如何在 Python 列表中查詢一個元素的索引
Azaz Farooq
2023年1月30日
Python
Python List
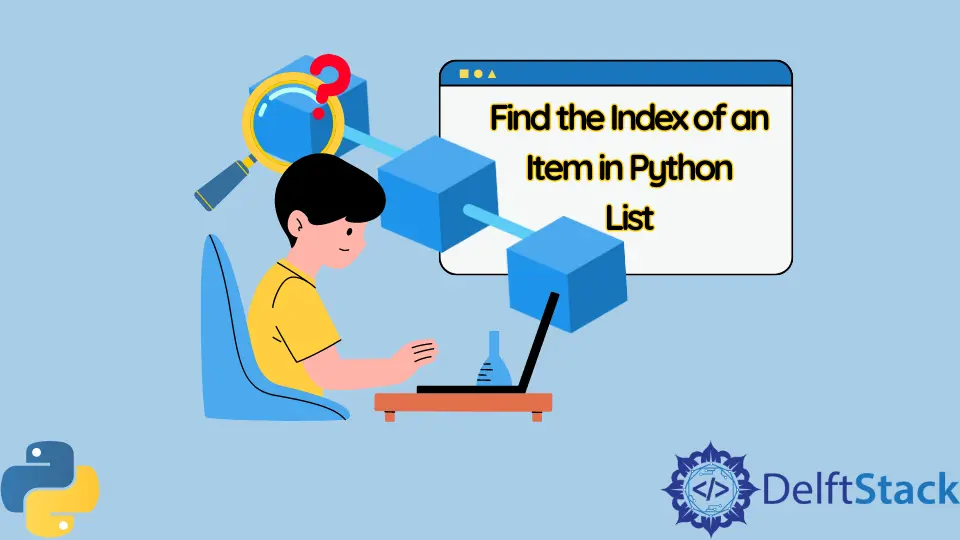
在 Python 中,列表元素是按順序排列的。我們可以使用索引來訪問列表中的任何元素。Python 列表索引從 0 開始。
本文將討論在 Python 列表中查詢元素索引的不同方法。
用 index()
方法查詢 Python 列表索引
語法是:
list.index(x, start, end)
這裡,start
和 end
是可選的。x
是我們需要在列表中找到的元素。
讓我們看下面的例子。
consonants = ["b", "f", "g", "h", "j", "k"]
i = consonants.index("g")
print("The index of g is:", i)
輸出:
The index of g is: 2
請注意,index()
方法只返回指定元素第一次出現的索引。
consonants = ["b", "f", "g", "h", "j", "g"]
i = consonants.index("g")
print("The index of g is:", i)
輸出:
The index of g is: 2
列表中有兩個 g
,結果顯示第一個 g
的索引。
如果一個元素在列表中不存在,它將產生 ValueError
。
consonants = ["b", "f", "g", "h", "j", "k"]
i = consonants.index("a")
print("The index of a is:", i)
輸出:
ValueError: 'a' is not in list
用 for
迴圈方法查詢 Python 列表索引
要在 Python 中找到列表中元素的索引,我們也可以使用 for
迴圈方法。
程式碼是:
consonants = ["b", "f", "g", "h", "j", "k"]
check = "j"
position = -1
for i in range(len(consonants)):
if consonants[i] == check:
position = i
break
if position > -1:
print("Element's Index in the list is:", position)
else:
print("Element's Index does not exist in the list:", position)
輸出:
Element's Index in the list is: 4
用迭代法查詢 Python 列表索引例項
如果我們需要在 Python 中找到指定元素在列表中出現的所有索引,我們必須對列表進行迭代才能得到它們。
程式碼是:
def iterated_index(list_of_elems, element):
iterated_index_list = []
for i in range(len(consonants)):
if consonants[i] == element:
iterated_index_list.append(i)
return iterated_index_list
consonants = ["b", "f", "g", "h", "j", "k", "g"]
iterated_index_list = iterated_index(consonants, "g")
print('Indexes of all occurrences of a "g" in the list are : ', iterated_index_list)
輸出:
Indexes of all occurrences of a "g" in the list are : [2, 6]
用列表推導法查詢 Python 列表索引的方法
我們可以通過使用列表推導法,得到與前一種方法相同的結果。
程式碼是:
consonants = ["b", "f", "g", "h", "j", "k", "g"]
iterated_index_position_list = [
i for i in range(len(consonants)) if consonants[i] == "g"
]
print(
'Indexes of all occurrences of a "g" in the list are : ',
iterated_index_position_list,
)
輸出:
Indexes of all occurrences of a "g" in the list are : [2, 6]
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe