How to Join List of Lists in Python
- Why We Need to Join a List of Lists Into a List
-
Join a List of Lists Into a List Using the
for
Loop in Python - Join a List of Lists Into a List Using List Comprehension
- Join a List of Lists Into One List Using Recursion in Python
- Join a List of Lists Into a List Using In-Built Python Functions
- Join a List of Lists Into a List Using Functools
-
Join a List of Lists Into a List Using the
Chain()
Method - Join a List of Lists Into a List Using Numpy Module
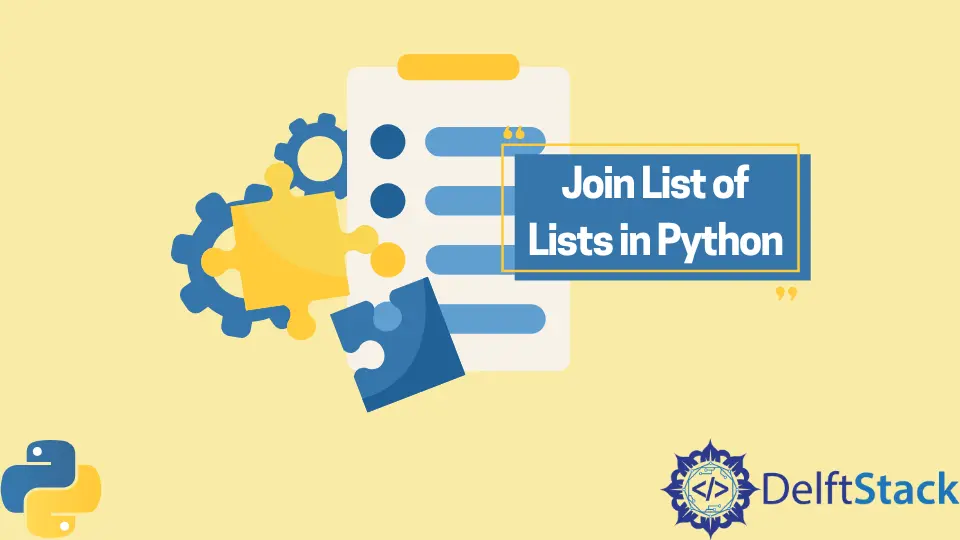
This article provides the various ways you can join a list of lists into a list in Python. Before we look at how you can join a list of lists into a list in Python, let us understand the requirement and what this joining process is called.
Why We Need to Join a List of Lists Into a List
A list of lists is a 2-D format, also known as a nested list, containing several sub-lists. A list is one of the most flexible data structures in Python, so joining a list of lists into a single 1-D list can be beneficial.
Here, we will convert this list of lists into a singular structure where the new list will comprise the elements contained in each sub-list from the list of lists. In the Python language, this process is also known as flattening.
A 1-D list containing all elements is a better approach as:
- It makes the code clear and readable for better documentation as it is easier to read from a single structure than a 2-D one.
- A 1-D list is helpful in data science applications as you can read, filter, or remove items from a list quickly.
- A list is also preferred when you wish to filter the data based on set theory.
Join a List of Lists Into a List Using the for
Loop in Python
The easiest method for converting a list of lists into a 1-D list is implementing a nested for loop with an if condition for distinct elements present. You can do this by:
Listoflists = [[100, 90, 80], [70, 60], [50, 40, 30, 20, 10]] # List of Lists
mylist = [] # new list
for a in range(len(Listoflists)): # List of lists level
for b in range(len(Listoflists[a])): # Sublist Level
mylist.append(Listoflists[a][b]) # Add element to mylist
print("List of Lists:", Listoflists)
print("1-D List:", mylist)
Output:
List of Lists: [[100, 90, 80], [70, 60], [50, 40, 30, 20, 10]]
1-D List: [100, 90, 80, 70, 60, 50, 40, 30, 20, 10]
In the above code snippet, we use nested for loops. The first loop picks each element in the main list and checks if it is a list type.
If the sub-element is a list, it initiates another for
loop to iterate this sub-list and add its values to the new list. Otherwise, it appends the single element found at that location.
Using the nested for
loop works with a regular and irregular list of lists but is a brute force approach.
Join a List of Lists Into a List Using List Comprehension
Another method of converting a nested list into a single list is using list comprehension. List comprehension provides an easily understandable single line of clean code to create entirely new lists using other structures such as strings, arrays, nested lists, etc.
Let us see how you can use list comprehension to solve our problem:
listoflists = [["Sahildeep", "Dhruvdeep"], [14.12, 8.6, 14.01], [100, 100]]
# mylist defined using list comprehension
mylist = [item for sublist in listoflists for item in sublist]
print("List of Lists", listoflists)
print("1-D list", mylist)
Output:
List of Lists [['Sahildeep', 'Dhruvdeep'], [14.12, 8.6, 14.01], [100, 100]]
1-D list ['Sahildeep', 'Dhruvdeep', 14.12, 8.6, 14.01, 100, 100]
In the above code snippet, we specify the creation of a new list with elements provided by each item of each sub-list found in our original list of lists. You can also combine sub-lists containing various data types elements into your 1-D list.
Join a List of Lists Into One List Using Recursion in Python
Recursion provides another way for flattening a list of lists in python. Let us see how you can implement recursion to create a single list from a list of lists:
def to1list(listoflists):
if len(listoflists) == 0:
return listoflists
if isinstance(listoflists[0], list):
return to1list(listoflists[0]) + to1list(listoflists[1:])
return listoflists[:1] + to1list(listoflists[1:])
print("MyList:", to1list([["Sahildeep", "Dhruvdeep"], [14.12, 8.6, 14.01], [100, 100]]))
Output:
MyList: ['Sahildeep', 'Dhruvdeep', 14.12, 8.6, 14.01, 100, 100]
In the above code snippet, we have defined a recursive function named to1list
that takes a list of lists, listoflists
is its parameter. If the list of lists contains a single element, it is returned as such.
