How to Get the Difference Between Two Lists in Python
-
Use the
in
Keyword to Find the Difference Between Two Lists in Python -
Use the
NumPy
Library to Find the Difference Between Two Lists in Python -
Use the
set.difference()
Method to Find the Difference Between Two Lists in Python -
Use the
set.symmetric_difference()
Method to Find the Difference Between Two Lists in Python - Conclusion
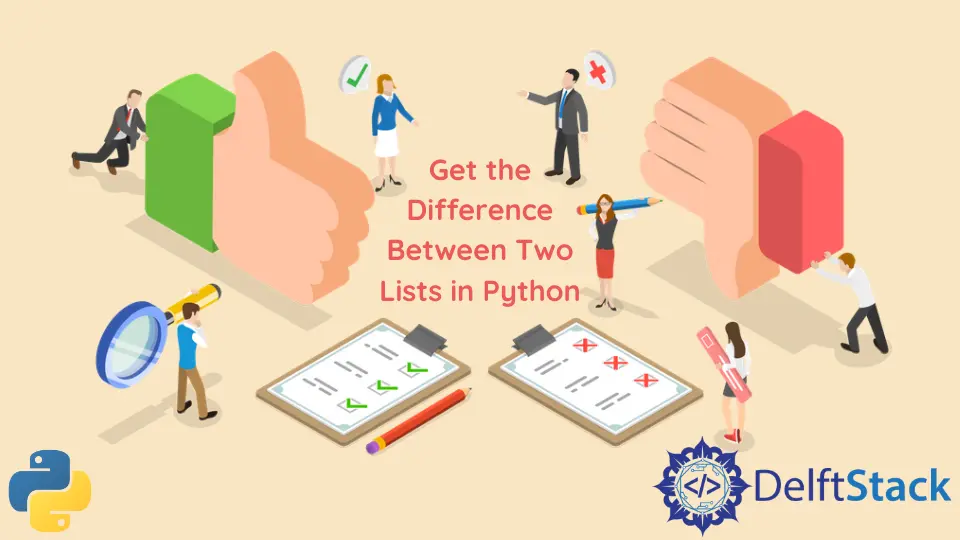
In Python, lists are an essential data structure that allows us to store and manipulate collections of items.
Often, we find ourselves in a situation where we need to compare two lists and determine the elements that exist in one list but not in the other. This process is known as finding the difference between two lists.
The problem is identifying the disparity between two lists in Python. By obtaining the difference between two lists, we can isolate the unique elements that distinguish one list from another.
This information can be valuable in various scenarios, such as data analysis, filtering, and data validation. Finding the difference between two lists can be particularly helpful when dealing with real-world examples.
Consider a scenario where we manage a customer database for an e-commerce platform. We have two lists: one containing the customers who made a purchase yesterday and another with the customers who made a purchase today.
By finding the difference between these two lists, we can quickly identify the new customers who made purchases today and target them for specific marketing campaigns or follow-up actions.
This tutorial will demonstrate several ways to get the difference between two lists in Python.
Use the in
Keyword to Find the Difference Between Two Lists in Python
The in
keyword helps check whether an element is present in an iterable sequence like a list. This method allows us to compare individual elements between the two lists and determine their inclusion or exclusion.
The append()
function is also used in this method. It is used to add elements to an existing list; it does not make a new list but modifies the initial list by adding elements.
By leveraging the simplicity and power of the in
keyword, we can easily obtain the unique elements between two lists in Python.
list_1 = [5, 10, 15, 20, 25, 30]
list_2 = [10, 20, 30, 40, 50, 60]
list_difference = []
for element in list_1:
if element not in list_2:
list_difference.append(element)
print(list_difference)
Output:
The provided code finds the difference between two lists, list_1
and list_2
, using a for
loop and the not in
condition. It initializes an empty list called list_difference
.
For each element in list_1
, it checks if the element is not present in list_2
. If the element is not found in list_2
, it is appended to the list_difference
. Finally, the list_difference
is printed, containing the elements in list_1
but not in list_2
.
This method’s drawback, though, is it doesn’t return the elements from the second list.
To make this method simpler, we can use list comprehension. List comprehension is a method that helps create a new list with the elements of an existing list.
list_1 = [5, 10, 15, 20, 25, 30]
list_2 = [10, 20, 30, 40, 50, 60]
list_difference = [element for element in list_1 if element not in list_2]
print(list_difference)
Output:
Use the NumPy
Library to Find the Difference Between Two Lists in Python
Python’s NumPy
Library is a very commonly used library. This library helps users perform arrays, matrices, and linear algebra tasks.
In addition to the previously discussed methods, we can leverage the powerful NumPy
library to find the difference between two lists in Python. The numpy.setdiff1d()
function efficiently computes the unique elements present in one list but not in another.
Syntax:
import numpy as np
list_difference = np.setdiff1d(list1, list2)
By importing NumPy and calling numpy.setdiff1d()
, we can directly obtain the unique elements from list1
that are not present in list2
. The resulting unique elements are stored in the list_difference
variable.
Using NumPy offers additional capabilities and performance optimizations compared to the previous methods. It is particularly beneficial when dealing with large datasets or when extensive numerical operations are involved.
This method uses three functions of the NumPy
library to get the difference between two lists. These three functions are np.array()
, np.setdiff1d()
, and np.concatenate()
.
The np.concatenate
function is used to combine two or more NumPy
arrays. Check the example below.
import numpy as np
list_1 = [5, 10, 15, 20, 25, 30]
list_2 = [10, 20, 30, 40, 50, 60]
array_1 = np.array(list_1)
array_2 = np.array(list_2)
difference_1 = np.setdiff1d(array_1, array_2)
difference_2 = np.setdiff1d(array_2, array_1)
list_difference = np.concatenate((difference_1, difference_2))
print(list(list_difference))
Output:
The above code uses the NumPy library to find the difference between list_1
and list_2
. It first converts the lists into NumPy arrays using np.array()
.
