How to Find the Index of an Item in Python List
-
Python List Index Finding With the
index()
Method -
Python List Index Finding With the
for
Loop Method - Python List Index Instances Finding With the Iterative Method
- Python List Index Finding With the List Comprehension Method
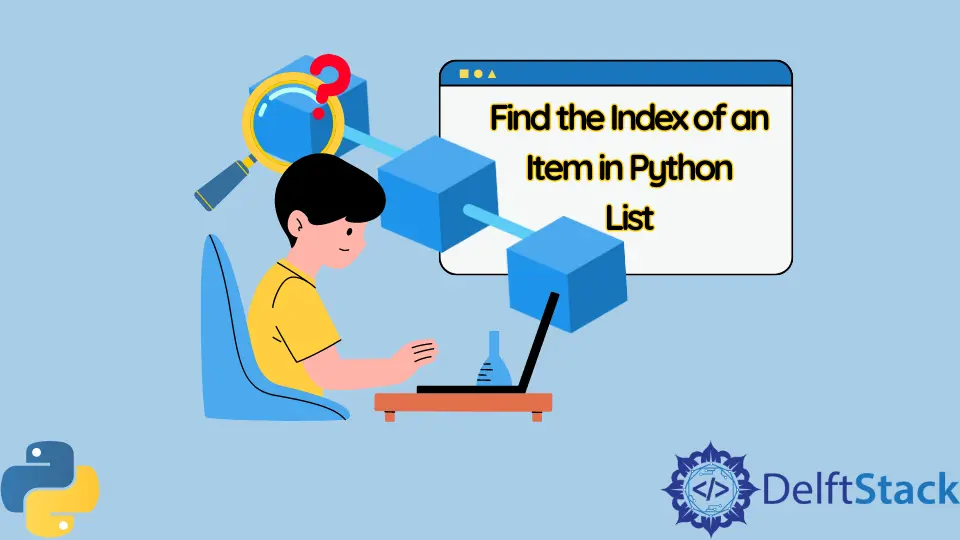
In Python, list elements are arranged in sequence. We can access any element in the list using indexes. Python list index starts from 0.
This article will discuss different methods to find the index of an item in the Python list.
Python List Index Finding With the index()
Method
The Syntax is:
list.index(x, start, end)
Here, start
and end
are optional. x
is the element that we need to find in the list.
Let’s see the example below.
consonants = ["b", "f", "g", "h", "j", "k"]
i = consonants.index("g")
print("The index of g is:", i)
Output:
The index of g is: 2
Be aware that the index()
method only returns the index of the first occurrence of the specified element.
consonants = ["b", "f", "g", "h", "j", "g"]
i = consonants.index("g")
print("The index of g is:", i)
Output:
The index of g is: 2
It has two g
in the list, and the result shows the index of the first g
.
If an element doesn’t exist in the list, it will generate ValueError
.
consonants = ["b", "f", "g", "h", "j", "k"]
i = consonants.index("a")
print("The index of a is:", i)
Output:
ValueError: 'a' is not in list
Python List Index Finding With the for
Loop Method
To find the index of an element in the list in Python, we can also use the for
loop method.
The code is:
consonants = ["b", "f", "g", "h", "j", "k"]
check = "j"
position = -1
for i in range(len(consonants)):
if consonants[i] == check:
position = i
break
if position > -1:
print("Element's Index in the list is:", position)
else:
print("Element's Index does not exist in the list:", position)
Output:
Element's Index in the list is: 4
Python List Index Instances Finding With the Iterative Method
If we need to find all the indices of the specified element’s occurrences in the list in Python, we have to iterate the list to get them.
The code is:
def iterated_index(list_of_elems, element):
iterated_index_list = []
for i in range(len(consonants)):
if consonants[i] == element:
iterated_index_list.append(i)
return iterated_index_list
consonants = ["b", "f", "g", "h", "j", "k", "g"]
iterated_index_list = iterated_index(consonants, "g")
print('Indexes of all occurrences of a "g" in the list are : ', iterated_index_list)
Output is:
Indexes of all occurrences of a "g" in the list are : [2, 6]
Python List Index Finding With the List Comprehension Method
We can get the same result as the previous method by using the list comprehension method.
The code is:
consonants = ["b", "f", "g", "h", "j", "k", "g"]
iterated_index_position_list = [
i for i in range(len(consonants)) if consonants[i] == "g"
]
print(
'Indexes of all occurrences of a "g" in the list are : ',
iterated_index_position_list,
)
Output is:
Indexes of all occurrences of a "g" in the list are : [2, 6]
Related Article - Python List
- How to Convert a Dictionary to a List in Python
- How to Remove All the Occurrences of an Element From a List in Python
- How to Remove Duplicates From List in Python
- How to Get the Average of a List in Python
- What Is the Difference Between List Methods Append and Extend
- How to Convert a List to String in Python