Otherwise, an if statement with an isinstance()
is used to check if the list at the 0th location of our list of lists is a list.
If it is true, then we return the values of the sub-list at [0] and call our function, to1list
once again, but this time, skipping the 0th location and starting from one by specifying the slice and passing the sub-list as an argument.
Let us now see how to join nested lists into a list using in-built functions.
Join a List of Lists Into a List Using In-Built Python Functions
We can use the sum() function and lambda functions to join a list of lists in Python.
Join a List of Lists Using the sum()
Function
You can use the in-built sum function to create a 1-D list from a list of lists.
listoflists = [
["Sahildeep", "Dhruvdeep"],
[14.12, 8.6, 14.01],
[100, 100],
] # List to be flattened
mylist = sum(listoflists, [])
print("List of Lists:", listoflists)
print("My List:", mylist)
Output:
List of Lists: [['Sahildeep', 'Dhruvdeep'], [14.12, 8.6, 14.01], [100, 100]]
My List: ['Sahildeep', 'Dhruvdeep', '14.12', '8.6', '14.01', '100', '100']
In the above program, the sum function is passed with two parameters; the first is the list of lists that it will iterate, and the second is the start of our 1-D list that is initially empty.
Join a List of Lists Using Lambda Function
You can also use lambda, i.e., an anonymous function, to convert a list of lists into a list. Let us see with a code example:
listoflists = [
["Sahildeep", "Dhruvdeep"],
[14.12, 8.6, 14.01],
[100, 100],
] # List to be flattened
to1list = (
lambda listoflists: [element for item in listoflists for element in to1list(item)]
if type(listoflists) is list
else [listoflists]
)
print("List of lists:", listoflists)
print("MyList:", to1list(listoflists))
Output:
List of lists: [['Sahildeep', 'Dhruvdeep'], [14.12, 8.6, 14.01], [100, 100]]
MyList: ['Sahildeep', 'Dhruvdeep', 14.12, 8.6, 14.01, 100, 100]
In the above program, we define a lambda function that iterates each element of an item, i.e., a sub-list in the list of lists, and adds that element to the single list using to1list(item)
.
Let us see how you can convert a list of lists into a 1-D list using Python libraries.
Join a List of Lists Into a List Using Functools
One of the fastest ways that you can use to convert a 2-D list into a 1-D list in Python is by using its Functools module, which provides functions to work efficiently with higher or complex functions.
We can use the reduce()
function provided in the Functools module to join a list of lists into a list, as shown in the code below:
import operator
import functools
listoflists = [["Sahildeep", "Dhruvdeep"], [14.12, 8.6, 14.01], [100, 100]]
# mylist using functools
mylist = functools.reduce(operator.iconcat, listoflists, [])
print("List of Lists", listoflists)
print("1-D list", mylist)
Output:
List of Lists [['Sahildeep', 'Dhruvdeep'], [14.12, 8.6, 14.01], [100, 100]]
1-D list ['Sahildeep', 'Dhruvdeep', 14.12, 8.6, 14.01, 100, 100]
In the above code snippet, the new 1-D list is defined with the reduce()
function that uses the operator.iconcat
function to concatenate all elements from the sub-lists of our list of lists moving from left to right.
It would be best to remember adding imports for functools
and operator
as this approach requires both.
Join a List of Lists Into a List Using the Chain()
Method
Another Python library, known as Itertools
, contains a chain()
method that you can use to convert a list of lists into a 1-D list. Let us see how:
import itertools
listoflists = [
["Sahildeep", "Dhruvdeep"],
[14.12, 8.6, 14.01],
[100, 100],
] # List to be flattened
mylist = list(itertools.chain(*listoflists))
print("List of Lists:", listoflists)
print("My List:", mylist)
Output:
List of Lists: [['Sahildeep', 'Dhruvdeep'], [14.12, 8.6, 14.01], [100, 100]]
My List: ['Sahildeep', 'Dhruvdeep', 14.12, 8.6, 14.01, 100, 100]
In the above program, the chain()
method is used to iterate all the sub-lists in the list of lists and return a single iterable list containing all elements of all sub-lists.
Join a List of Lists Into a List Using Numpy Module
The Numpy library provides the functions to concatenate the substring and flatten them into a single 1-D list. Let us take a look at how you can convert a nested list into a list using the numpy.concatenate()
method.
import numpy
listoflists = [
["Sahildeep", "Dhruvdeep"],
[14.12, 8.6, 14.01],
[100, 100],
] # List to be flattened
mylist = list(numpy.concatenate(listoflists).flat)
print("List of Lists:", listoflists)
print("My List:", mylist)
Output:
List of Lists: [['Sahildeep', 'Dhruvdeep'], [14.12, 8.6, 14.01], [100, 100]]
My List: ['Sahildeep', 'Dhruvdeep', '14.12', '8.6', '14.01', '100', '100']
In the above code snippet, we have used our list of lists, the same as the above programs, and created a new list named mylist
using NumPy
’s concatenate()
and flat()
functions.
Related Article - Python List
- How to Convert a Dictionary to a List in Python
- How to Remove All the Occurrences of an Element From a List in Python
- How to Remove Duplicates From List in Python
- How to Get the Average of a List in Python
- What Is the Difference Between List Methods Append and Extend
- How to Convert a List to String in Python