Python NumPy numpy.shape() Function
-
Syntax of
numpy.shape()
-
Example Codes:
numpy.shape()
-
Example Codes:
numpy.shape()
to Pass a Simple Array -
Example Codes:
numpy.shape()
to Pass a Multi-Dimensional Array -
Example Codes:
numpy.shape()
to Call the Function Using Array’s Name
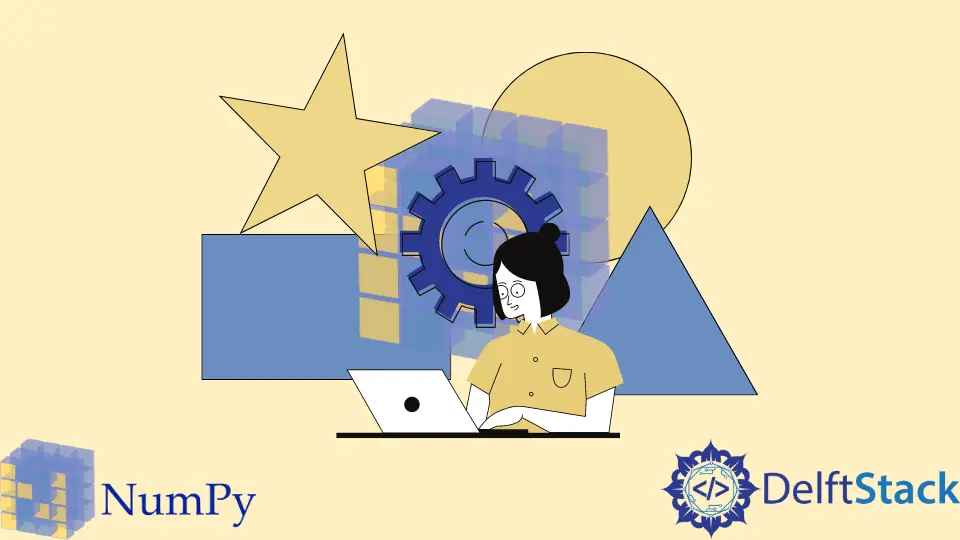
Python NumPy numpy.shape()
function finds the shape of an array. By shape
, we mean that it helps in finding the dimensions of an array. It returns the shape in the form of a tuple because we cannot alter a tuple just like we cannot alter the dimensions of an array.
Syntax of numpy.shape()
numpy.shape(a)
Parameters
a |
It is an array-like structure. It is the input array to find the dimensions. |
Return
It returns the shape of an array in the form of a tuple of integers. The values of the tuples show the length of the array dimensions.
Example Codes: numpy.shape()
The parameter a
is a mandatory parameter. If we execute this function on an empty array, it generates the following output.
import numpy as np
a = np.array([])
dimensions = np.shape(a)
print(dimensions)
Output:
(0,)
It has returned a tuple with a single integer - 0. It shows that the array is one dimensional with zero elements.
Example Codes: numpy.shape()
to Pass a Simple Array
We will pass a simple one-dimensional array now.
import numpy as np
a = np.array(
[89, 34, 56, 87, 90, 23, 45, 12, 65, 78, 9, 34, 12, 11, 2, 65, 78, 82, 28, 78]
)
dimensions = np.shape(a)
print(dimensions)
Output:
(20,)
The output shows that the array is one-dimensional and contains 20 elements.
Example Codes: numpy.shape()
to Pass a Multi-Dimensional Array
import numpy as np
a = np.array([[11, 12, 5], [15, 6, 10], [10, 8, 12], [12, 15, 8], [34, 78, 90]])
dimensions = np.shape(a)
print(dimensions)
Output:
(5, 3)
Note that the output tuple now contains two integer elements. It shows that the array contains five rows and three columns.
Now we will pass a more complex array.
import numpy as np
a = np.array(
[[[11, 12, 5], [15, 6, 10]], [[10, 8, 12], [12, 15, 8]], [[34, 78, 90], [4, 8, 10]]]
)
dimensions = np.shape(a)
print(dimensions)
Output:
(3, 2, 3)
We have passed an array that contains three arrays of 2-D arrays. The output tuple shows that the array has three layers, two rows, and three columns.
Example Codes: numpy.shape()
to Call the Function Using Array’s Name
We can call this function using the array’s name as well. It generates the same output. The following code snippets implement this function using the array’s name.
We will pass a one-dimensional array first.
import numpy as np
a = np.array(
[89, 34, 56, 87, 90, 23, 45, 12, 65, 78, 9, 34, 12, 11, 2, 65, 78, 82, 28, 78]
)
dimensions = a.shape
print(dimensions)
Output:
(20,)
Note that it has generated the same output as the output generated using the numpy.shape()
calling method.
import numpy as np
a = np.array(
[[[11, 12, 5], [15, 6, 10]], [[10, 8, 12], [12, 15, 8]], [[34, 78, 90], [4, 8, 10]]]
)
dimensions = a.shape
print(dimensions)
Output:
(3, 2, 3)