How to Check if Variable Is Integer Python
-
Use the
isinstance()
Method to Check if an Object Is anint
Type in Python -
Use the
int()
Method to Check if an Object Is anint
Type in Python -
Use the
float.is_integer()
Method to Check if an Object Is anint
Type in Python
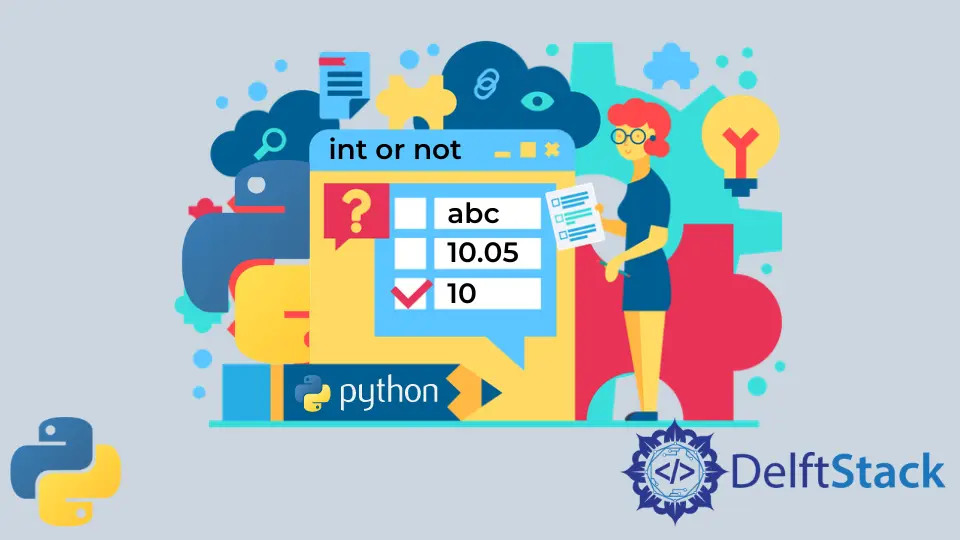
In Python, we have different datatypes when we deal with numbers. These are the int
, float
, and complex
datatypes.
The int
datatype is used to represent integers. In this tutorial, we will discuss how to check if a variable is int
or not.
In Python, we usually check the type()
function to return the type of the object. For example,
x = 10
print(type(x))
print(type(x) == int)
Output:
<class 'int'>
True
This method may work normally, but it blocks all flexibility of polymorphism. For example, if we subclass an object as int
and expect it to register as int
, this might fail with the type()
function.
The following code demonstrates this.
class def_int(int):
pass
x = def_int(0)
print(type(x))
print(type(x) == int)
Output:
<class '__main__.def_int'>
False
Use the isinstance()
Method to Check if an Object Is an int
Type in Python
It is therefore encouraged to use the isinstance()
function over the traditional type()
. The isinstance()
function is used to check whether an object belongs to the specified subclass. The following code snippet will explain how we can use it to check for int
objects.
y = 10
class def_int(int):
pass
x = def_int(0)
print(isinstance(y, int))
print(isinstance(x, int))
Output:
True
True
It is recommended to use the abstract base class numbers.Integral
, instead of the concrete int
class. In this way, we can check for int
, long
, and even the user-defined methods that can act as an integer.
Use the int()
Method to Check if an Object Is an int
Type in Python
We can create a simple logic also to achieve this using the int
function. For an object to be int
, it should be equal to the value returned by the int()
function when this object is passed to it. The following code demonstrates this.
x = 10.05
if int(x) == x:
print("True")
else:
print("False")
Output:
False
This method will return a Type-error when worked with complex objects.
Use the float.is_integer()
Method to Check if an Object Is an int
Type in Python
This method works only for float
objects. It checks if the value in a float object is an integer or not. For example,
x = 10.0
print(float.is_integer(x))
Output:
True
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn