How to Fix Error - IO.UnsupportedOperation: Not Writable Error in Python
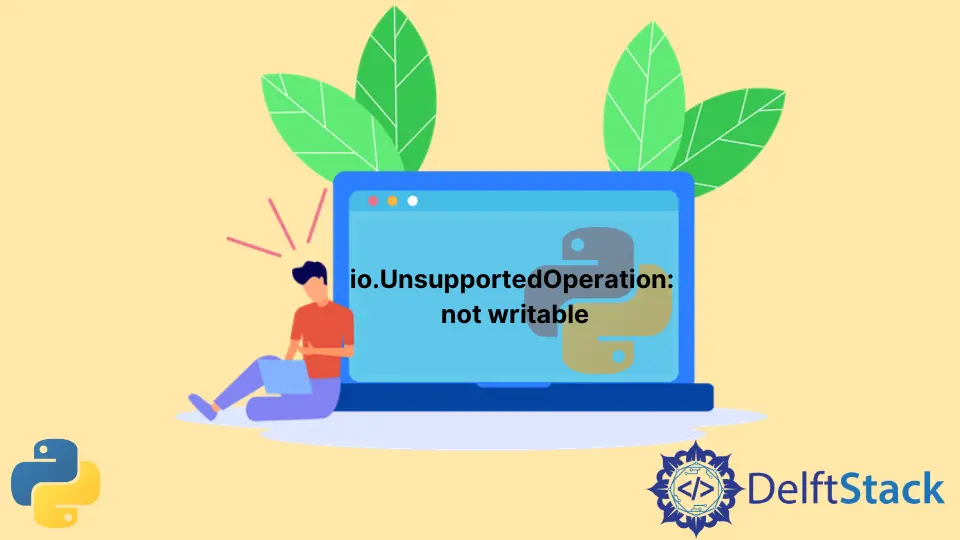
Python is very efficient in reading and writing data from files. It has a variety of functions to help in file handling.
The basics of file handling involve opening a file using the open()
function and reading or writing data based on the file mode.
The open()
opens a given file and creates a file object that can be used to perform reading and writing operations on a file.
The file can be opened in different types of modes. By default, it opens the file in read mode.
This tutorial will discuss the io.UnsupportedOperation: not writable
error in Python and ways to fix it.
Fix the io.UnsupportedOperation: not writable
Error in Python
This error is caused when we try to perform the write
operation on a file opened in reading mode. A file opened in read mode can only read the contents.
For example:
with open("sample.txt", "r") as f:
f.write("Text")
Output:
io.UnsupportedOperation: not writable
Note that in the above example, we open the file in r
mode (read) and try to write some data to this file using the write()
function, which causes the error.
Remember to open the file in modes that support this operation to solve this. The write (w
) or append (a
) modes are used to write some data to a file.
The previous contents are truncated if we open the file in w
mode. The a
mode adds content to the end of the file and preserves the previous data.
For example:
with open("sample.txt", "w") as f:
f.write("Text")
In the above example, we successfully avoid errors and can write data to the file.
If we want to simultaneously read and write data from a file, we can use the r+b
mode. We can perform reading and writing operations in binary mode when the file is opened in this mode.
For example:
with open("sample.txt", "r+b") as f:
f.write(bytes("Text", "utf-8"))
Note that we write data as bytes since the file is opened in binary mode. The text is encoded as bytes in the utf-8
encoding in the above example.
Alternatively, we can also use the writable()
function to check whether we can perform writing operations using the file handle or not. It returns True
or False
.
See the code below.
with open("sample.txt", "r") as f:
print(f.writable())
with open("sample.txt", "a") as f:
print(f.writable())
Output:
False
True
The above example shows that the writable
function returns False
when the file is opened in r
mode and returns True
when the file is opened in a
mode.
Conclusion
To conclude, we discussed the cause behind the io.UnsupportedOperation: not writable
error and how to fix it. We discussed how opening the file in the wrong mode can cause this and what file modes support writing operations.
We also demonstrated the use of the writable
function that can be used to check whether a file object can perform writing operations or not.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedInRelated Article - Python Error
- Can Only Concatenate List (Not Int) to List in Python
- How to Fix Value Error Need More Than One Value to Unpack in Python
- How to Fix ValueError Arrays Must All Be the Same Length in Python
- Invalid Syntax in Python
- How to Fix the TypeError: Object of Type 'Int64' Is Not JSON Serializable
- How to Fix the TypeError: 'float' Object Cannot Be Interpreted as an Integer in Python