Python でリストのすべての順列を生成する方法
Hassan Saeed
2023年1月30日
Python
Python List
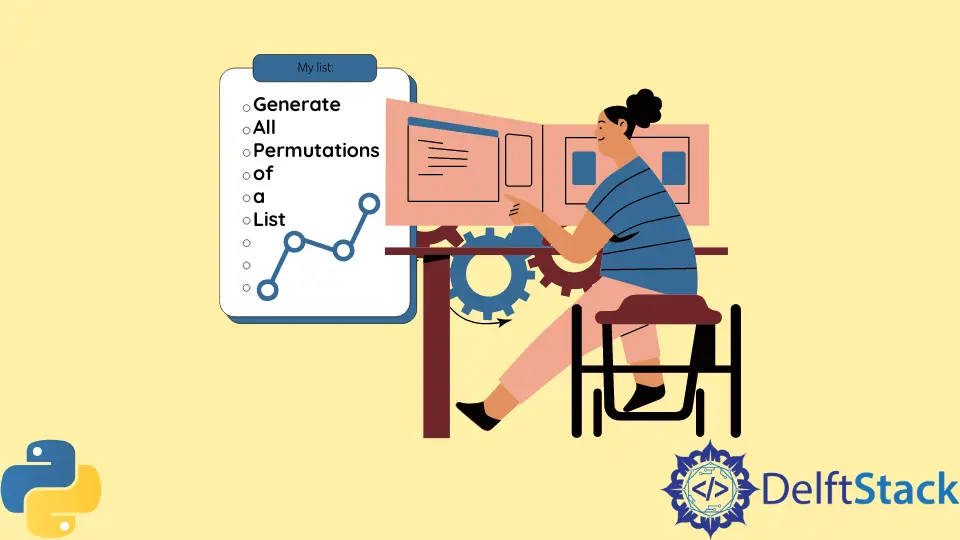
このチュートリアルでは、Python でリストのすべての順列を生成する方法について説明します。
Python でリストのすべての順列を生成するには itertools.permutations
を使用する
Python は、パーミュテーションを生成するための標準的なライブラリツールを提供しています:itertools.permutation
。以下の例は、これを使ってリストのすべての順列を生成する方法を示しています。
import itertools
inp_list = [4, 5, 6]
permutations = list(itertools.permutations(inp_list))
print(permutations)
出力:
[(4, 5, 6), (4, 6, 5), (5, 4, 6), (5, 6, 4), (6, 4, 5), (6, 5, 4)]
パーミュテーションのデフォルトの長さは、入力リストの長さに設定されています。しかし、itertools.permutations
関数呼び出しで並べ替えの長さを指定することができます。以下の例はこれを示しています。
import itertools
inp_list = [1, 2, 3]
permutations = list(itertools.permutations(inp_list, r=2))
print(permutations)
出力:
[(4, 5), (4, 6), (5, 4), (5, 6), (6, 4), (6, 5)]
以下の例は、与えられたリストのすべての可能な長さの順列を生成する方法を示しています。
import itertools
inp_list = [1, 2, 3]
permutations = []
for i in range(1, len(inp_list) + 1):
permutations.extend(list(itertools.permutations(inp_list, r=i)))
print(permutations)
出力:
[(4,), (5,), (6,), (4, 5), (4, 6), (5, 4), (5, 6), (6, 4), (6, 5), (4, 5, 6), (4, 6, 5), (5, 4, 6), (5, 6, 4), (6, 4, 5), (6, 5, 4)]
Python でリストのすべての順列を生成するために再帰を使用する
Python では、リストのすべての順列を生成するために再帰を使用することもできます。以下の例はこれを示しています。
def permutations(start, end=[]):
if len(start) == 0:
print(end)
else:
for i in range(len(start)):
permutations(start[:i] + start[i + 1 :], end + start[i : i + 1])
permutations([4, 5, 6])
出力:
[4, 5, 6]
[4, 6, 5]
[5, 4, 6]
[5, 6, 4]
[6, 4, 5]
[6, 5, 4]
チュートリアルを楽しんでいますか? <a href="https://www.youtube.com/@delftstack/?sub_confirmation=1" style="color: #a94442; font-weight: bold; text-decoration: underline;">DelftStackをチャンネル登録</a> して、高品質な動画ガイドをさらに制作するためのサポートをお願いします。 Subscribe