在 Python 中将列表的列表连接为列表
- 为什么我们需要将列表的列表连接为列表
-
使用
for
循环将列表加入列表 - 使用列表推导将列表加入列表
- Python 中使用递归将列表的列表连接为列表
- 使用内置 Python 函数将列表的列表连接为列表
- 使用 Functools 将列表的列表连接为列表
-
使用
Chain()
方法将列表的列表连接为列表 - 使用 Numpy 模块将列表的列表连接为列表
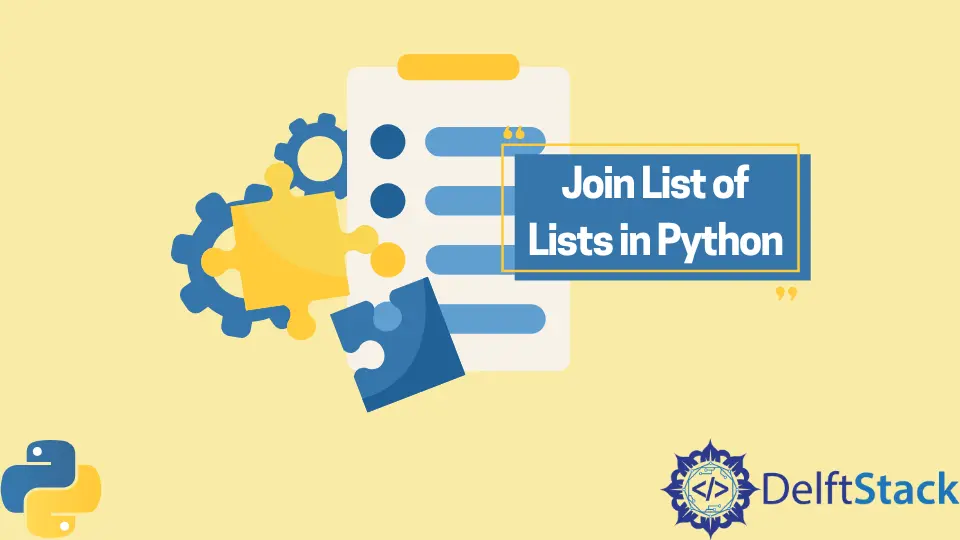
本文提供了在 Python 中将列表加入列表的各种方法。在我们了解如何将列表列表加入 Python 中的列表之前,让我们了解要求以及此加入过程称为什么。
为什么我们需要将列表的列表连接为列表
列表列表是一种二维格式,也称为嵌套列表,包含多个子列表。列表是 Python 中最灵活的数据结构之一,因此将列表列表加入单个一维列表可能是有益的。
在这里,我们将这个列表列表转换为一个单一结构,其中新列表将包含列表列表中每个子列表中包含的元素。在 Python 语言中,这个过程也称为展平。
包含所有元素的一维列表是一种更好的方法,因为:
- 它使代码清晰易读,以获得更好的文档,因为从单一结构比二维结构更容易阅读。
- 一维列表在数据科学应用程序中很有帮助,因为你可以快速读取、过滤或删除列表中的项目。
- 当你希望基于集合论过滤数据时,列表也是首选。
使用 for
循环将列表加入列表
将列表列表转换为一维列表的最简单方法是实现嵌套 for 循环,其中包含不同元素的 if 条件。你可以通过以下方式做到这一点:
Listoflists = [[100, 90, 80], [70, 60], [50, 40, 30, 20, 10]] # List of Lists
mylist = [] # new list
for a in range(len(Listoflists)): # List of lists level
for b in range(len(Listoflists[a])): # Sublist Level
mylist.append(Listoflists[a][b]) # Add element to mylist
print("List of Lists:", Listoflists)
print("1-D List:", mylist)
输出:
List of Lists: [[100, 90, 80], [70, 60], [50, 40, 30, 20, 10]]
1-D List: [100, 90, 80, 70, 60, 50, 40, 30, 20, 10]
在上面的代码片段中,我们使用了嵌套的 for 循环。第一个循环选择主列表中的每个元素并检查它是否是列表类型。
如果子元素是一个列表,它会启动另一个 for
循环来迭代这个子列表并将其值添加到新列表中。否则,它会附加在该位置找到的单个元素。
使用嵌套的 for
循环适用于规则和不规则的列表列表,但这是一种蛮力方法。
使用列表推导将列表加入列表
将嵌套列表转换为单个列表的另一种方法是使用列表推导。列表推导式提供了易于理解的单行干净代码,可以使用其他结构(如字符串、数组、嵌套列表等)创建全新的列表。
让我们看看如何使用列表推导来解决我们的问题:
listoflists = [["Sahildeep", "Dhruvdeep"], [14.12, 8.6, 14.01], [100, 100]]
# mylist defined using list comprehension
mylist = [item for sublist in listoflists for item in sublist]
print("List of Lists", listoflists)
print("1-D list", mylist)
输出:
List of Lists [['Sahildeep', 'Dhruvdeep'], [14.12, 8.6, 14.01], [100, 100]]
1-D list ['Sahildeep', 'Dhruvdeep', 14.12, 8.6, 14.01, 100, 100]
在上面的代码片段中,我们指定创建一个新列表,其中包含原始列表列表中每个子列表的每个项目提供的元素。你还可以将包含各种数据类型元素的子列表组合到一维列表中。
Python 中使用递归将列表的列表连接为列表
递归提供了另一种在 python 中展平列表列表的方法。让我们看看如何实现递归以从列表列表创建单个列表:
def to1list(listoflists):
if len(listoflists) == 0:
return listoflists
if isinstance(listoflists[0], list):
return to1list(listoflists[0]) + to1list(listoflists[1:])
return listoflists[:1] + to1list(listoflists[1:])
print("MyList:", to1list([["Sahildeep", "Dhruvdeep"], [14.12, 8.6, 14.01], [100, 100]]))
输出:
MyList: ['Sahildeep', 'Dhruvdeep', 14.12, 8.6, 14.01, 100, 100]
在上面的代码片段中,我们定义了一个名为 to1list
的递归函数,它接受一个列表列表,listoflists
是它的参数。如果列表列表包含单个元素,则按原样返回。
否则,使用带有 isinstance()
的 if 语句来检查列表列表中第 0 个位置的列表是否为列表。
如果为真,那么我们返回 [0]
处子列表的值并再次调用我们的函数 to1list
,但这一次,跳过第 0 个位置并通过指定切片并传递子列表从第一个位置开始-list 作为参数。
现在让我们看看如何使用内置函数将嵌套列表连接到列表中。
使用内置 Python 函数将列表的列表连接为列表
我们可以使用 sum() 函数和 lambda 函数来连接 Python 中的列表列表。
使用 sum()
函数将列表的列表连接为列表
你可以使用内置的 sum 函数从列表列表中创建一维列表。
listoflists = [
["Sahildeep", "Dhruvdeep"],
[14.12, 8.6, 14.01],
