How to Declare a Variable Without Value in Python
- Understanding Variable Declaration in Python
- Method 1: Using None
- Method 2: Using an Empty String
- Method 3: Using an Empty List
- Conclusion
- FAQ
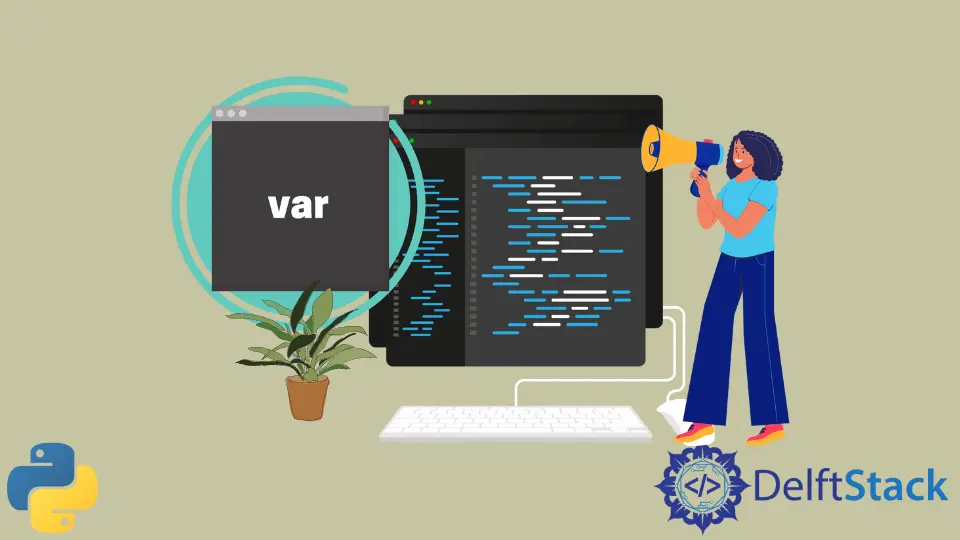
In the world of programming, understanding how to declare variables is fundamental. Python, known for its simplicity and readability, allows you to declare variables in various ways. But what if you want to declare a variable without immediately assigning a value?
This tutorial will guide you through the methods to declare a variable without a value in Python, helping you grasp this essential concept. Whether you’re preparing for a coding interview or just brushing up on your Python skills, this article will provide you with clear explanations and practical examples. Let’s dive into the world of Python variables!
Understanding Variable Declaration in Python
Before we explore how to declare a variable without a value, it’s essential to understand what a variable is in Python. A variable acts as a storage location in your program, allowing you to hold data that can be referenced later. In Python, you don’t need to specify a type when declaring a variable, making it flexible and easy to use.
When you declare a variable without assigning a value, you essentially create a placeholder. This can be useful in scenarios where you plan to assign a value later in your code. Let’s look at some methods to declare a variable without a value in Python.
Method 1: Using None
One of the most common ways to declare a variable without a value in Python is by using the None
keyword. None
is a special constant in Python that represents the absence of a value. By assigning None
to a variable, you can indicate that it currently holds no value.
my_variable = None
Output:
None
Using None
as a placeholder is clear and straightforward. It allows you to check whether the variable has been assigned a value later in your code. For instance, you can use conditional statements to determine if the variable is still None
before performing operations on it. This practice enhances code readability and prevents errors that might occur from trying to use uninitialized variables.
Furthermore, using None
can be particularly helpful in functions where you may want to provide default parameters. By setting a variable to None
, you can easily check if the user has provided a value or not, allowing for more flexible function behavior.
Method 2: Using an Empty String
Another method to declare a variable without a value is to initialize it with an empty string. This approach is especially useful when you expect the variable to hold string data later in your program.
my_string = ""
Output:
''
Declaring a variable as an empty string is a common practice when you want to build a string incrementally. For example, if you are constructing a message or concatenating strings, starting with an empty string allows you to append data as needed. This method is intuitive and aligns with many programming scenarios where you might not have an initial value but will be adding content later.
Moreover, an empty string can serve as a default value in functions or classes, indicating that no input has been provided yet. This can be particularly useful in user interfaces or data processing tasks where input may be optional.
Method 3: Using an Empty List
If you anticipate that your variable will eventually hold multiple values, you might want to declare it as an empty list. This method is particularly effective when you plan to collect data over time, such as user inputs or results from computations.
my_list = []
Output:
[]
By initializing a variable as an empty list, you set the stage for dynamic data storage. As you gather information, you can append items to the list using methods like append()
or extend()
. This flexibility is one of Python’s strengths, allowing for easy data manipulation.
Using an empty list also makes it clear to anyone reading your code that the variable is intended to hold a collection of items. This can improve code clarity, making it easier to understand the data flow within your program. Additionally, lists in Python are versatile, allowing you to store different data types, making them suitable for various applications.
Conclusion
Declaring a variable without a value in Python is a straightforward process that can enhance your programming practices. Whether you choose to use None
, an empty string, or an empty list, each method serves a specific purpose. Understanding these techniques not only helps you write cleaner code but also prepares you for more complex programming scenarios. Keep these methods in mind as you continue your journey in Python programming, and remember that the clarity of your code is just as important as its functionality.
FAQ
-
What is the purpose of declaring a variable without a value in Python?
Declaring a variable without a value allows you to create a placeholder, which can be assigned a value later in your code. -
Can I declare a variable without using None?
Yes, you can use an empty string or an empty list as alternatives to declare a variable without a value. -
Why is using None a good practice in Python?
Using None indicates that a variable currently holds no value, which can help prevent errors and improve code readability. -
How do I check if a variable is None?
You can use an if statement to check if a variable is None, like this:if my_variable is None:
. -
Are there any performance implications of declaring variables without values?
Generally, there are no significant performance implications, but it’s essential to manage memory wisely, especially in larger applications.