How to Check if a Variable Is None in Python
-
Use the
is
Operator to Check if a Variable IsNone
in Python -
Use the
isinstance()
Function to Check if a Variable IsNone
in Python -
Use a Dictionary to Check if a Variable Is
None
in Python -
Use the
try
andexcept
Block to Check if a Variable IsNone
in Python -
Use the Equality (
==
) Operator to Check if a Variable IsNone
in Python - Conclusion
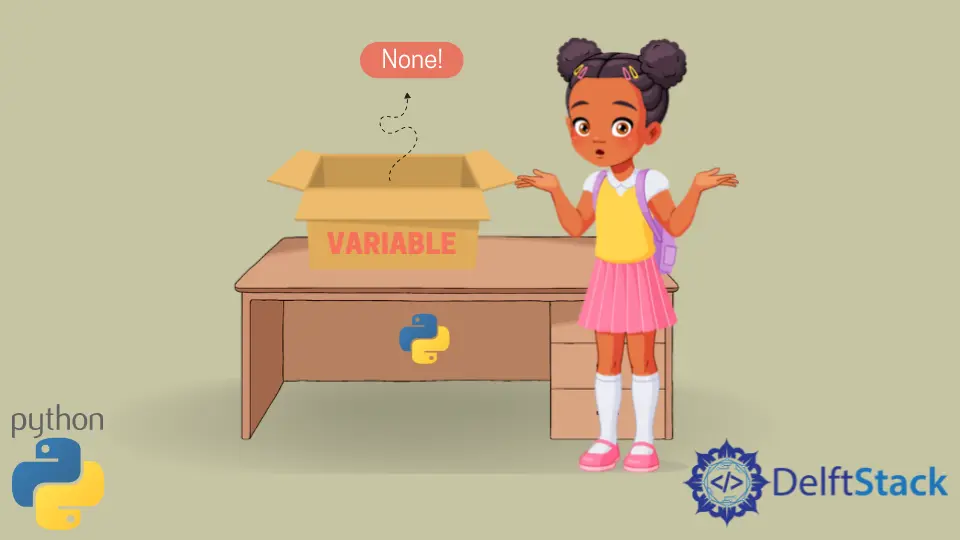
In Python, variables can store a variety of values, including integers, characters, floats, and a special value called None
. None
is not equivalent to zero; it signifies the absence of a value or a NULL value in Python.
This special value is represented by an object of type NoneType
. Occasionally, while working with variables, you may encounter situations where you need to determine whether a variable is of type None
.
In this article, we will explore various methods to check if a variable is of type None
in Python.
Use the is
Operator to Check if a Variable Is None
in Python
The is
operator in Python is used for identity comparison. It checks if two objects reference the same memory location, essentially determining if they are the same object.
When testing for None
, this operator is particularly useful because None
is a singleton object in Python. In other words, there is only one instance of None
in memory, and all variables assigned None
reference the same object.
To test if a variable is None
using the is
operator, first, declare your variable.
my_variable = None
Then, use the is
operator to check if the variable is None
.
if my_variable is None:
# Variable is None
else:
# Variable is not None
Here’s a practical example:
my_variable = None
if my_variable is None:
print("The variable is None")
else:
print("The variable is not None")
Output:
The variable is None
In this code, we assign None
to the my_variable
variable and then use the is
operator to check if it is None
.
If the variable is None
, the code in the first branch of the if
statement is executed, which prints The variable is None
. If the variable is not None
, the second branch is executed, which prints The variable is not None
.
Alternatively, you can also use the is not
operator to test if a variable is None
:
my_variable = None
if my_variable is not None:
print("The variable is not None")
else:
print("The variable is None")
Output:
The variable is None
In this code, we perform the same task as in the previous example, but this time, we use the is not
operator.
If the variable is not None
, the code in the first branch of the if
statement is executed, which prints The variable is not None
. If the variable is None
, the second branch is executed, which prints The variable is None
.
