Python NumPy numpy.concatenate() Function
-
Syntax of
numpy.concatenate()
-
Example Codes:
numpy.concatenate()
-
Example Codes:
numpy.concatenate()
to Pass a Multi-Dimensional Array -
Example Codes:
numpy.concatenate()
to Pass a Multi-Dimensional Array With theaxis
Parameter
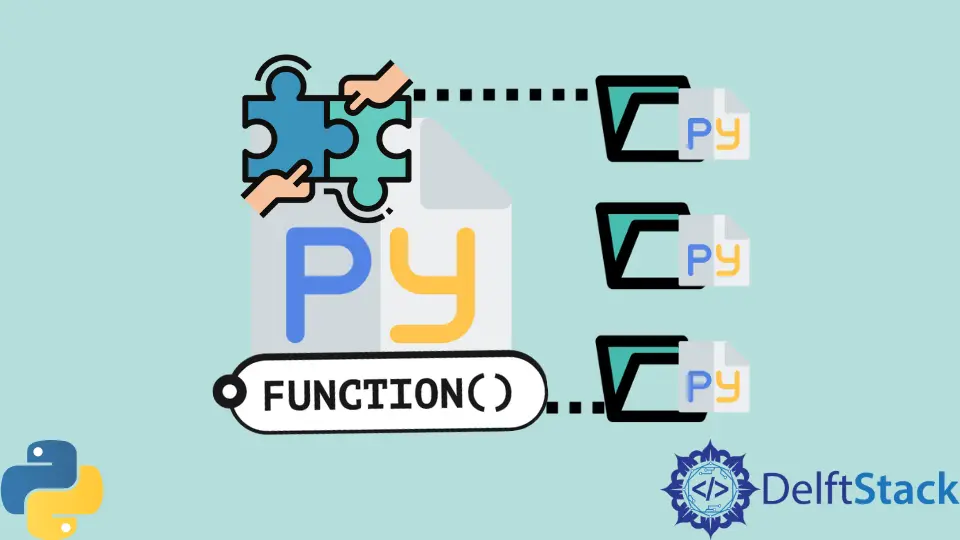
Python NumPy numpy.concatenate()
function concatenates multiple arrays over a specified axis. It accepts a sequence of arrays as a parameter and joins them into a single array.
Syntax of numpy.concatenate()
numpy.concatenate((a1, a2, ...), axis=0, out=None)
Parameters
a1, a2,... |
It is a sequence of an array-like structure. It is the sequence of input arrays to concatenate. The input arrays should have the same shape. |
axis |
It is an integer . It represents the axis over which the function will concatenate the arrays. Its default value is zero which means that the concatenation of arrays will be row-wise. If it is 1, the concatenation will be column-wise. |
out |
It is an N-dimensional array. It shows the final shape of the concatenated arrays. If it is provided then its shape must match with the output concatenated array. |
Return
It returns an N-dimensional array. This array shows the concatenation of input arrays.
Example Codes: numpy.concatenate()
We will concatenate a one-dimensional array first using numpy.concatenate
function.
import numpy as np
a1 = np.array([45, 12, 65, 78, 9, 34, 12, 11, 2, 65, 78, 82, 28, 78])
print("First array:")
print(a1)
a2 = np.array([89, 34, 56, 87, 90])
print("Second array:")
print(a2)
outarray = np.concatenate([a1, a2])
print("Concatenated array:")
print(outarray)
Output:
First array:
[45 12 65 78 9 34 12 11 2 65 78 82 28 78]
Second array:
[89 34 56 87 90]
Concatenated array:
[45 12 65 78 9 34 12 11 2 65 78 82 28 78 89 34 56 87 90]
The function has returned a concatenated array. If you want the output array to start with the second array, simply pass the second array first as a parameter.
Example Codes: numpy.concatenate()
to Pass a Multi-Dimensional Array
We will pass a multi-dimensional array now.
import numpy as np
a1 = np.array([[11, 12], [15, 10]])
print("First array:")
print(a1)
a2 = np.array([[10, 13], [15, 8]])
print("Second array:")
print(a2)
a3 = np.array([[11, 5], [34, 78]])
print("Third array:")
print(a3)
outarray = np.concatenate((a1, a2, a3))
print("Concatenated array:")
print(outarray)
Output:
First array:
[[11 12]
[15 10]]
Second array:
[[10 13]
[15 8]]
Third array:
[[11 5]
[34 78]]
Concatenated array:
[[11 12]
[15 10]
[10 13]
[15 8]
[11 5]
[34 78]]
The output shows that the input arrays are now joined together to form a single array.
Example Codes: numpy.concatenate()
to Pass a Multi-Dimensional Array With the axis
Parameter
Now, we will pass a sequence of multi-dimensional arrays as a parameter with axis
as 0. The resultant array will show the concatenation row-wise.
import numpy as np
a1 = np.array([[11, 12, 5], [15, 6, 10]])
print("First array:")
print(a1)
a2 = np.array([[10, 8, 13], [12, 15, 8]])
print("Second array:")
print(a2)
a3 = np.array([[11, 12, 5], [34, 78, 90]])
print("Third array:")
print(a3)
outarray = np.concatenate((a1, a2, a3))
print("Concatenated array:")
print(outarray)
Output:
First array:
[[11 12 5]
[15 6 10]]
Second array:
[[10 8 13]
[12 15 8]]
Third array:
[[11 12 5]
[34 78 90]]
Concatenated array:
[[11 12 5]
[15 6 10]
[10 8 13]
[12 15 8]
[11 12 5]
[34 78 90]]
Note that the output array shows the concatenation of arrays in an increasing rows manner as the default value for axis
is 0.
Now we will set the value of axis
to be 1.
import numpy as np
a1 = np.array([[11, 12, 5], [15, 6, 10]])
print("First array:")
print(a1)
a2 = np.array([[10, 8, 13], [12, 15, 8]])
print("Second array:")
print(a2)
a3 = np.array([[11, 12, 5], [34, 78, 90]])
print("Third array:")
print(a3)
outarray = np.concatenate((a1, a2, a3), axis=1)
print("Concatenated array:")
print(outarray)
Output:
First array:
[[11 12 5]
[15 6 10]]
Second array:
[[10 8 13]
[12 15 8]]
Third array:
[[11 12 5]
[34 78 90]]
Concatenated array:
[[11 12 5 10 8 13 11 12 5]
[15 6 10 12 15 8 34 78 90]]
The output array shows the concatenation in an increasing columns manner.