How to Convert List to Comma-Separated String in Python
-
Use the
join()
Method to Convert a List to a Comma-Separated String in Python -
Use List Comprehension and the
join()
Method to Convert a List to a Comma-Separated String in Python -
Use
map()
with thejoin()
Method to Convert a List to a Comma-Separated String in Python -
Use the
StringIO
Module to Convert a List to a Comma-Separated String in Python -
Use the
print()
Function With theUnpack
Operator to Convert a List to a Comma-Separated String in Python -
Use the
reduce()
Function to Convert a List to a Comma-Separated String in Python - Use a Loop to Convert a List to a Comma-Separated String in Python
- Conclusion
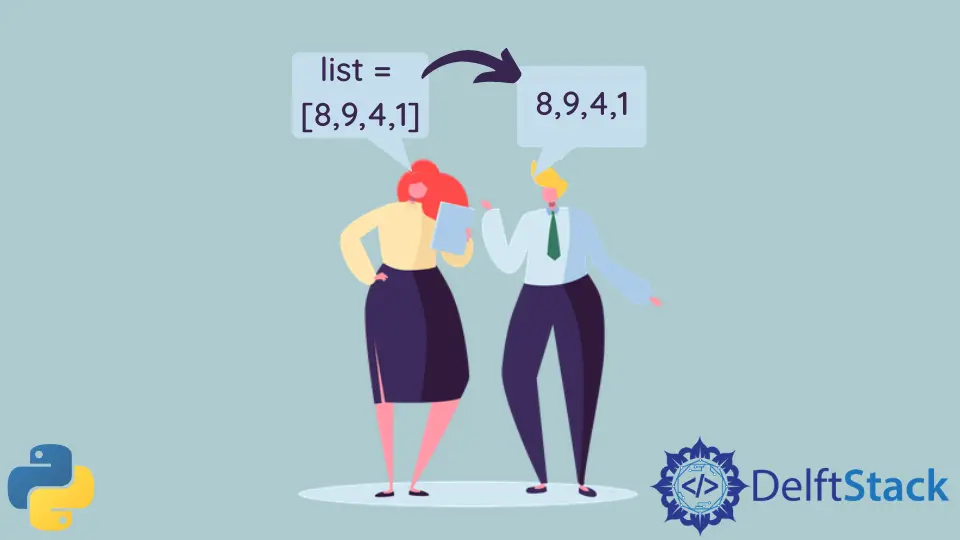
Converting a list into a comma-separated string is a fundamental operation in Python, essential for various data manipulation tasks.
In this tutorial, we’ll explore multiple methods to achieve this objective.
Use the join()
Method to Convert a List to a Comma-Separated String in Python
One of the most common ways to convert a list into a comma-separated string is by using the join()
method. This method is a string method that concatenates the elements of an iterable (such as a list) into a single string.
The syntax of the join()
method is as follows:
separator.join(iterable)
Here, separator
is the string that will be used to concatenate the elements, and iterable
is the iterable object (e.g., a list) whose elements we want to join.
Let’s dive into a practical example using the join()
method to convert a list to a comma-separated string:
my_list = ["apple", "banana", "orange"]
result_string = ", ".join(my_list)
print(result_string)
In the provided code example, we start by defining a list named my_list
containing strings representing fruits. We then use the join()
method to concatenate the elements of the list into a single string, separating each element with a comma and a space (,
).
The resulting string is stored in the variable result_string
. Then, the print(result_string)
statement outputs the final comma-separated string to the console.
apple, banana, orange
In this output, you can see that the elements of the list have been successfully joined into a comma-separated string, creating a clean and readable output.
Using the join()
method is not only concise but also efficient, making it a preferred choice for converting lists to strings in Python.
Use List Comprehension and the join()
Method to Convert a List to a Comma-Separated String in Python
An alternative and concise approach to transforming a list into a comma-separated string involves using list comprehension along with the join()
method. This combination allows us to iterate through the elements of the list and join them into a single string with a specified separator.
