在 Python 中查詢列表的模式
Samyak Jain
2023年1月30日
-
在 Python 中使用
max()
函式和一個鍵來查詢列表的模式 -
在 Python 中使用集合包中的
Counter
類查詢列表的模式 -
在 Python 中使用
statistics
模組中的mode()
函式查詢列表的模式 -
在 Python 中使用統計模組中的
multimode()
函式查詢模式列表
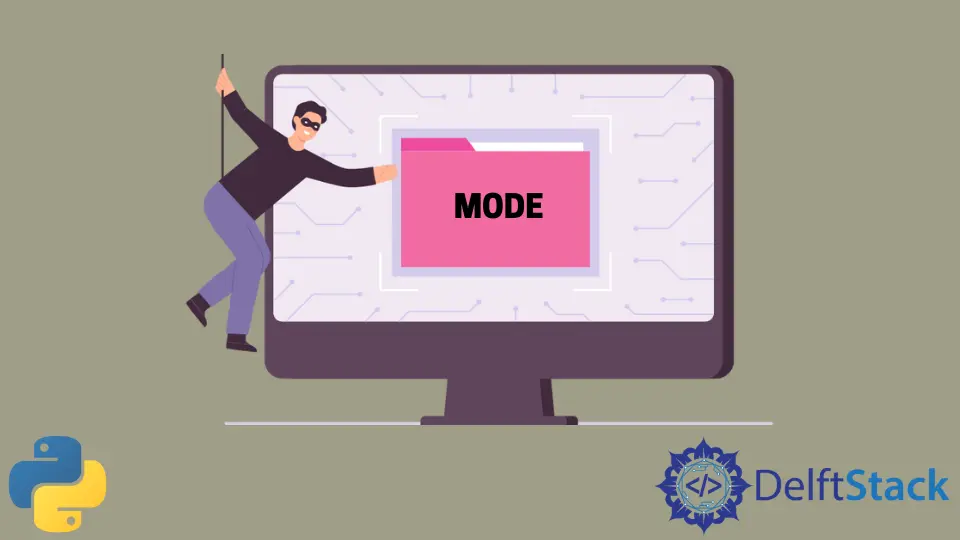
列表是 Python 中最強大的資料結構之一,用於儲存資料序列並對其進行迭代。它可以包含不同的資料型別,如數字、字串等。
在給定的資料集中,眾數是出現頻率最高的值或元素。可以有一種模式、多種模式或根本沒有模式。如果所有元素都是唯一的,就沒有模式。
在本教程中,我們將討論如何在 Python 中找到列表的模式。
在 Python 中使用 max()
函式和一個鍵來查詢列表的模式
max()
函式可以返回給定資料集的最大值。帶有 count()
方法的 key
引數比較並返回每個元素在資料集中出現的次數。
因此,函式 max(set(list_name), key = list_name.count)
將返回給定列表中出現次數最多的元素,即列表所需的模式。
例如,
A = [10, 30, 50, 10, 50, 80, 50]
print("Mode of List A is % s" % (max(set(A), key=A.count)))
B = ["Hi", 10, 50, "Hi", 100, 10, "Hi"]
print("Mode of List B is % s" % (max(set(B), key=B.count)))
輸出:
Mode of List A is 50
Mode of List B is Hi
當資料集中存在多種模式時,此函式將返回最小的模式。
例如,
C = [10, 30, "Hello", 30, 10, "Hello", 30, 10]
print("Mode of List C is % s" % (max(set(C), key=C.count)))
輸出:
Mode of List C is 10
在 Python 中使用集合包中的 Counter
類查詢列表的模式
collections 包中的 Counter
類用於計算給定資料集中每個元素出現的次數。
Counter
類的 .most_common()
方法返回一個列表,該列表包含具有每個唯一元素及其頻率的兩項元組。
例如,
from collections import Counter
A = [10, 10, 30, 10, 50, 30, 60]
Elements_with_frequency = Counter(A)
print(Elements_with_frequency.most_common())
輸出:
[(10, 3), (30, 2), (50, 1), (60, 1)]
Counter(list_name).most_common(1)[0][0]
函式將返回列表所需的模式。當列表中存在多個模式時,它將返回最小的模式。
例子 :
from collections import Counter
A = [10, 10, 30, 10, 50, 30, 60]
print("Mode of List A is % s" % (Counter(A).most_common(1)[0][0]))
輸出:
Mode of List A is 10
在 Python 中使用 statistics
模組中的 mode()
函式查詢列表的模式
python 統計模組中的 mode()
函式將一些資料集作為引數並返回其模式值。
例子 :
from statistics import mode
A = [10, 20, 20, 30, 30, 30]
print("Mode of List A is % s" % (mode(A)))
B = ["Yes", "Yes", "Yes", "No", "No"]
print("Mode of List B is % s" % (mode(B)))
輸出:
Mode of List A is 30
Mode of List B is Yes
當資料集為空或存在多個模式時,此函式將引發 StatisticsError
。但是,在較新版本的 Python 中,當一個序列有多個模式時,最小元素將被視為模式。
在 Python 中使用統計模組中的 multimode()
函式查詢模式列表
統計模組中的 multimode()
函式將一些資料集作為引數並返回一個模式列表。當給定資料集中存在多個模態值時,我們可以使用此函式。
例子 :
from statistics import multimode
A = [10, 20, 20, 30, 30, 30, 20]
print("Mode of List A is % s" % (multimode(A)))
B = ["Yes", "Yes", "Yes", "No", "No", "No", "Maybe", "Maybe"]
print("Mode of List B is % s" % (multimode(B)))
輸出:
Mode of List A is [20, 30]
Mode of List B is ['Yes', 'No']