How to Fix Python IndentationError: Unindent Does Not Match Any Outer Indentation Level
- The Indentation Rule in Python
-
Causes of
IndentationError
in Python -
Fix the
IndentationError: unindent does not match any outer indentation level
in Python
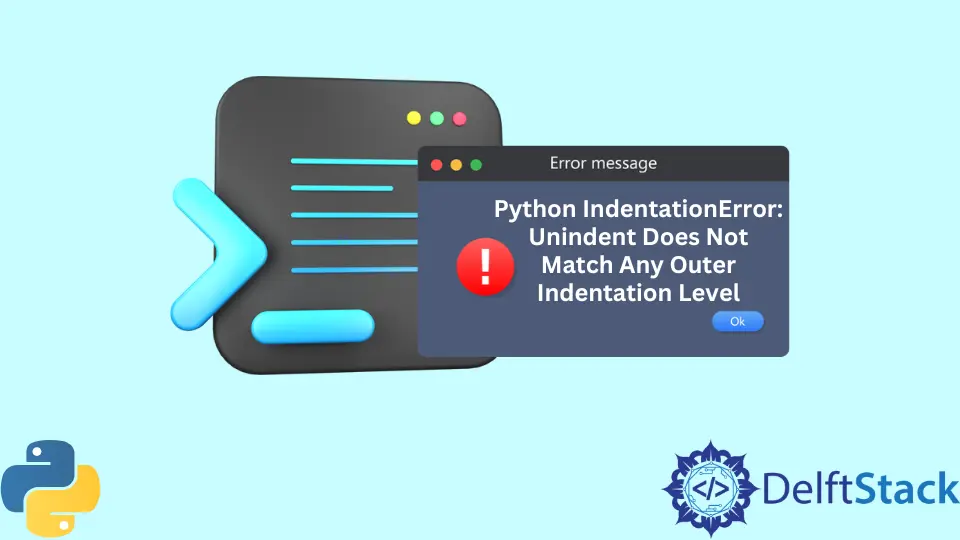
In the article, we will learn how to resolve the IndentationError
that occurs during the execution of the code. We will look at the different reasons that cause this error.
We will also find ways to resolve this error in Python. Let’s begin with what the IndentationError
is in Python.
The Indentation Rule in Python
The indentation is an essential part of the Python language. It depends upon indentation to define code blocks in its code.
While indentation can be done using both spaces and tabs. It is very easy for a developer to spot the flow of the code because of the indentation.
You can easily spot where a specific method/condition starts and ends.
Causes of IndentationError
in Python
Use of Both Spaces and Tabs
If you use both space and tab for indentation in the Python code, the IDE will throw an IndentationError
. While this is usually the case for these errors, they may also come up if you fail to use indentation in some chunk of code.
As we mentioned above, there are two ways to indent your code: tab or spaces. In Python, the standard of every indentation level is four spaces or one tab.
While this way of indenting is the rule of law for Python, it is also a visual aid for Python developers who can keep track of their code constructs because of this indentation.
Example Code:
def exploring_indentation():
number = 9
if number == 9:
print("Indentation works like this!")
exploring_indentation()
The output of the code:
Indentation works like this!
Indentation Error Due to Extra Indent in the Code
Another reason for an IndentationError
may be an extra indent added like in the example code below.
Example code:
def exploring_indentation():
number = 9
if number == 9:
print("Indentation works like this!")
else:
print("this is an example of an unnecessary indent")
exploring_indentation()
The output of the code:
File "<tokenize>", line 5
else:
^
IndentationError: unindent does not match any outer indentation level
The else
portion of the code is inside the if
condition because the indent is wrong.
Fix the IndentationError: unindent does not match any outer indentation level
in Python
Consistent Use of Spaces and Tabs
While keeping track of indentation sounds tedious enough, there are code editors like PyCharm and VS Code that have built-in features to help you keep track of indentation.
While writing code, when you hit Enter to move on to the following line, the editor will automatically indent your following line of code.
Moreover, if you mix tabs and spaces in your code, you can always find the option of converting tabs to spaces or vice versa.
For example, in PyCharm, you must go to Edit
in the top left corner, select the Convert Indents
option, and choose To Tabs
or To Spaces
as per your requirement.
Match the Outer Indent Level
In the below code given in the example, the if
and elif
statements are assigned with no indent. At the same time, the else
statement is assigned with an extra indent.
Due to this extra indent in the outer level, the Python compiler cannot recognize the else
statement and thus gives the IndentationError
(unindent does not match any outer indentation level).
Example code:
a = int(input("Enter an integer A: "))
b = int(input("Enter an integer B: "))
if b > a:
print("B is greater than A")
elif a == b:
print("A and B are equal")
else:
print("A is greater than B")
The output of the code:
File "<string>", line 7
else:
^
IndentationError: unindent does not match any outer indentation level
Correct Example code:
a = int(input("Enter an integer A: "))
b = int(input("Enter an integer B: "))
if b > a:
print("B is greater than A")
elif a == b:
print("A and B are equal")
else:
print("A is greater than B")
These problems are easily fixable in Python-specific editors as they are made specifically to enhance the programming experience without worrying about minor mistakes like an indentation.
However, when using an IDE-like Notepad, you may want to be wary of tabs and spaces and keep track of indentation very minutely, as they don’t point these errors out until you have run the code.
We hope you find this article helpful in understanding how to fix the IndentationError
in Python.
My name is Abid Ullah, and I am a software engineer. I love writing articles on programming, and my favorite topics are Python, PHP, JavaScript, and Linux. I tend to provide solutions to people in programming problems through my articles. I believe that I can bring a lot to you with my skills, experience, and qualification in technical writing.
LinkedInRelated Article - Python Error
- Can Only Concatenate List (Not Int) to List in Python
- How to Fix Value Error Need More Than One Value to Unpack in Python
- How to Fix ValueError Arrays Must All Be the Same Length in Python
- Invalid Syntax in Python
- How to Fix the TypeError: Object of Type 'Int64' Is Not JSON Serializable
- How to Fix the TypeError: 'float' Object Cannot Be Interpreted as an Integer in Python