The syntax of list comprehension with the join()
method can be expressed as follows:
separator.join(str(element) for element in iterable)
Here, separator
denotes the string used as a separator, and iterable
represents the iterable object (e.g., a list) from which we want to construct the string.
Let’s see an example using list comprehension and the join()
method to convert a list to a comma-separated string:
number_list = [1, 2, 3, 4, 5]
result_string = ",".join(str(number) for number in number_list)
print(result_string)
In the provided code example, we initialize a list named number_list
containing numeric values. Using list comprehension and the join()
method, we iterate through each element in the list, converting it to a string using str(number)
.
The join()
method then concatenates these string elements with commas as separators, resulting in a single string stored in the variable result_string
.
Output:
1,2,3,4,5
In this output, you can observe that the elements of the list have been successfully transformed into a comma-separated string. The combination of list comprehension and the join()
method provides a concise and efficient solution for this common task in Python.
Use map()
with the join()
Method to Convert a List to a Comma-Separated String in Python
Another method we can use for converting a list into a comma-separated string is the map()
function in conjunction with the join()
method. This approach allows us to apply a specified function (in this case, the str()
function) to each element of the list before joining them into a single string.
The syntax for using map()
with the join()
method can be summarized as follows:
separator.join(map(str, iterable))
Here, separator
represents the string used as a separator, and iterable
is the iterable object (e.g., a list) whose elements we want to convert and join.
Let’s delve into a practical example using the map()
function and the join()
method:
temperature_list = [25.5, 30.2, 22.8, 18.5]
result_string = ",".join(map(str, temperature_list))
print(result_string)
In the provided code example, we begin with a list named temperature_list
containing floating-point values. Using the map()
function, we apply the str()
function to each element in the list, converting them into strings.
Subsequently, the join()
method concatenates these string elements with commas as separators, creating a single string stored in the variable result_string
.
Output:
25.5,30.2,22.8,18.5
Here, you can see that the elements of the list, representing temperatures, have been successfully converted and joined into a comma-separated string.
Use the StringIO
Module to Convert a List to a Comma-Separated String in Python
An alternative method for converting a list into a comma-separated string involves utilizing the StringIO
module. The StringIO
module is part of the io
module in Python and functions similarly to a file object.
This allows us to work with text data in memory, providing a way to construct strings similar to working with files.
In this example, we’ll employ it to convert a list into a comma-separated string.
import io
import csv
temperature_list = [22.5, 30.0, 18.2, 25.5]
string_io = io.StringIO()
csv_writer = csv.writer(string_io)
csv_writer.writerow(temperature_list)
result_string = string_io.getvalue()
print(result_string)
To begin, we import the io
module and the csv
module. We define a list named temperature_list
containing floating-point values.
We then create a StringIO
object named string_io
, followed by a csv.writer
object named csv_writer
. Using the writerow()
method, we write the contents of the temperature_list
as a comma-separated row.
The getvalue()
method retrieves the value stored in the StringIO
object, and the resulting string is stored in the variable result_string
.
Output:
22.5,30.0,18.2,25.5
Here, you can observe that the elements of the list have been successfully transformed into a comma-separated string using the StringIO
module. This method is particularly useful when working with data that needs to be formatted as a CSV row or when a more file-like approach is desired for string construction.
Use the print()
Function With the Unpack
Operator to Convert a List to a Comma-Separated String in Python
In addition to the previously discussed methods, we can employ the print()
function along with the unpack
operator (*
) as an alternative way to convert a list into a comma-separated string. The unpack
operator can unpack all the elements of an iterable, and when combined with the print()
function, it allows for concise string construction.
The syntax for using the unpack
operator with the print()
function is as follows:
print(*iterable, sep=separator, end="")
Here, iterable
represents the iterable object (e.g., a list) whose elements we want to print, separator
is the string used as a separator, and end
specifies the string that is printed at the end.
