How to Fix Error EOF While Parsing in Python
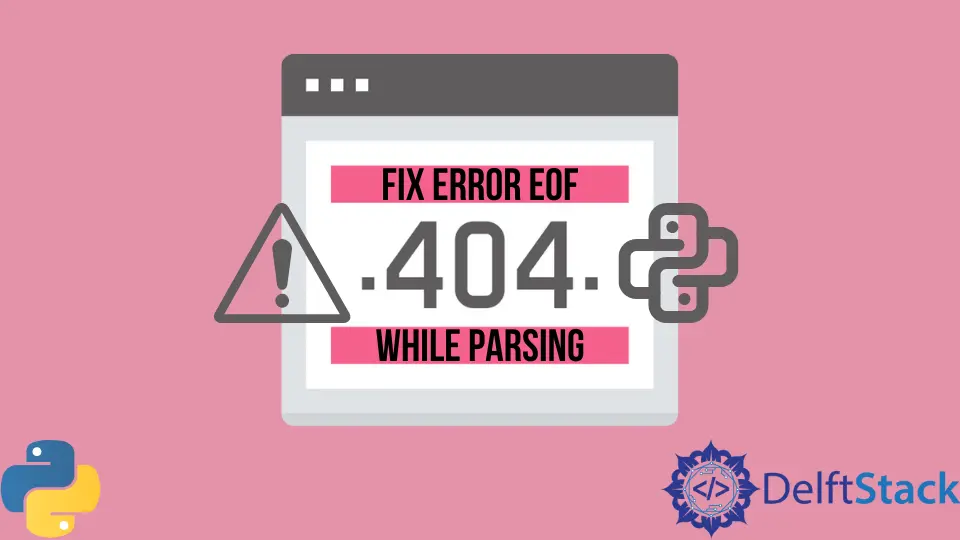
SyntaxError: unexpected EOF while parsing
occurs when an interpreter reaches the end of a file before executing a code block.
This is often a sign that some part of the code is missing. We need to locate the syntactical error and fix it.
Modern Python interpreters such as Pycharm would often highlight the code section that needs to be fixed. More often, resolving the error involves adding the section of the code that is missing.
Using for
loops often requires adding some code in the code. Failure to add some code in the body results in the unexpected EOF while parsing
error.
for
loops are often used to iterate over some iterable; in some scenarios, we may fail to add code to the body of the for
loop. This is one of the scenarios that result in such an error.
names = ['Tesla', 'Lucid', 'Samsung']
for company in names:
Output:
File "<string>", line 14
^
SyntaxError: unexpected EOF while parsing
According to the correct Python syntax, the compiler expects to execute some code after the indentation.
This new code in the indentation block forms part of the body of the for
loop. Therefore, we need to ensure that the loop’s body has some code to avert the unexpected end of the file and conform to the correct Python syntax.
We can fix this error, as shown in the code below.
names = ["Tesla", "Lucid", "Samsung"]
for company in names:
result = company.upper()
print(result)
Output:
TESLA
LUCID
SAMSUNG
This error may also come up when working with if-else
statements. if
statements are used to execute a block of code on a given condition; the indented block in an if
statement forms the body of all if
statements.
Apart from being used as a delimiter and defining functions, a full colon signifies the beginning of code blocks in if
statements. Failure to write any code in the block preceding the full colon would result in an EOF file while parsing errors
.
Below is an example demonstrating how this error comes about.
company_name = "tesla"
if company_name.upper() == True:
Output:
File "<string>", line 4
^
SyntaxError: unexpected EOF while parsing
This error can be fixed by ensuring that the if
statement has some code in its body, as shown below.
company_name = "tesla"
if company_name.isupper() == True:
print(company_name.lower())
else:
print(company_name.upper())
Output:
TESLA
The interpreter will equally raise the error EOF while parsing
if no code is written in the body of the else
statement that immediately follows an if
statement. This error will be thrown even though there is a valid code in the if
statement block.
