How to Fix NameError: Name 'xrange' Is Not Defined in Python
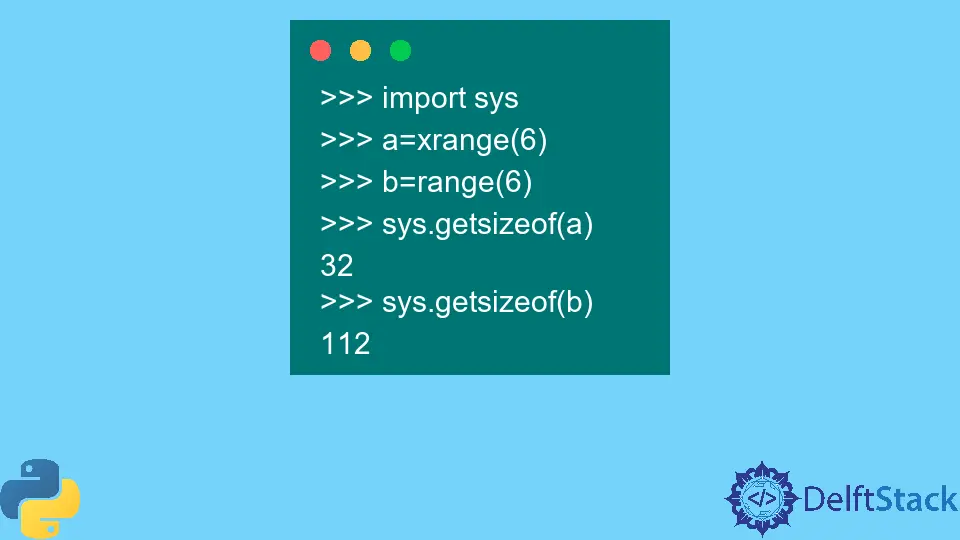
We will learn why we get an error when we call the xrange()
function in Python3 and see how we can fix this issue. We will also learn the difference between range()
and xrange()
and see how to use the range()
function in different Python versions.
NameError: name 'xrange' is not defined
When Using the xrange()
Function Python3
You may know that a big part of the transition from Python2 to Python3 is that the xrange()
function no longer exists in Python3. We will use Python2 and Python3 side-by-side and compare them and see the differences between range()
and xrange()
in both versions of Python.
First, we will use a Python2 environment where we can use the xrange()
function, but the Python2 environment also has the range()
function. We will see these functions behave slightly differently; xrange()
returns an xrange()
object, and range()
returns a list.
In Python3, the range()
function returns a range()
object, whereas in Python2, the range()
function returns a list. Basically, xrange()
is a generator, and range()
is also a generator in Python3.
Let’s now focus on Python2. We first want to examine this xrange()
object a bit.
We will see what type it has and if we have an xrange
object.
type(xrange(6))
Output
<type 'xrange'>
Next, we will see what happens if we wrap a list around it.
list(xrange(6))
Output
[0, 1, 2, 3, 4, 5]
Now, we will assign xrange(6)
to a variable named a
, create a range()
object and assign that to the b
variable. We will import sys
to get the size of both a
and b
.
When we run this code in the Python shell, we should notice a big difference between both variable sizes.
>>> import sys
>>> a=xrange(6)
>>> b=range(6)
>>> sys.getsizeof(a)
32
>>> sys.getsizeof(b)
112
The xrange()
object has a smaller size than range()
that is why xrange()
is faster than range()
.
Because of faster execution, beginners usually try to use the xrange()
function in Python3 and get an error (name 'xrange' is not defined
) because Python3 does not support the xrange()
function.
# in python 3
xrange(6)
Output:
NameError: name 'xrange' is not defined
In Python3, the xrange()
object became the range()
object.
# in python 3
>>> range(6)
range(0, 6)
Let’s import sys
to see the sizes of the list and range()
objects in Python3.
# in python 3
>>> a=range(6)
>>> b=[0,1,2,3,4,5]
>>> import sys
>>> sys.getsizeof(a)
48
>>> sys.getsizeof(b)
152
We got 48 which is much more comparable with the list. You can see that xrange()
from Python2 and range()
from Python3 would probably run at a similar speed and take a similar amount of space in memory.
In the Python3 environment, we cannot use the xrange()
function since, as we said, xrange()
no longer exists; it was never made into Python3. We can use the range()
function instead of xrange()
because range()
function is much faster in Python3.
Hello! I am Salman Bin Mehmood(Baum), a software developer and I help organizations, address complex problems. My expertise lies within back-end, data science and machine learning. I am a lifelong learner, currently working on metaverse, and enrolled in a course building an AI application with python. I love solving problems and developing bug-free software for people. I write content related to python and hot Technologies.
LinkedInRelated Article - Python Error
- Can Only Concatenate List (Not Int) to List in Python
- How to Fix Value Error Need More Than One Value to Unpack in Python
- How to Fix ValueError Arrays Must All Be the Same Length in Python
- Invalid Syntax in Python
- How to Fix the TypeError: Object of Type 'Int64' Is Not JSON Serializable
- How to Fix the TypeError: 'float' Object Cannot Be Interpreted as an Integer in Python