How to Find Mode of a List in Python
-
Use the
max()
Function and a Key to Find the Mode of a List in Python -
Use the
Counter
Class in the Collections Package to Find the Mode of a List in Python -
Use the
mode()
Function From thestatistics
Module to Find the Mode of a List in Python -
Use the
multimode()
Function From the Statistics Module to Find a List of Modes in Python - Conclusion
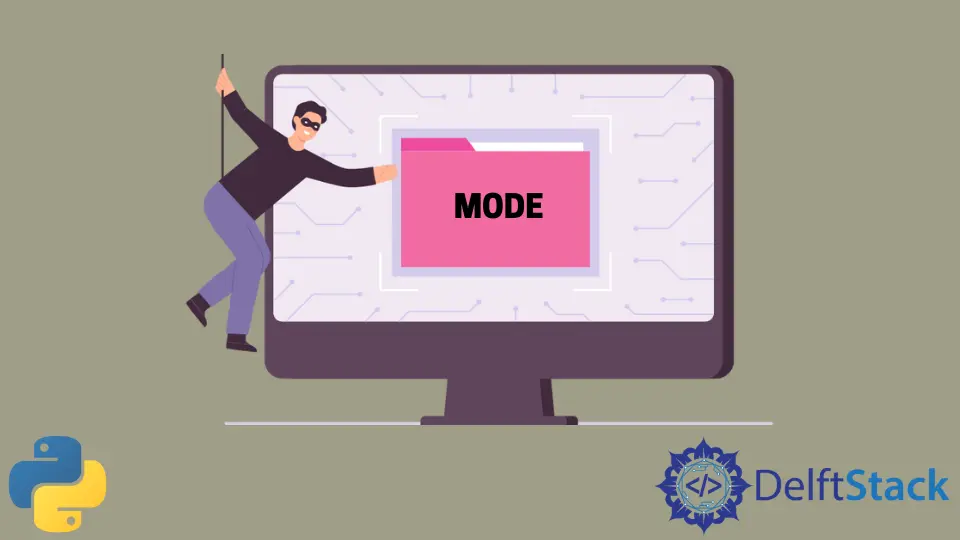
A list is one of the most powerful data structures used in Python to preserve the sequence of data and iterate over it. It can contain different data types like numbers, strings, and more.
In a given data set, a mode is a value or element that appears with the highest frequency.
There can be one mode, more than one mode, or no mode at all. There will be no mode if all the elements are unique.
In this tutorial, we will discuss how to find the mode of a list in Python.
Use the max()
Function and a Key to Find the Mode of a List in Python
The max()
function can return the maximum value of the given data set. The key
argument with the count()
method compares and returns the number of times each element is present in the data set.
Therefore, the function max(set(list_name), key = list_name.count)
will return the element that occurs the maximum times in the given list that is the required mode of the list.
Basic Syntax:
max(iterable, key=func, default=defaultValue)
Parameters:
iterable
: This parameter represents the collection of elements from which the maximum value is determined.key
(optional): This parameter is a function that can be used to customize the comparison and determine the maximum based on the result of applying this function to each element. If not provided, the maximum is determined based on the natural ordering of the elements.default
(optional): When the iterable is empty, this parameter specifies the value to be returned. Ifdefault
is not provided and the iterable is empty, aValueError
is raised.
Example Code 1:
A = [10, 30, 50, 10, 50, 80, 50]
print("Mode of List A is % s" % (max(set(A), key=A.count)))
B = ["Hi", 10, 50, "Hi", 100, 10, "Hi"]
print("Mode of List B is % s" % (max(set(B), key=B.count)))
Output:
Mode of List A is 50
Mode of List B is Hi
In the first example, we have two lists, A
and B
. We are determining their modes using the max(set(data), key=data.count)
approach.
First, for list A
, we find the mode, which is the element with the highest frequency in the list.
Then, we create a set from the list to remove duplicates, and then we use the max()
function with the key
argument set to A.count
to identify the element with the maximum count in the original list A
. For List B
, we apply the same approach to find its mode.
In this case, since the list contains both strings and numbers, we use the same technique to find the mode, which turns out to be the string Hi
with the highest frequency. The output of the code will display the modes of both lists A
and list B
, providing the most frequently occurring elements in each list.
Example Code 2:
C = [10, 30, "Hello", 30, 10, "Hello", 30, 10]
print("Mode of List C is % s" % (max(set(C), key=C.count)))
Output:
Mode of List C is 10
In the second example, we have a list named C
that contains a combination of numbers and strings. We want to find the mode of this list, which is the element with the highest frequency.
To do this, we first create a set from the list C
to eliminate duplicates, and then we apply the max(set(C), key=C.count)
approach. This approach determines the element with the maximum count in the original list C
.
In this specific case, the mode of list C
is Hello
because it appears three times, which is more frequent than any other element in the list. Therefore, the code will output Mode of List C is Hello
, indicating that Hello
is the mode of list C
.
Use the Counter
Class in the Collections Package to Find the Mode of a List in Python
The Counter
class in the collections package is used to count the number of occurrences of each element present in the given data set.
The .most_common()
method of the Counter
class returns a list containing two-item tuples with each unique element and its frequency.
Basic Syntax:
from collections import Counter
# Create a Counter object from an iterable
counter_object = Counter(iterable)
Parameter:
iterable
: This is the collection of elements for which you want to count occurrences.