Using the is
and is not
operators to test if a variable is None
has several advantages:
- The
is
andis not
operators provide a precise check forNone
because they check for object identity, ensuring that the variable is the actualNone
object and not just a value that evaluates toFalse
or0
. - Since
None
is a singleton object, using theis
andis not
operators ensures consistent behavior across all instances in your code. - These operators are generally faster than equality (
==
) when checking forNone
because they involve a simple identity comparison.
Use the isinstance()
Function to Check if a Variable Is None
in Python
The isinstance()
function in Python is typically used to check if an object belongs to a specific class or type. However, you can also use it to test for None
. You can learn more about isinstance()
and its applications in our guide on [Python isinstance() Function](HowTo/Python/type hints in python.en.md).
This is because in Python, None
is a constant object, and you can consider it as an instance of the NoneType
class.
The isinstance()
function has the following syntax:
isinstance(object, classinfo)
Parameters:
object
: The object you want to check the type of.classinfo
: A class, type, or a tuple of classes and types. Ifobject
is an instance of any of the classes or types inclassinfo
,isinstance()
returnsTrue
.
It returns a tuple whose first element is the variable we want to check. The second element is either True
or False
, whether the variable matches the required type or not.
To check if a variable is None
using isinstance()
, first, declare your variable.
my_variable = None
Then, use the isinstance()
function to check if the variable is an instance of NoneType
.
if isinstance(my_variable, type(None)):
# Variable is None
else:
# Variable is not None
Here’s a practical example:
my_variable = None
if isinstance(my_variable, type(None)):
print("The variable is None.")
else:
print(f"The variable is not None.")
Output:
The variable is None.
In this code, we use isinstance()
to check if my_variable
is an instance of NoneType
. If it is, we print a message indicating that the variable is None
; otherwise, we print that the variable is not None
.
There are several advantages to using isinstance()
for testing None
:
- The
isinstance()
function can be used to check for various data types, not justNone
. This makes it a versatile tool in your Python toolkit. - The
isinstance()
function makes your code more explicit, allowing you to clearly express your intent to check if a variable is of a specific type (in this case,None
). - This method is consistent with the way you would check the type of an object in Python, and it leverages the language’s built-in capabilities.
Use a Dictionary to Check if a Variable Is None
in Python
Now, we’ll see an unconventional method of testing if a variable is None
using a dictionary. While this approach may not be the most common, it can be useful in specific situations where you want to create a mapping between variable names and their values.
The idea here is to use a dictionary to store variables and their corresponding values. By checking if a variable is present in the dictionary and if its value is None
, you can effectively test if the variable is None
.
This method can be particularly useful in situations where you want to manage and access variables dynamically.
Let’s begin by creating a dictionary that contains an entry for None
. We’ll associate it with a message that indicates the variable is None
.
Here’s an example:
my_variable = None
result_dict = {None: "The variable is None"}
In this dictionary, we’ve created an entry with None
as the key and the message "The variable is None"
as its corresponding value. This sets the stage for testing if my_variable
is None
.
With the dictionary entry in place, you can now use it to check if a variable is None
. Here’s how you can do it:
my_variable = None
result_dict = {None: "The variable is None"}
if my_variable in result_dict:
print(result_dict[my_variable])
else:
print("The variable is not None")
Output:
The variable is None
In this code, we use an if
statement to check if my_variable
exists as a key in the result_dict
dictionary. If it does, we print the associated message, indicating that the variable is None
.
Otherwise, we print a message stating that the variable is not None
.
Using a dictionary to test if a variable is None
might not be the most common method, but it can have its advantages:
- It allows you to manage and access variables dynamically, making it useful in scenarios where variable names are determined at runtime.
- By using a dictionary, you can keep all your variable names and values organized in one data structure, which can be helpful for debugging and data tracking.
- This method can make your code more readable, especially when dealing with multiple variables that need to be tested for
None
.
Use the try
and except
Block to Check if a Variable Is None
in Python
In Python, the try
and except
block is an indispensable construct used for exception handling. It allows you to gracefully manage errors and exceptions, and it can also be utilized to test if a variable is None
.