Let’s dive into a practical example using the unpack
operator with the print()
function to convert a list to a comma-separated string:
import io
city_list = ["New York", "London", "Tokyo", "Paris"]
string_io = io.StringIO()
print(*city_list, sep=",", end="", file=string_io)
result_string = string_io.getvalue()
print(result_string)
In this code example, we start by defining a list named city_list
containing string values representing cities. We then create a StringIO
object named string_io
.
Using the print()
function with the unpack
operator (*city_list
), we print the elements of the list with a comma separator. The sep
parameter is set to ,
, and the end
parameter is set to an empty string to ensure there is no newline character at the end.
The getvalue()
method retrieves the value stored in the StringIO
object, and the resulting string is stored in the variable result_string
.
The second print(result_string)
statement outputs the final comma-separated string to the console.
Output:
New York,London,Tokyo,Paris
Here, the elements of the list have been successfully converted into a comma-separated string using the unpack
operator with the print()
function. This method is particularly useful when a concise one-liner is preferred for list-to-string conversion.
Use the reduce()
Function to Convert a List to a Comma-Separated String in Python
Yet another method for converting a list into a comma-separated string involves using the reduce()
function from the functools
module. The reduce()
function is particularly powerful for iteratively applying a binary function to the items of an iterable, progressively reducing it to a single accumulated result.
The syntax for the reduce()
function is as follows:
functools.reduce(function, iterable, initial=None)
Here, function
is the binary function to be applied cumulatively to the items of the iterable, and initial
is an optional initial value for the accumulation. In our case, the iterable is a list of elements that we want to concatenate into a string.
Let’s delve into an example using the reduce()
function along with the join()
method to convert a list to a comma-separated string:
from functools import reduce
country_list = ["USA", "Canada", "UK", "Australia"]
result_string = reduce(lambda x, y: x + ", " + y, country_list)
print(result_string)
In this code example, we start by importing the reduce()
function from the functools
module. We define a list named country_list
containing string values representing countries.
We then use the reduce()
function along with a lambda function as the binary function. The lambda function takes two arguments (x
and y
) and concatenates them with a comma and a space in between.
The reduce()
function iteratively applies the lambda function to the items of the country_list
, progressively accumulating the result. The final comma-separated string is stored in the variable result_string
.
Output:
USA, Canada, UK, Australia
You can observe that the elements of the list have been successfully converted into a comma-separated string using the reduce()
function. This method is especially useful when a more complex binary function is needed for accumulation.
Use a Loop to Convert a List to a Comma-Separated String in Python
In certain situations, using a loop can be a straightforward and effective approach to convert a list into a comma-separated string. This method allows us to iterate through the elements of the list and concatenate them with a specified separator.
Let’s delve into a practical example:
fruit_list = ["apple", "banana", "orange", "kiwi"]
result_string = ""
for fruit in fruit_list:
result_string += fruit + ", "
result_string = result_string[:-2]
print(result_string)
We start by defining a list named fruit_list
containing string values representing fruits. We then initialize an empty string result_string
to store the final result.
A loop is then employed to iterate through each element in fruit_list
. During each iteration, the current fruit is concatenated to result_string
with a comma and a space separator.
After the loop completes, we remove the trailing comma and space from result_string
to ensure a clean output.
Output:
apple, banana, orange, kiwi
While this method may involve more explicit steps compared to other approaches, it offers a clear and intuitive way to concatenate elements in a controlled manner.
Conclusion
In this article, we’ve explored various methods to convert a list to a comma-separated string in Python. Each approach has its merits, catering to different scenarios and preferences.
Whether you prioritize simplicity, versatility, or intricate operations, understanding these techniques equips you with the knowledge to adapt your code effectively. Choose the method that aligns with your specific needs and enhances your proficiency in Pythonic data manipulation.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedInRelated Article - Python List
- How to Convert a Dictionary to a List in Python
- How to Remove All the Occurrences of an Element From a List in Python
- How to Remove Duplicates From List in Python
- How to Get the Average of a List in Python
- What Is the Difference Between List Methods Append and Extend
- How to Convert a List to String in Python