Then, it utilizes np.setdiff1d()
to calculate the unique elements present in array_1
but not in array_2
and vice versa. The resulting differences are stored in difference_1
and difference_2
.
Finally, the two sets of differences are concatenated using np.concatenate()
and converted back to a Python list, which is then printed as list_difference
.
Use the set.difference()
Method to Find the Difference Between Two Lists in Python
The set()
method helps the user convert any iterable to an iterable sequence, also called a set. The iterables can be a list, a dictionary, or a tuple.
Python provides the set.difference()
method that allows us to find the difference between two lists easily. By utilizing the set data structure, we can use its unique property to compute the different elements efficiently.
Syntax:
result = set(list1).difference(set(list2))
Here, list1
represents the original list from which we want to extract the unique elements, and list2
is the list whose elements we want to exclude from list1
. The set.difference()
function computes the elements present in list1
but not in list2
and stores the result in the result
variable.
The set.difference()
function returns the difference between the two sets. This function eliminates the common elements in two sets.
Compared to the previously discussed in
method and the NumPy approach, set.difference()
offers a simple and straightforward way to obtain the difference between two lists. It doesn’t require additional imports or array conversions.
By converting the lists to sets and using the set.difference()
method, we can directly obtain the unique elements in one list but not in the other.
Using this straightforward approach, we can quickly identify the distinct items between two lists in Python, enabling us to perform various operations on the unique elements as needed.
list_1 = [5, 10, 15, 20, 25, 30]
list_2 = [10, 20, 30, 40, 50, 60]
difference_1 = set(list_1).difference(set(list_2))
difference_2 = set(list_2).difference(set(list_1))
list_difference = list(difference_1.union(difference_2))
print(list_difference)
Output:
For this method, the first step is to store the two lists in two variables. The set()
function converts these lists into sets.
In the same step, the set.difference()
function is used to find the difference between the two sets. Note that the difference_1
variable gets the elements in list_1
and not in list_2
.
The difference_2
variable gets the elements in list_2
and not in list_1
. After that, the union
function is used to get all the elements from the difference_1
and difference_2
variables.
The list()
function also converts both sets into lists again. Finally, the resultant list is printed.
Use the set.symmetric_difference()
Method to Find the Difference Between Two Lists in Python
In Python, the set.symmetric_difference()
method provides a convenient way to find the difference between two lists. This method returns a new set that contains the elements present in either of the lists but not in both.
Syntax:
result = set(list1).symmetric_difference(set(list2))
Here, list1
and list2
represent the two lists for comparison. The set.symmetric_difference()
method takes the two lists converted to sets as input parameters and computes the symmetric difference between them.
The resulting elements existing in either list but not in both are stored in the result
set.
Compared to the previously discussed methods, set.symmetric_difference()
provides a comprehensive solution that includes elements unique to either list. It offers more flexibility by considering both lists’ elements and is suitable when identifying unique elements from both lists is desired.
list_1 = [5, 10, 15, 20, 25, 30]
list_2 = [10, 20, 30, 40, 50, 60]
set_difference = set(list_1).symmetric_difference(set(list_2))
list_difference = list(set_difference)
print(list_difference)
Output:
The above code uses the set.symmetric_difference()
method to find the difference between list_1
and list_2
. It first converts both lists into sets using the set()
function.
Then, it applies the set.symmetric_difference()
method to obtain the elements present in either of the sets but not in both. The resulting set of differences is stored in set_difference
.
Finally, the set_difference
is converted back to a list using the list()
function, and the list of differences is printed as list_difference
.
Conclusion
In this tutorial, we explored various approaches to find the difference between two lists in Python. We discussed methods such as using the in
keyword, NumPy’s setdiff1d()
, set.difference()
, and set.symmetric_difference()
.
Each method offers its advantages and is suitable for different scenarios.
The in
keyword approach is straightforward and intuitive. It allows for direct comparison between elements but may not be the most efficient approach for large lists since it requires iterating over each element.
NumPy’s setdiff1d()
offers performance optimizations and is ideal for large datasets or numerical operations scenarios. It operates on NumPy arrays and provides concise syntax. However, importing the NumPy library and converting the lists into arrays adds some overhead.
Using set.difference()
provides a simple and efficient way to find the difference between two lists. It leverages the set data structure and offers a concise syntax. However, it doesn’t preserve the order of elements and may not be suitable when maintaining the original order is crucial.
The set.symmetric_difference()
function provides a comprehensive solution by considering elements unique to either list. It offers flexibility but requires converting the lists into sets, which may alter the original order and remove duplicate elements.
The choice of method depends on the specific requirements of the problem at hand. If simplicity and efficiency are paramount and preserving the order is not crucial, set.difference()
is a good choice.
NumPy’s setdiff1d()
is advantageous for numerical operations or large datasets. If the goal is to find the elements unique to either list, set.symmetric_difference()
is suitable.
Lakshay Kapoor is a final year B.Tech Computer Science student at Amity University Noida. He is familiar with programming languages and their real-world applications (Python/R/C++). Deeply interested in the area of Data Sciences and Machine Learning.
LinkedInRelated Article - Python List
- How to Convert a Dictionary to a List in Python
- How to Remove All the Occurrences of an Element From a List in Python
- How to Remove Duplicates From List in Python
- How to Get the Average of a List in Python
- What Is the Difference Between List Methods Append and Extend
- How to Convert a List to String in Python