How to Fix the Key Error in a Dictionary in Python
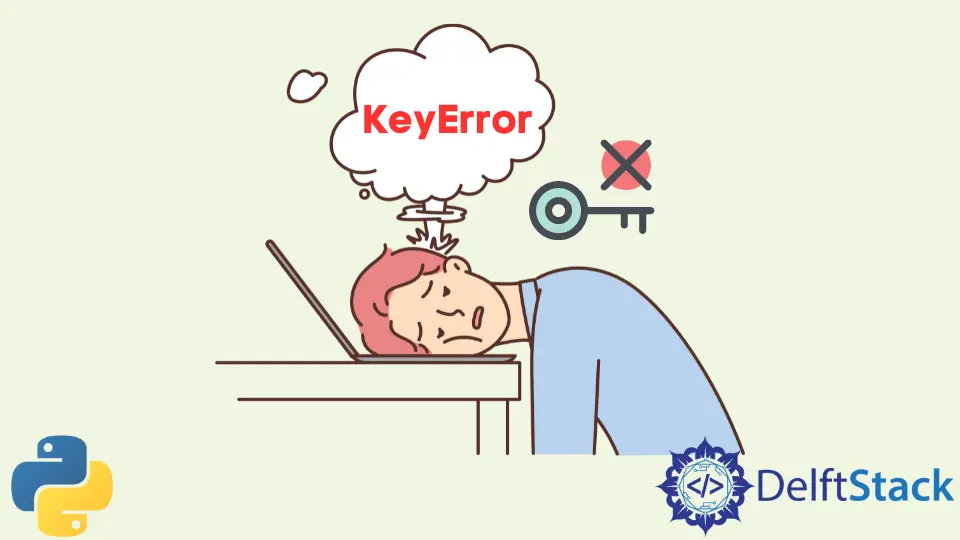
Dictionary is a scalable data structure available in Python. It stores data in the form of key-value pairs where a key can be any hashable and immutable object and value can be anything; a list, a tuple, a dictionary, a list of objects, and so on.
Using keys, we can access the values these keys are pointing to. If a non-existent key is given to a dictionary, it throws a KeyError
exception. In this article, we will learn how to handle this exception in Python.
Fix the KeyError
Exception in a Dictionary in Python
To resolve the KeyError
exception, one can check if the key exists in the dictionary before accessing it. The keys()
method returns a list of keys inside the dictionary. Before accessing the value at a key, it is recommended to check if the key exists in this list if you are unsure about its existence. The following Python code depicts the same.
data = {
"a": 101,
"b": 201,
"c": 301,
"d": 401,
"e": 501,
}
keys = ["a", "e", "r", "f", "c"]
for key in keys:
if key in data.keys():
print(data[key])
else:
print(f"'{key}' not found.")
Output:
101
501
'r' not found.
'f' not found.
301
Apart from the approach discussed above, one can also use a try...except
block to catch the KeyError
exception or any exception. Refer to the following Python code for the same.
data = {
"a": 101,
"b": 201,
"c": 301,
"d": 401,
"e": 501,
}
keys = ["a", "e", "r", "f", "c"]
for key in keys:
try:
print(data[key])
except:
print(f"'{key}' not found.")
Output:
101
501
'r' not found.
'f' not found.
301
The code under the except
block will get executed if a KeyError
exception occurs.
Related Article - Python Error
- Can Only Concatenate List (Not Int) to List in Python
- How to Fix Value Error Need More Than One Value to Unpack in Python
- How to Fix ValueError Arrays Must All Be the Same Length in Python
- Invalid Syntax in Python
- How to Fix the TypeError: Object of Type 'Int64' Is Not JSON Serializable
- How to Fix the TypeError: 'float' Object Cannot Be Interpreted as an Integer in Python