How to Generate All Permutations of a List in Python
- Using the itertools Library
- Recursive Function for Permutations
- Iterative Approach to Generate Permutations
- Conclusion
- FAQ
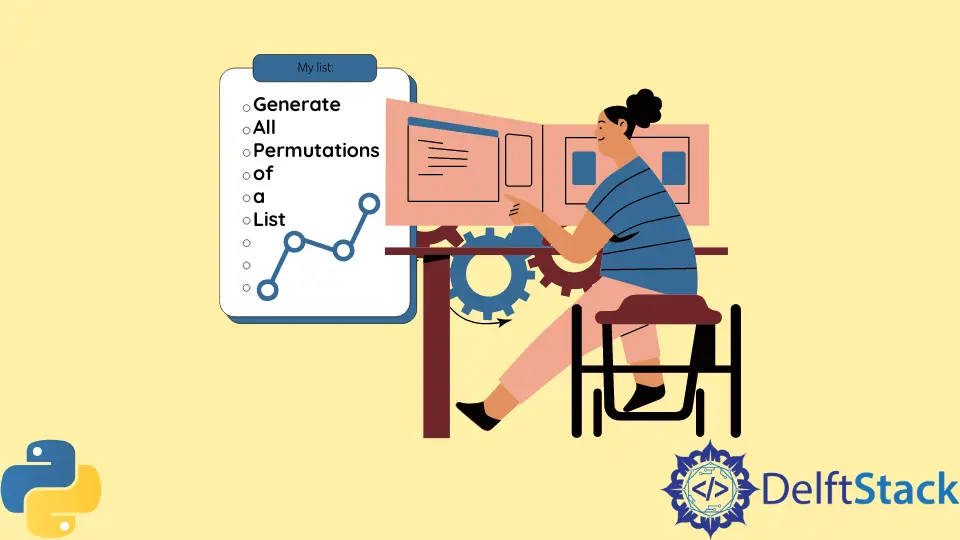
Generating all permutations of a list in Python can be a fascinating and useful task, especially for those delving into combinatorial algorithms or data analysis. Whether you’re looking to solve a problem in data science, create unique combinations for a game, or simply explore the capabilities of Python, understanding how to generate permutations is essential.
In this article, we will explore different methods to achieve this, using Python’s built-in libraries and some custom implementations. We’ll break down the code step by step, ensuring you grasp each concept along the way. So, let’s dive in and unlock the power of permutations in Python!
Using the itertools Library
One of the simplest and most efficient ways to generate permutations in Python is by using the itertools
library. This built-in library provides a method called permutations
, which can take a list and return all possible arrangements of its elements.
Here’s how you can use it:
import itertools
data = [1, 2, 3]
permutations = list(itertools.permutations(data))
for perm in permutations:
print(perm)
Output:
(1, 2, 3)
(1, 3, 2)
(2, 1, 3)
(2, 3, 1)
(3, 1, 2)
(3, 2, 1)
The itertools.permutations()
function generates all possible permutations of the input list. In the example above, we import the library and define a list of numbers. By passing this list to the permutations
function, we get an iterator of tuples, each representing a unique arrangement of the list elements. Converting this iterator to a list allows us to easily iterate through and print each permutation.
This method is efficient and straightforward, making it ideal for quick tasks or when working with smaller datasets. However, keep in mind that the number of permutations grows factorially with the size of the list, so performance may become an issue with larger lists.
Recursive Function for Permutations
If you want to understand the underlying mechanics of generating permutations, implementing a recursive function can be an enlightening experience. Recursion breaks down the problem into smaller sub-problems, allowing you to build permutations step by step.
Here’s a simple implementation:
def permute(data):
if len(data) == 1:
return [data]
permutations = []
for i in range(len(data)):
current = data[i]
remaining = data[:i] + data[i+1:]
for p in permute(remaining):
permutations.append([current] + p)
return permutations
data = [1, 2, 3]
result = permute(data)
for perm in result:
print(perm)
Output:
[1, 2, 3]
[1, 3, 2]
[2, 1, 3]
[2, 3, 1]
[3, 1, 2]
[3, 2, 1]
In this code, the permute
function checks if the input list has only one element. If so, it returns a list containing that element. If the list has more than one element, it iterates through each element, treating it as the current element, and recursively calls itself with the remaining elements. Each time a complete permutation is formed, it’s appended to the permutations
list.
This method provides a clear understanding of how permutations are constructed, although it may not be as efficient as using itertools
, especially for larger lists. The recursive approach can lead to a significant increase in function calls, making it less suitable for performance-critical applications.
Iterative Approach to Generate Permutations
An alternative to recursion is using an iterative approach to generate permutations. This method can be more intuitive for some, as it avoids the complexity of recursive calls.
Here’s how you can implement it:
def iterative_permute(data):
permutations = [[]]
for element in data:
new_permutations = []
for perm in permutations:
for i in range(len(perm) + 1):
new_permutations.append(perm[:i] + [element] + perm[i:])
permutations = new_permutations
return permutations
data = [1, 2, 3]
result = iterative_permute(data)
for perm in result:
print(perm)
Output:
[1, 2, 3]
[1, 3, 2]
[2, 1, 3]
[2, 3, 1]
[3, 1, 2]
[3, 2, 1]
In this code, we start with an empty list of permutations. For each element in the input list, we create new permutations by inserting the current element into every possible position of the existing permutations. This approach effectively builds up the list of permutations iteratively.
While this method is less commonly used than the recursive approach or the itertools
library, it can be a good fit for those who prefer to avoid recursion. It provides a clear, step-by-step construction of permutations, making it easier to follow.
Conclusion
Generating permutations in Python can be achieved through various methods, each with its strengths and weaknesses. The itertools
library offers a quick and efficient solution, while recursive and iterative approaches provide deeper insights into the mechanics of permutation generation. Depending on your needs—whether it’s performance, understanding, or simplicity—you can choose the method that best suits your project. By mastering these techniques, you can enhance your programming skills and tackle a variety of problems with confidence.
FAQ
-
What is a permutation?
A permutation is a rearrangement of the elements in a set. For example, the permutations of the list [1, 2] are [1, 2] and [2, 1]. -
How does the itertools library work in Python?
The itertools library provides functions that create iterators for efficient looping. The permutations function generates all possible arrangements of a given iterable. -
Can I generate permutations of a list with duplicate elements?
Yes, you can generate permutations of a list with duplicates, but it will result in repeated permutations. To get unique permutations, you can convert the result to a set. -
What is the time complexity of generating permutations?
The time complexity for generating permutations is O(n!), where n is the number of elements in the list, as the number of permutations grows factorially with the size of the input. -
Is there a limit to the size of the list for generating permutations?
While there is no strict limit, the number of permutations grows rapidly with the size of the list, making it impractical to generate permutations for large lists due to memory and processing constraints.
Related Article - Python List
- How to Convert a Dictionary to a List in Python
- How to Remove All the Occurrences of an Element From a List in Python
- How to Remove Duplicates From List in Python
- How to Get the Average of a List in Python
- What Is the Difference Between List Methods Append and Extend
- How to Convert a List to String in Python