Example Code:
from collections import Counter
A = [10, 10, 30, 10, 50, 30, 60]
Elements_with_frequency = Counter(A)
print(Elements_with_frequency.most_common())
Output:
[(10, 3), (30, 2), (50, 1), (60, 1)]
In this code, we are using the Counter
class from the collections
module to determine the frequency of each element in the list A
. We first import the Counter
class and then create an instance called Elements_with_frequency
by applying it to the list A
.
The most_common()
method is used to retrieve a list of elements in A
along with their corresponding frequencies, sorted in descending order of frequency. When we print the result of Elements_with_frequency.most_common()
, it will display the elements and their frequencies in list A
.
The output will show the elements and their frequencies in descending order of frequency, allowing us to see which elements occur most frequently in the list.
In the following code example, the Counter(list_name).most_common(1)[0][0]
function will return the required mode of the list. When there are multiple modes present in the list, it will return the smallest mode.
Example Code:
from collections import Counter
A = [10, 10, 30, 10, 50, 30, 60]
print("Mode of List A is % s" % (Counter(A).most_common(1)[0][0]))
Output:
Mode of List A is 10
In this code, we create an instance of it directly by applying it to the list A
. To determine the mode, we use the most_common(1)
method, which returns a list containing the element with the highest frequency and its count.
By accessing the first element of this list with [0][0]
, we obtain the mode of List A
, which is the element with the highest frequency. The code will output Mode of List A is 10
, indicating that the number 10
is the mode of list A
, as it appears most frequently in the list.
Use the mode()
Function From the statistics
Module to Find the Mode of a List in Python
The mode()
function in Python, which is part of the statistics
module, is used to find the mode (the most frequently occurring value) in a sequence of data.
Basic Syntax:
from statistics import mode
# Calculate the mode of a sequence of data
mode_value = mode(data)
Parameter:
data
: This parameter represents the sequence of data for which you want to find the mode.
Example Code:
from statistics import mode
A = [10, 20, 20, 30, 30, 30]
print("Mode of List A is % s" % (mode(A)))
B = ["Yes", "Yes", "Yes", "No", "No"]
print("Mode of List B is % s" % (mode(B)))
Output:
Mode of List A is 30
Mode of List B is Yes
In this code, we are using the mode
function from the statistics
module to find the mode of two lists, A
and B
. First, we import the mode
function.
Then, for list A
, which contains numerical values, we apply the mode()
function to determine the mode, which is the most frequently occurring value. For list B
, which contains string values, we use the same function to find its mode.
The output of the code will display the mode of both lists. In this case, for list A
, the mode is 30
because it appears most frequently (3 times), and for list B
, the mode is Yes
because it is the most frequent string (3 times) in the list.
This function will raise the StatisticsError
when the data set is empty or when more than one mode is present. However, in the newer versions of Python, the smallest element will be considered the mode when there are multiple modes of a sequence.
Use the multimode()
Function From the Statistics Module to Find a List of Modes in Python
The multimode()
function in the statistics module takes some data set as a parameter and returns a list of modes. We can use this function when more than one modal value is present in a given data set.
Basic Syntax:
from statistics import multimode
modes = multimode(data)
Parameter:
data
: This is a required parameter and represents the list of data for which you want to find the mode(s). It can be a list of numbers or strings.
Parameter:
Example :
from statistics import multimode
A = [10, 20, 20, 30, 30, 30, 20]
print("Mode of List A is % s" % (multimode(A)))
B = ["Yes", "Yes", "Yes", "No", "No", "No", "Maybe", "Maybe"]
print("Mode of List B is % s" % (multimode(B)))
Output:
Mode of List A is [20, 30]
Mode of List B is ['Yes', 'No']
In this code, we are using the multimode
function from the statistics
module to find the mode(s) of two lists, A
and B
. We begin by importing the multimode
function.
For list A
, which contains numerical values, we apply the multimode()
function to determine its mode(s). Similarly, for list B
, which consists of string values, we use the same function to find its mode(s).
The output of the code will display the mode(s) of both lists. For list A
, the mode is 30
, as it occurs most frequently (3 times), and for list B
, the modes are 'Yes'
and 'No'
because they are the most frequently occurring strings (3 times each) in the list.
Conclusion
In summary, a list is a fundamental data structure in Python, capable of storing diverse data types while preserving their order. The mode of a list represents the value with the highest frequency of occurrence.
When it comes to determining the mode of a list in Python, several methods are at your disposal. One approach involves using the max()
function along with the count()
method, which returns the element with the highest count.
Alternatively, the Counter
class from the collections
package counts the occurrences of each element and provides the mode using Counter(list_name).most_common(1)[0][0]
. The statistics
module offers a mode()
function to find the mode of a list, and it selects the smallest element in case of multiple modes in newer Python versions.
For situations with multiple modes, the multimode()
function from the statistics
module returns a list of all modes. These methods give you the flexibility to choose the most suitable approach for finding the mode of a list in Python, depending on your specific needs.
Related Article - Python List
- How to Convert a Dictionary to a List in Python
- How to Remove All the Occurrences of an Element From a List in Python
- How to Remove Duplicates From List in Python
- How to Get the Average of a List in Python
- What Is the Difference Between List Methods Append and Extend
- How to Convert a List to String in Python