How to Fix Function Is Not Defined Error in Python
- Avoid Calling a Function Before Declaration in Python
- Avoid Using Misspelled Variables or Function Names in Python
- Avoid Using Built-In Modules Without Import in Python
- Fix Variable Out of Scope Issues in Python
- Conclusion
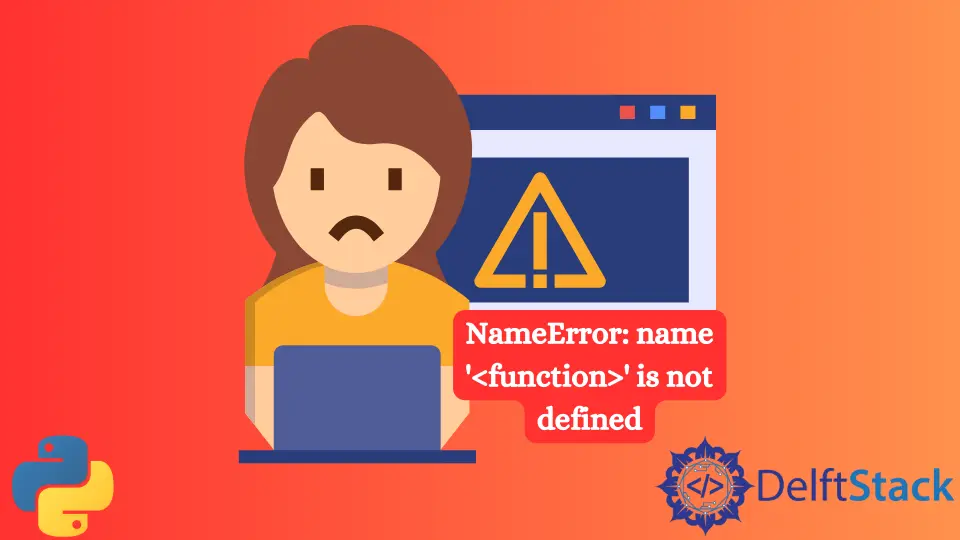
The NameError
in Python occurs when the interpreter encounters a name (function or variable) that it cannot find or recognize. This error can arise due to several reasons, such as attempting to call a function before declaring it, using misspelled variable or function names, accessing built-in functions without proper imports, or facing variable out-of-scope issues.
In this article, we will learn with what is the case when a Python program displays an error like a NameError: name '<functionName>' is not defined
even though the function is present in the script.
We will also learn what happens when we use misspelled variables or an inbuilt function without import and how to avoid these errors in Python.
Avoid Calling a Function Before Declaration in Python
There are many reasons to get the NameError: function is not defined
in Python, and we will discuss the most common reason for getting this error. When we call the function before defining it in our program, the Python interpreter cannot find the function definition.
In that case, the function will be unseen, and the Python interpreter will not yet encounter the function definition. Therefore, the Python interpreter will get confused and throw an error, meaning the function has not been defined according to the interpreter.
Let’s see a quick example of this.
Hello()
def Hello():
print("I will never be called")
We have written a call
statement in this program before defining a function. Since the interpreter executes the Python program line by line, it encounters a particular function call when it starts executing with the first line, but it does not know what Hello()
is.
When we run this Python script, it will be confused about whether it is a function, and the Python interpreter will stop and throw an error. This is because the definition of this function is present after the function call; that is why we can never invoke or call a function before defining it.
NameError: name 'Hello' is not defined
We need to define the function before calling it to fix this error.
Avoid Using Misspelled Variables or Function Names in Python
Another reason to get this error is when a user is making mistakes in defining the correct spelling of a function; that is why the user is getting this type of error. Python is case-sensitive, so lower and upper cases will be different functions.
We examine an example to demonstrate how the interpreter acts when it gets a misspelled variable.
Orange = "orange"
for i in orange:
print(i)
Output:
NameError: name 'orange' is not defined
When we run the Python script, we get an error that says 'orange' is not defined
, but this time we did not make a spelling mistake. We get this error even though we have defined this variable because we defined that variable that starts with a capital letter and is trying to access it with lowercase.