The try
and except
block in Python is your go-to solution for handling unexpected situations or errors. If you’re looking for more details on handling exceptions effectively, check out [Python Try Except](HowTo/Python/python exception message.en.md). It enables your program to recover from errors and continue executing, making it an essential tool for writing robust and reliable code.
When testing if a variable is None
, the try
block is used to attempt an operation that might raise an exception, while the except
block handles the exception gracefully.
Testing if a variable is None
using the try
and except
block is particularly useful in situations where you expect exceptions due to operations on a None
variable. Here’s how it works:
a = None
b = 5
try:
c = a + b
except:
print("Some variable is None")
Output:
Some variable is None
In this example, we have a variable a
set to None
and another variable b
set to 5
. We place the potentially problematic operation within the try
block, which involves adding a
and b
.
If a
is None
, this operation will raise a TypeError
due to the attempt to perform arithmetic on a None
value. The except
block then handles this exception by printing the message Some variable is None
.
Using the try
and except
block to test for None
has several advantages:
- This approach enables your program to handle exceptions gracefully. If Python encounters a variable that is
None
and attempts an operation on it, it may raise aTypeError
. Usingtry
andexcept
, you can catch and manage this error, preventing it from causing your program to crash. - The
except
block can be customized to handle specific types of exceptions, providing flexibility for dealing with different error scenarios. For instance, you can handle aTypeError
caused byNone
and separately handle other exceptions that may occur in your code. - The structure of the code makes it explicit that you are performing an operation that may raise an exception, and it provides a clear contingency plan for handling errors, making your code more readable and maintainable.
Use the Equality (==
) Operator to Check if a Variable Is None
in Python
One of the most straightforward methods to check if a variable is None
is by using the equality (==
) operator.
The equality (==
) operator in Python is used for comparison, allowing you to check if two values are equal. When it comes to testing if a variable is None
, the equality operator compares the variable to the None
singleton object.
Here’s how you can use the equality operator to test if a variable is None
:
my_variable = None
if my_variable == None:
print("The variable is None")
else:
print("The variable is not None")
Output:
The variable is None
In this code, we assign None
to the my_variable
variable and then use the equality operator to compare it to None
.
If the variable is None
, the code in the first branch of the if
statement is executed, which prints The variable is None
. If the variable is not None
, the second branch is executed, which prints The variable is not None
.
While testing for None
using the equality operator is simple and common, you should be aware of potential false positives. In Python, other falsy values like 0
, empty strings, and empty containers (e.g., an empty list) are also equal to None
when compared using the equality operator.
For example:
my_variable = 0
if my_variable == None:
print("The variable is None") # This will be executed
else:
print("The variable is not None")
Output:
The variable is not None
In the code above, the variable my_variable
is assigned the value 0
, which is a falsy value, and the equality check incorrectly indicates that it’s None
. To avoid false positives, you can use the is
operator for a more precise check.
Using the equality operator to test if a variable is None
has several advantages:
- It’s a straightforward and intuitive method. Python’s equality operator is designed for comparing values, and testing for
None
using this operator aligns with the language’s design philosophy of readability and simplicity. - This method is clear in its intent; you are explicitly checking if the variable is equal to
None
. This clarity makes your code easy to read and understand. - It’s a common approach used by Python developers, so using the equality operator for testing
None
ensures consistency in your codebase.
Conclusion
In Python, understanding how to check if a variable is of type None
is a fundamental skill. We’ve discussed several approaches to accomplish this task, each with its advantages.
You can use the is
operator for precise checks, the isinstance()
function for versatility, a dictionary for dynamic variable management, or the try
and except
blocks for error handling. Alternatively, you can use the equality (==
) operator, although it may lead to false positives with other falsy values.
Choosing the right method depends on your specific use case and coding preferences. Regardless of the method you choose, the ability to identify and handle None
values is a valuable skill in Python programming.