How to Get List Shape in Python
-
Use the
len()
Function to Get the Shape of a One-Dimensional List in Python -
Use List Comprehensions and the
len()
Function to Get the Shape of Nested Lists in Python - Use NumPy to Get the Shape of Multi-Dimensional Lists in Python
- Use Recursion to Get the Shape of Irregularly Nested Lists in Python
- Conclusion
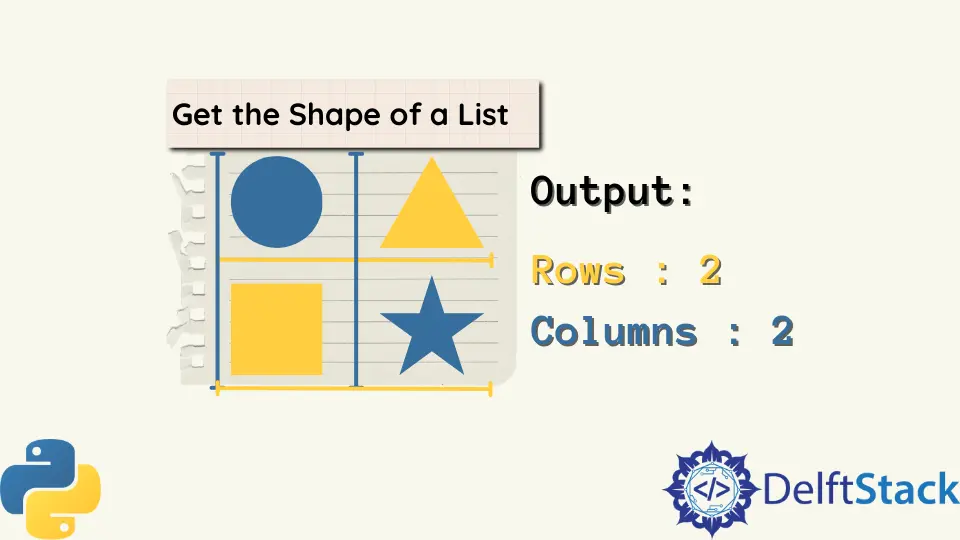
In Python, understanding the shape of a list is crucial when working with data structures, especially in scenarios involving multi-dimensional or nested lists.
This article explores various methods to determine the shape of a list in Python, ranging from simple one-dimensional lists to complex multi-dimensional or irregularly nested lists.
Use the len()
Function to Get the Shape of a One-Dimensional List in Python
The simplest method to get the shape of a list in Python is by using the len()
function. It provides the length (number of elements) of a list, effectively giving the size of the first dimension.
The len()
function has a simple and concise syntax:
len(object)
The object
is the object for which you want to determine the length. It can be a sequence (like a list, tuple, or string) or a collection (like a dictionary or set).
The following code example shows us how we can use the len()
method to get the shape of a list in Python.
my_list = [1, 2, 3, 4, 5]
length = len(my_list)
print("Length of the list:", length)
Output:
Length of the list: 5
In this example, the length of the list my_list
is computed, and it’s printed to the console.
Use List Comprehensions and the len()
Function to Get the Shape of Nested Lists in Python
In addition to determining the length of a flat (1D) list, we can also use the len()
function to determine the shape of nested lists (2D or higher-dimensional lists).
In the example below, we determine the number of rows and columns in a 2D nested list.
nested_list = [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
num_rows = len(nested_list)
# Assuming all sublists have the same number of columns
num_cols = len(nested_list[0])
print(f"Number of rows: {num_rows}, Number of columns: {num_cols}")
Output:
Number of rows: 3, Number of columns: 3
Here, num_rows
represents the number of rows (sublists), and num_cols
represents the number of columns (elements in each sublist) in the nested list.
Use NumPy to Get the Shape of Multi-Dimensional Lists in Python
If we want our code to work with any multi-dimensional lists, we can use NumPy’s .shape
attribute. It returns a tuple that contains the number of elements in each dimension of an array.
The NumPy package was originally designed to be used with arrays but could also work with lists. NumPy is an external package and does not come pre-installed with Python, so we need to install it before using it.
The command to install the NumPy
package is given below.
pip install numpy
The following code example shows how we can get the shape of a multi-dimensional list using NumPy.
import numpy as np
my_array = np.array([[1, 2, 3], [4, 5, 6]])
num_rows, num_cols = my_array.shape
print(f"Number of rows: {num_rows}, Number of columns: {num_cols}")
Output:
Number of rows: 2, Number of columns: 3
Here, a NumPy array my_array
is created, and then its shape is retrieved using the .shape
attribute. This method works seamlessly for multi-dimensional arrays, providing both the number of rows and columns.
Use Recursion to Get the Shape of Irregularly Nested Lists in Python
In some cases, we might encounter irregularly nested lists, where the depth of nesting varies, and sublists have different lengths. To handle such scenarios, we can use a recursive function.
def get_shape(data):
if isinstance(data, list):
return [len(data)] + get_shape(data[0])
else:
return [] # Assuming leaf elements are considered as a single column
nested_list = [[1, 2], [3, 4, 5], [6, [7, 8]]]
shape = get_shape(nested_list)
num_rows, num_cols = shape
print(f"Number of rows: {num_rows}, Number of columns: {num_cols}")
Output:
Number of rows: 3, Number of columns: 2
The get_shape()
function recursively navigates through the nested list, counting the number of rows in each dimension and assuming that leaf elements are considered as a single column. This approach provides both the number of rows and columns for irregularly nested lists.
Conclusion
The most straightforward approach to get the shape of a one-dimensional list in Python involves using the len()
function. For nested lists, list comprehensions along with the len()
function can help determine the number of rows and columns effectively.
For more advanced scenarios involving multi-dimensional arrays, NumPy’s .shape
attribute provides a convenient way to obtain the number of rows and columns. Finally, when dealing with irregularly nested lists where sublists have varying depths and lengths, a recursive function can be employed to determine the shape accurately.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedInRelated Article - Python List
- How to Convert a Dictionary to a List in Python
- How to Remove All the Occurrences of an Element From a List in Python
- How to Remove Duplicates From List in Python
- How to Get the Average of a List in Python
- What Is the Difference Between List Methods Append and Extend
- How to Convert a List to String in Python