The same scenario will be applied with function. It works if we override the Orange
variable with orange
.
orange = "orange"
for i in orange:
print(i)
Output:
o
r
a
n
g
e
Avoid Using Built-In Modules Without Import in Python
Let’s say when you are writing a Python program, and if you want to use any of the inbuilt functions like print()
, input()
, etc., you can use it in your code. What happens when you need to use a function that is not built-in in Python but is present in certain modules?
To use a function that is part of certain modules, you must first import that module into your Python program. Some beginners fail when trying to use a function from a module instead of importing that specific module; look at an example.
In this program, the user tries to generate random values, but when the user runs this code, the user gets the same error we discussed. The reason is that the user gets failed when trying to access the random()
function without importing its module that is random
.
Randome_Values = random.random()
print(Randome_Values)
Output:
NameError: name 'random' is not defined
It works when the user imports a random
module in the Python script.
import random
Randome_Values = random.random()
print(Randome_Values)
Output:
0.07463088966802744
The output will be different every time since the random
module specializes in generating random values.
Sometimes, the users write the Python module themselves but face the issue and get the same error.
This is because the user is trying to call a function from another file after importing a class, but it may be possible the user did not save that file where the function is defined, which is why the user is getting this error. So make sure to save it before calling this function.
Fix Variable Out of Scope Issues in Python
Another reason most beginners fail is when they try to access a variable out of scope and get the same error. Let’s take a look at an example where we will get a better understanding.
def take_order():
orders = input("Enter your orders and separate it using comma :").split(",")
return orders
def Delete_Orders():
deleted_orders = input("Enter your order name which you want to cancel :")
orders.remove(deleted_orders)
return orders
print(take_order())
print(Delete_Orders())
When we run this Python script, the take_order()
function will work correctly, but the error occurs when we call the Delete_Orders()
function to delete the order from the orders
list.
NameError: name 'orders' is not defined
This is because the orders
variable is defined in the take_order()
function, and we are trying to access it from the Delete_Orders()
function. That is why when the execution control reaches out where we are removing the item from the orders
list, it throws an error because we are accessing it out of scope.
To fix this issue, we have to declare a variable that stores an empty string, and the variable name would be orders
. After that, we need to use the global
keyword, which we call an orders
variable, to make it reusable.
orders = ""
def take_order():
global orders
orders = input("Enter your orders and separate it using comma :").split(",")
return orders
def Delete_Orders():
deleted_orders = input("Enter your order name which you want to cancel :")
orders.remove(deleted_orders)
return orders
print(take_order())
print(Delete_Orders())
Output:
Enter your orders and separate it using comma :pizza,drink,water
['pizza', 'drink', 'water']
Enter your order name which you want to cancel :water
['pizza', 'drink']
Conclusion
This article has explored the common causes of the NameError
, providing practical examples and effective solutions.
Understanding the importance of defining functions before calling them, using consistent variable and function names, importing necessary modules, and managing variable scopes is crucial for writing robust and error-free Python code.
By avoiding common pitfalls, developers can mitigate NameError
issues and ensure the smooth execution of their programs.
Remember, meticulous coding practices and a keen understanding of scope and naming conventions are key to writing clean and reliable Python code.
Hello! I am Salman Bin Mehmood(Baum), a software developer and I help organizations, address complex problems. My expertise lies within back-end, data science and machine learning. I am a lifelong learner, currently working on metaverse, and enrolled in a course building an AI application with python. I love solving problems and developing bug-free software for people. I write content related to python and hot Technologies.
LinkedInRelated Article - Python Error
- Can Only Concatenate List (Not Int) to List in Python
- How to Fix Value Error Need More Than One Value to Unpack in Python
- How to Fix ValueError Arrays Must All Be the Same Length in Python
- Invalid Syntax in Python
- How to Fix the TypeError: Object of Type 'Int64' Is Not JSON Serializable
- How to Fix the TypeError: 'float' Object Cannot Be Interpreted as an Integer in Python