How to Convert Radians to Degrees and Vice-Versa in Python
- Understanding Radians and Degrees
- Method 1: Using the math Module
- Method 2: Custom Conversion Functions
- Method 3: Using NumPy for Vectorized Operations
- Conclusion
- FAQ
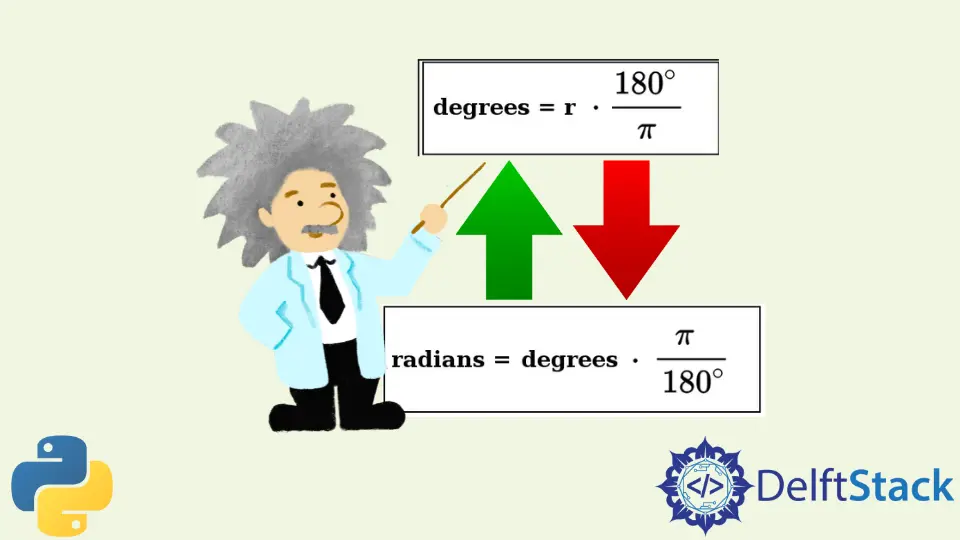
Converting between radians and degrees is a fundamental skill in programming, especially when dealing with trigonometric functions. Whether you’re working on a scientific application, game development, or data analysis, understanding how to convert these units is essential.
In this tutorial, we will explore how to convert radians to degrees and degrees to radians using Python. We’ll cover various methods, including built-in functions and custom implementations. By the end of this article, you will have a solid grasp of these conversions and be able to apply them in your own projects. Let’s dive in!
Understanding Radians and Degrees
Before we jump into the conversion methods, it’s crucial to understand what radians and degrees represent. Degrees are a measure of angle, with a full circle comprising 360 degrees. Radians, on the other hand, relate to the radius of a circle. One complete revolution equates to 2π radians. This relationship means that:
- 180 degrees = π radians
- 360 degrees = 2π radians
Knowing this, we can easily derive conversion formulas. The formula to convert radians to degrees is:
degrees = radians * (180 / π)
And to convert degrees to radians:
radians = degrees * (π / 180)
Now, let’s look at how to implement these conversions in Python.
Method 1: Using the math Module
Python’s built-in math
module provides a straightforward way to handle these conversions. By utilizing the math.radians()
and math.degrees()
functions, you can easily convert between radians and degrees without needing to implement the conversion formulas manually.
Here’s how to use these functions:
import math
# Convert degrees to radians
degrees = 90
radians = math.radians(degrees)
# Convert radians to degrees
radians_input = math.pi / 2
degrees_output = math.degrees(radians_input)
print(f"{degrees} degrees is {radians} radians.")
print(f"{radians_input} radians is {degrees_output} degrees.")
Output:
90 degrees is 1.5707963267948966 radians.
1.5707963267948966 radians is 90.0 degrees.
Using the math
module simplifies the process significantly. The math.radians()
function takes a degree value and converts it to radians, while math.degrees()
does the opposite. This approach is not only efficient but also leverages Python’s built-in capabilities, ensuring accuracy and reliability.
Method 2: Custom Conversion Functions
If you prefer to have more control over your conversions or want to avoid using external libraries, you can create your own functions to perform the conversions. This method allows you to understand the underlying mathematics better and can be a good exercise in function creation.
Here’s how you can implement custom conversion functions:
import math
def degrees_to_radians(degrees):
return degrees * (math.pi / 180)
def radians_to_degrees(radians):
return radians * (180 / math.pi)
# Example usage
degrees = 45
radians = degrees_to_radians(degrees)
radians_input = math.pi
degrees_output = radians_to_degrees(radians_input)
print(f"{degrees} degrees is {radians} radians.")
print(f"{radians_input} radians is {degrees_output} degrees.")
Output:
45 degrees is 0.7853981633974483 radians.
3.141592653589793 radians is 180.0 degrees.
In this example, we defined two functions: degrees_to_radians()
and radians_to_degrees()
. Each function takes a single input and applies the respective conversion formula. This method not only enhances your understanding of the conversion process but also allows for easy customization if needed in the future.
Method 3: Using NumPy for Vectorized Operations
If you’re dealing with arrays or large datasets, using the NumPy library can make your conversions even more efficient. NumPy allows for vectorized operations, meaning you can convert entire arrays of angles at once. This is particularly beneficial when working with scientific data or in machine learning applications.
Here’s how to use NumPy for these conversions:
import numpy as np
# Array of angles in degrees
degrees_array = np.array([0, 30, 45, 60, 90])
# Convert degrees to radians
radians_array = np.radians(degrees_array)
# Array of angles in radians
radians_input_array = np.array([0, np.pi/6, np.pi/4, np.pi/3, np.pi/2])
# Convert radians to degrees
degrees_output_array = np.degrees(radians_input_array)
print("Degrees to Radians:", radians_array)
print("Radians to Degrees:", degrees_output_array)
Output:
Degrees to Radians: [0. 0.52359878 0.78539816 1.04719755 1.57079633]
Radians to Degrees: [ 0. 30. 45. 60. 90.]
In this example, we utilized NumPy’s np.radians()
and np.degrees()
functions. These functions can handle arrays, allowing you to convert multiple values in one go. This method is not only faster but also cleaner, making it ideal for data analysis and scientific computations.
Conclusion
Converting between radians and degrees in Python is a straightforward process, thanks to the built-in functions provided by the math
module and libraries like NumPy. Whether you choose to use these built-in functions, create your own conversion methods, or leverage NumPy for array operations, understanding these conversions is essential for any programmer working with angles. By following the methods outlined in this tutorial, you can confidently perform these conversions in your projects.
FAQ
-
What is the difference between radians and degrees?
Radians and degrees are both units for measuring angles. Degrees are based on a full circle being 360 degrees, while radians are based on the radius of a circle, where a full circle is 2π radians. -
Why would I need to convert between radians and degrees in Python?
Many mathematical functions, especially trigonometric functions, require angles to be in a specific unit. Depending on the context, you may need to convert between radians and degrees to ensure accurate calculations. -
Can I use the conversion methods in other programming languages?
Yes, the conversion formulas are universal and can be implemented in any programming language. The methods shown in this tutorial are specific to Python, but the logic remains the same across languages. -
Is it necessary to import the math module for conversions?
While you can create your own functions to convert between radians and degrees, using themath
module simplifies the process and ensures accuracy. -
How does NumPy improve the conversion process?
NumPy allows for vectorized operations, enabling you to convert entire arrays of angles at once, which is more efficient and cleaner than processing each value individually.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn