파이썬 목록에서 항목의 인덱스를 찾는 방법
Azaz Farooq
2023년10월10일
Python
Python List
-
index()
메소드로 파이썬 목록 인덱스 찾기 -
for
루프 메서드를 사용하여 파이썬 목록 인덱스 찾기 - 반복 방법으로 찾는 Python 목록 인덱스 인스턴스
- 리스트 내포 방법으로 파이썬 목록 인덱스 찾기
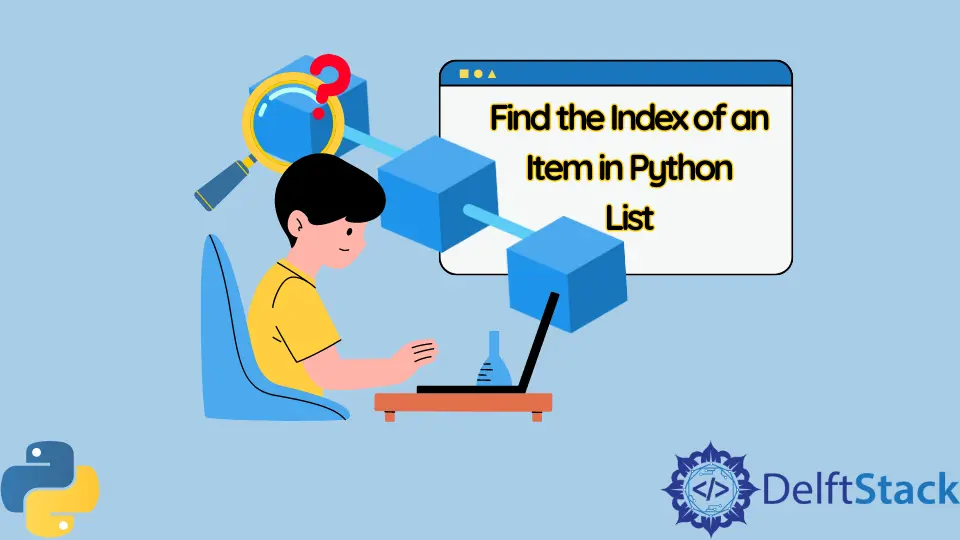
Python에서는 목록 요소가 순서대로 정렬됩니다. 인덱스를 사용하여 목록의 모든 요소에 액세스 할 수 있습니다. Python 목록 색인은 0부터 시작합니다.
이 기사에서는 Python 목록에서 항목의 색인을 찾는 다양한 방법에 대해 설명합니다.
index()
메소드로 파이썬 목록 인덱스 찾기
구문은 다음과 같습니다.
list.index(x, start, end)
여기서start
와end
는 선택 사항입니다. x
는 목록에서 찾아야하는 요소입니다.
아래 예를 보겠습니다.
consonants = ["b", "f", "g", "h", "j", "k"]
i = consonants.index("g")
print("The index of g is:", i)
출력:
The index of g is: 2
index()
메서드는 지정된 요소가 처음 나타나는 색인 만 반환합니다.
consonants = ["b", "f", "g", "h", "j", "g"]
i = consonants.index("g")
print("The index of g is:", i)
출력:
The index of g is: 2
목록에 두 개의 g
가 있으며 결과는 첫 번째 g
의 색인을 보여줍니다.
목록에 요소가 없으면 ValueError
가 생성됩니다.
consonants = ["b", "f", "g", "h", "j", "k"]
i = consonants.index("a")
print("The index of a is:", i)
출력:
ValueError: 'a' is not in list
for
루프 메서드를 사용하여 파이썬 목록 인덱스 찾기
Python의 목록에서 요소의 인덱스를 찾으려면for
루프 메서드를 사용할 수도 있습니다.
코드는 다음과 같습니다.
consonants = ["b", "f", "g", "h", "j", "k"]
check = "j"
position = -1
for i in range(len(consonants)):
if consonants[i] == check:
position = i
break
if position > -1:
print("Element's Index in the list is:", position)
else:
print("Element's Index does not exist in the list:", position)
출력:
Element's Index in the list is: 4
반복 방법으로 찾는 Python 목록 인덱스 인스턴스
Python의 목록에서 지정된 요소의 발생 인덱스를 모두 찾아야한다면 목록을 반복하여 가져와야합니다.
코드는 다음과 같습니다.
def iterated_index(list_of_elems, element):
iterated_index_list = []
for i in range(len(consonants)):
if consonants[i] == element:
iterated_index_list.append(i)
return iterated_index_list
consonants = ["b", "f", "g", "h", "j", "k", "g"]
iterated_index_list = iterated_index(consonants, "g")
print('Indexes of all occurrences of a "g" in the list are : ', iterated_index_list)
출력은 다음과 같습니다.
Indexes of all occurrences of a "g" in the list are : [2, 6]
리스트 내포 방법으로 파이썬 목록 인덱스 찾기
목록 이해 방법을 사용하여 이전 방법과 동일한 결과를 얻을 수 있습니다.
코드는 다음과 같습니다.
consonants = ["b", "f", "g", "h", "j", "k", "g"]
iterated_index_position_list = [
i for i in range(len(consonants)) if consonants[i] == "g"
]
print(
'Indexes of all occurrences of a "g" in the list are : ',
iterated_index_position_list,
)
출력은 다음과 같습니다.
Indexes of all occurrences of a "g" in the list are : [2, 6]
튜토리얼이 마음에 드시나요? DelftStack을 구독하세요 YouTube에서 저희가 더 많은 고품질 비디오 가이드를 제작할 수 있도록 지원해주세요. 구독하다