The maketrans Function in Python
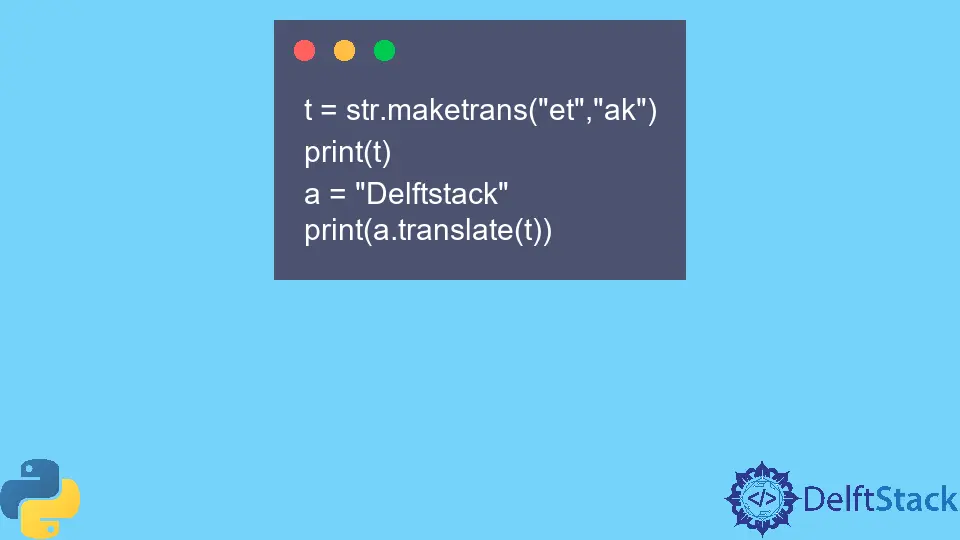
The maketrans()
function is used to create a translation table that maps out some integers or characters to some specified value.
This value can be an integer, character, or None
. This table is used with the translate()
function to map out and replace the elements with the given values in a string.
We can create the translation table using three ways with the maketrans()
. We will discuss these methods in this article.
Using the maketrans()
Function in Python
We will provide the maketrans()
function with only one argument in the first method.
This argument is a dictionary, where we map out the elements using key-value pairs. The key will contain the elements to be replaced, and their replacement elements will be the value of the keys.
We can display the translation table and use it with the translate()
function. See the following example.
t = str.maketrans({"e": "a", "t": "k"})
print(t)
a = "Delftstack"
print(a.translate(t))
Output:
{101: 'a', 116: 'k'}
Dalfkskack
In the above example, we displayed the translation table.
The elements to be replaced are mapped out using their ASCII value. We use the defined table with the a
string using the translate()
function.
Another way to use the maketrans()
function is by providing it with two arguments. The two arguments provided are strings and should be of equal length.
The element at a particular index in the first string is replaced by the character at the corresponding index in the second string.
For example,
t = str.maketrans("et", "ak")
print(t)
a = "Delftstack"
print(a.translate(t))
Output:
{101: 97, 116: 107}
Dalfkskack
In this method, all elements in the translation table are converted to their corresponding ASCII values.
The final method involves the use of three arguments. The first two arguments are the same as discussed in the previous method.
The additional third argument is also a string. The characters from this argument are mapped out to None
.
This indicates that they will be removed whenever encountered. See the following example.
t = str.maketrans("et", "ak", "lf")
print(t)
a = "Delftstack"
print(a.translate(t))
Output:
{101: 97, 116: 107, 108: None, 102: None}
Dakskack
Note how the third argument string characters, l
and f
, are mapped out to None
and are omitted from the final string.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedInRelated Article - Python String
- How to Remove Commas From String in Python
- How to Check a String Is Empty in a Pythonic Way
- How to Convert a String to Variable Name in Python
- How to Remove Whitespace From a String in Python
- How to Extract Numbers From a String in Python
- How to Convert String to Datetime in Python