[100, 100],
] # List to be flattened
mylist = sum(listoflists, [])
print("List of Lists:", listoflists)
print("My List:", mylist)
输出:
List of Lists: [['Sahildeep', 'Dhruvdeep'], [14.12, 8.6, 14.01], [100, 100]]
My List: ['Sahildeep', 'Dhruvdeep', '14.12', '8.6', '14.01', '100', '100']
在上面的程序中,sum 函数传递了两个参数;第一个是它将迭代的列表列表,第二个是我们最初为空的一维列表的开始。
使用 Lambda 函数加入列表列表
你还可以使用 lambda(即匿名函数)将列表列表转换为列表。让我们看一个代码示例:
listoflists = [
["Sahildeep", "Dhruvdeep"],
[14.12, 8.6, 14.01],
[100, 100],
] # List to be flattened
to1list = (
lambda listoflists: [element for item in listoflists for element in to1list(item)]
if type(listoflists) is list
else [listoflists]
)
print("List of lists:", listoflists)
print("MyList:", to1list(listoflists))
输出:
List of lists: [['Sahildeep', 'Dhruvdeep'], [14.12, 8.6, 14.01], [100, 100]]
MyList: ['Sahildeep', 'Dhruvdeep', 14.12, 8.6, 14.01, 100, 100]
在上面的程序中,我们定义了一个 lambda 函数,它迭代项目的每个元素,即列表列表中的子列表,并使用 to1list(item)
将该元素添加到单个列表中。
让我们看看如何使用 Python 库将列表列表转换为一维列表。
使用 Functools 将列表的列表连接为列表
在 Python 中将二维列表转换为一维列表的最快方法之一是使用其 Functools 模块,该模块提供的函数可以有效地处理更高或复杂的函数。
我们可以使用 Functools 模块中提供的 reduce()
函数将一个列表加入到一个列表中,如下代码所示:
import operator
import functools
listoflists = [["Sahildeep", "Dhruvdeep"], [14.12, 8.6, 14.01], [100, 100]]
# mylist using functools
mylist = functools.reduce(operator.iconcat, listoflists, [])
print("List of Lists", listoflists)
print("1-D list", mylist)
输出:
List of Lists [['Sahildeep', 'Dhruvdeep'], [14.12, 8.6, 14.01], [100, 100]]
1-D list ['Sahildeep', 'Dhruvdeep', 14.12, 8.6, 14.01, 100, 100]
在上面的代码片段中,新的一维列表是用 reduce()
函数定义的,该函数使用 operator.iconcat
函数连接我们从左到右移动的列表列表的子列表中的所有元素.
最好记住为 functools
和 operator
添加导入,因为这种方法需要两者。
使用 Chain()
方法将列表的列表连接为列表
另一个 Python 库,称为 Itertools
,包含一个 chain()
方法,你可以使用该方法将列表列表转换为一维列表。让我们看看如何:
import itertools
listoflists = [
["Sahildeep", "Dhruvdeep"],
[14.12, 8.6, 14.01],
[100, 100],
] # List to be flattened
mylist = list(itertools.chain(*listoflists))
print("List of Lists:", listoflists)
print("My List:", mylist)
输出:
List of Lists: [['Sahildeep', 'Dhruvdeep'], [14.12, 8.6, 14.01], [100, 100]]
My List: ['Sahildeep', 'Dhruvdeep', 14.12, 8.6, 14.01, 100, 100]
在上述程序中,chain()
方法用于迭代列表列表中的所有子列表,并返回包含所有子列表的所有元素的单个可迭代列表。
使用 Numpy 模块将列表的列表连接为列表
Numpy 库提供了连接子字符串并将它们展平为单个一维列表的函数。让我们看看如何使用 numpy.concatenate()
将嵌套列表转换为列表:
import numpy
listoflists = [
["Sahildeep", "Dhruvdeep"],
[14.12, 8.6, 14.01],
[100, 100],
] # List to be flattened
mylist = list(numpy.concatenate(listoflists).flat)
print("List of Lists:", listoflists)
print("My List:", mylist)
输出:
List of Lists: [['Sahildeep', 'Dhruvdeep'], [14.12, 8.6, 14.01], [100, 100]]
My List: ['Sahildeep', 'Dhruvdeep', '14.12', '8.6', '14.01', '100', '100']
在上面的代码片段中,我们使用了列表列表,与上面的程序相同,并使用 NumPy
的 concatenate()
和 flat()
函数创建了一个名为 mylist
的新列表。