i = i + 2 # incrementing counter variable with inter of 2 for even numbers
print("Sum of the first five even numbers =", sum)
Sum of the first five even numbers = 30
Firstly, you need to initialize the value of your counter variable i
. Then you have while
loop containing a condition which tells that the loop should be terminated if i
becomes greater than 10. Then counter variable i
is incremented by adding 2 in each iteration which will generate even numbers as initially i
was zero.
When i
becomes 12, the loop is terminated and the sum
is printed. In each iteration of the loop, the value of i
is added to sum
.
while
Loop With else
In a while
loop, you can also have an else
part which will be executed when the condition
of while
is evaluated to be False
.
break
to terminate the while
loop, it will ignore the else
part.count = 0
while count < 4:
print("You are inside while loop")
count = count + 1
else:
print("You are in else part")
You are inside while loop
You are inside while loop
You are inside while loop
You are inside while loop
You are in else part
When count
becomes greater than 4, else
part is executed.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook