How to Calculate Log Base 2 of a Number in Python
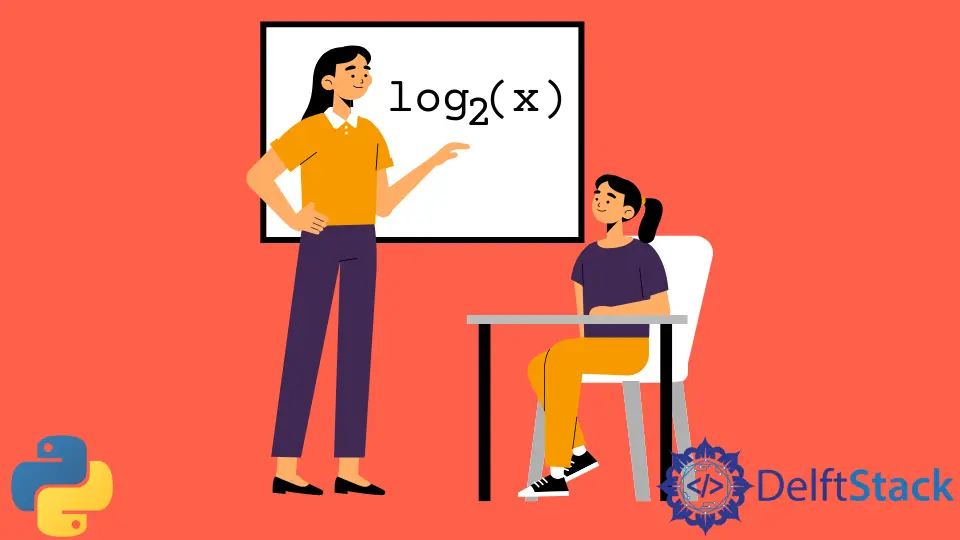
Python is well known for its ease of use, a diverse range of libraries, and easy-to-understand syntax. Many common problems can be easily solved using the power of Python. And, calculating logarithmic values is also an effortless task in Python.
Python has a built-in library, math
, which has all sorts of mathematical functions to carry out mathematical computations. And, this library provides accessible functions to calculate logarithmic results as well.
Log Base 2 of a Number Using math
Library in Python
There are two functions from the math
library that we can use to calculate log with base 2. The first method uses the log()
function, and the second method uses the log2()
function.
The log()
function accepts two arguments. The first argument is the number, and the second argument is the base value. Since we wish to calculate the log with the base as 2, we’ll pass the base value as 2
. By default, the math.log()
function considers the base value to be e
or the natural logarithm.
Refer to the following code.
import math
number = 25
answer = math.log(number, 2)
print(answer)
Output:
4.643856189774724
The log2()
function directly calculates the log base 2 of a number. We have to pass the number we wish to calculate the log of, and this function will take care of the rest.
Refer to the following code.
import math
number = 25
answer = math.log2(number)
print(answer)
Output:
4.643856189774724
Apart from the two ways we talked about above, we can also use the property of log to compute log base 2. By default, as mentioned above, the math.log()
function considers the base to be e
or natural log. Hence, we can easily calculate the value for our desired base value, 2, using the property below.
See the below example.
import math
number = 25
numerator = math.log(number)
denominator = math.log(2)
answer = numerator / denominator
print(answer)
Output:
4.643856189774724
To learn more about the
math
library in-depth, refer to the official documents here