How to Find Element by Text Using Selenium in Python
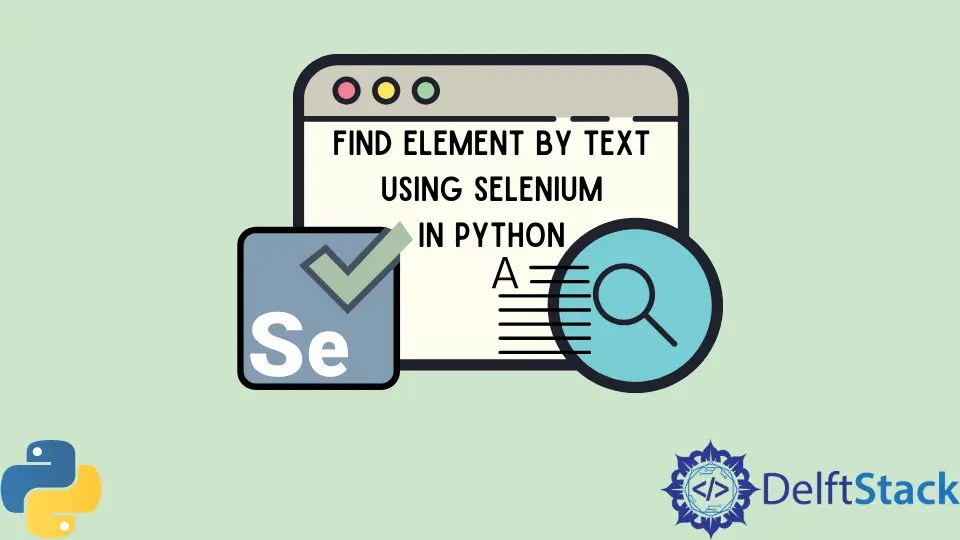
We will introduce the method to find elements by text using selenium in Python with examples.
Find Element by Text Using Selenium in Python
Software testing is a technique that checks whether an application meets the user’s requirements. The technique helps in making the application an error-free one.
Software testing is either done manually or through some software. Selenium is automation testing (an inbuilt Python library feature) that helps test an application before its publication.
This article discusses the steps to find the element by text through python selenium.
- First involved in this method is to import the python libraries, selenium, and time.
- Establish a connection with the web driver by using an executable path between the two.
- Go to the website from (URL of the application) where the text element is found.
- Wait a few moments for the entire webpage to load successfully.
- Now, find the required element by using text on the webpage.
Let’s have an example where we find the About Us
page of a website. First, we install selenium
by using the following command.
Command:
pip install selenium
Next, we install another library time
that we will use for wait purposes during webpage requests.
Command:
pip install time
Once we’ve installed all the libraries required, we import them into our code. Using the Chrome
driver, we will go to a website and try to find an element by text using the driver’s find_element_by_xpath()
method.
Full Code:
# python
from selenium import webdriver
from time import sleep
Chromedriver = webdriver.Chrome()
Chromedriver.get("https://inventicosolutions.com")
sleep(3)
Chromedriver.find_element_by_xpath(
"/html/body/header/div/nav[1]/div/ul/li[2]/a[contains(text(),'About Us')]"
).click()
sleep(3)
Output:
The output shows that it opens a new window in chrome and goes to the website. When our program finds the element, it clicks on it.
In this way, we can find the element by text using selenium in Python.
Rana is a computer science graduate passionate about helping people to build and diagnose scalable web application problems and problems developers face across the full-stack.
LinkedInRelated Article - Python Selenium
- How to Check if Element Exists Using Selenium Python
- How to Refresh Page in Python Selenium
- WebDriverException: Message: Geckodriver Executable Needs to Be in PATH Error in Python
- How to Install Python Selenium in macOS
- How to Login to a Website Using Selenium Python
- How to Open and Close Tabs in a Browser Using Selenium Python