Therefore, both code blocks should be complete to write a successful if-else
statement. Otherwise, we will get the error EOF while parsing
.
number = input("Please Enter a number: ")
if number % 2 == 0:
print("This is an even number:")
else:
Output:
File "<string>", line 7
^
SyntaxError: unexpected EOF while parsing
We need to ensure that the other part has a complete body to fix this error. Some if
statements do not require else
statements; in such a case, we don’t need to have any else
statements at all.
number = int(input("Please Enter a number: "))
if number % 2 == 0:
print("This is a even number")
else:
print("This is not an even number")
Output:
Please Enter a number: 23
This is not an even number
This error also occurs if the body of the while
loop is too defined. Similarly, the error can be fixed by ensuring that the body of the while
loop is provided.
i = 10
while i < 6:
Output:
File "<string>", line 3
^
SyntaxError: unexpected EOF while parsing
The error can also arise when working with functions if the function’s body is not provided.
In large programs with several functions, we may want to implement a function in the future. Thus, it would not be unnecessary to define the function’s body right away.
It may also be the case when one has yet to decide its functionality. The function below is supposed to calculate the sum of two numbers.
However, the body containing the statements that state what the function should do is not declared. This results in an error, as shown below.
def add_numbers(num1, num2):
Output:
File "<string>", line 3
^
SyntaxError: unexpected EOF while parsing
This error can be fixed by writing the statements that define the function, as shown in the example below.
def add_numbers(num1, num2):
result = num1 + num2
print(result)
add_numbers(23, 45)
Output:
68
If we do not want to specify what the function implements, we can also use the keyword pass
. The interpreter ignores the pass
statement, so nothing is executed.
We can also use this statement to avoid executing functions that we don’t want to implement immediately. The keyword pass
can be used to avert the error EOF while parsing
, as shown in the example below.
def add_numbers(num1, num2):
pass
Output:
Unexpected EOF While Parsing may also result from missing parentheses in functions or statements that make use of parentheses.
The print function and many other built-in Python functions are executed using parentheses. When writing code in a hurry, such small syntax errors can often be made unknowingly; this results in the error EOF while parsing
as shown in the example below.
def say_hello():
print("Hello user welcome on board"
Output:
File "<string>", line 2
^
SyntaxError: unexpected EOF while parsing
This error can only be resolved by ensuring that there are no parentheses left open.
Modern interpreters would often inform you that there is an error before proceeding to the next line. This error also extends to self-defined functions.
Improperly calling functions would also result in this error. The code below shows that an improper way of calling a self-defined function constitutes a failure to use both parentheses.
def add_sum(num1, num2):
print(num1 + num2)
add_sum(23, 78
Output:
File "e:\CodeBerry\eoferroinpython.py", line 8
^
SyntaxError: unexpected EOF while parsing
The Python try
and except
tests for the presence of errors in our code that could make our programs stop and handle them, respectively.
The try
block cannot function as a standalone method and should always accompany an except
block. Using the try
block to test a piece without specifying any code in the except
block results in the SyntaxError: unexpected EOF while parsing
as shown below.
number = int(input("Please enter a number:"))
def add_numbers(number):
try:
print(number + 2)
except ValueError:
Output:
File "<string>", line 7
^
SyntaxError: unexpected EOF while parsing
Isaac Tony is a professional software developer and technical writer fascinated by Tech and productivity. He helps large technical organizations communicate their message clearly through writing.
LinkedInRelated Article - Python Error
- Can Only Concatenate List (Not Int) to List in Python
- How to Fix Value Error Need More Than One Value to Unpack in Python
- How to Fix ValueError Arrays Must All Be the Same Length in Python
- Invalid Syntax in Python
- How to Fix the TypeError: Object of Type 'Int64' Is Not JSON Serializable
- How to Fix the TypeError: 'float' Object Cannot Be Interpreted as an Integer in Python