Ceiling Division in Python
- Understanding Ceiling Division
-
Method 1: Using the
math.ceil()
Function - Method 2: Using Integer Division and Conditional Logic
- Method 3: Using NumPy for Ceiling Division
- Conclusion
- FAQ
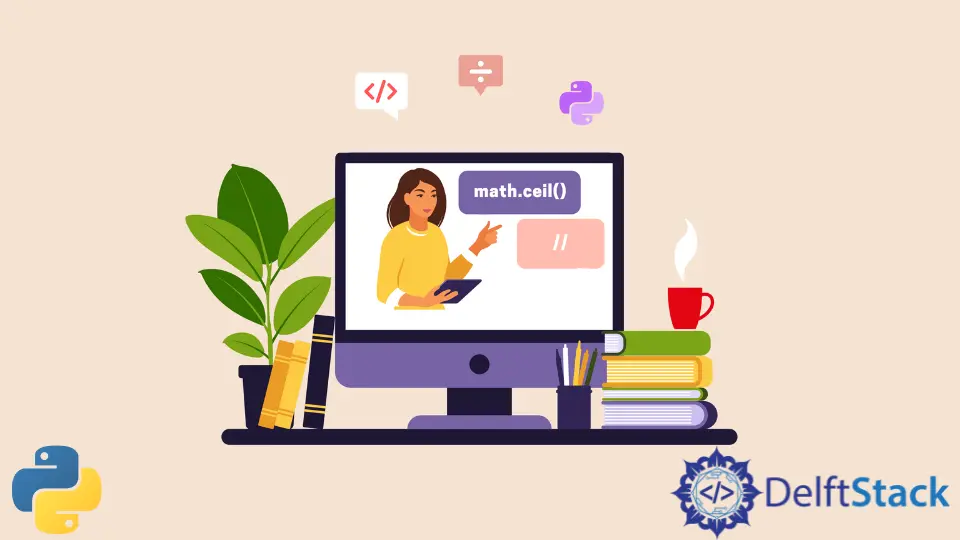
Ceiling division is a handy mathematical operation that rounds up the result of a division to the nearest whole number. In Python, this operation can be particularly useful when dealing with scenarios where you need to ensure that you have enough resources or space, such as dividing items into groups or calculating pages in pagination.
In this article, we’ll explore various methods to perform ceiling division in Python, providing clear examples and explanations to help you understand how to implement this functionality in your projects. Whether you’re a beginner or an experienced developer, this guide will equip you with the knowledge you need to master ceiling division in Python.
Understanding Ceiling Division
Ceiling division is the process of dividing two numbers and rounding up the result to the nearest integer. For instance, if you divide 5 by 2, the result is 2.5. With ceiling division, you would round this up to 3. This operation is particularly useful in scenarios where you cannot have fractional results, such as when calculating the number of containers needed to hold a certain number of items.
In Python, there are several ways to perform ceiling division. The most common methods include using the math.ceil()
function, the //
operator combined with conditional logic, and leveraging the numpy
library. Let’s delve into each method to see how they work.
Method 1: Using the math.ceil()
Function
The math
module in Python provides a built-in function called ceil()
that can be used to perform ceiling division. This method is straightforward and easy to understand. Here’s how you can implement it:
import math
def ceiling_division(a, b):
return math.ceil(a / b)
result = ceiling_division(5, 2)
print(result)
Output:
3
In this example, we first import the math
module, which contains the ceil()
function. The ceiling_division
function takes two parameters, a
and b
, divides a
by b
, and then applies math.ceil()
to round the result up to the nearest integer. When we call the function with 5 and 2, it returns 3, as expected.
Using math.ceil()
is a clean and efficient way to achieve ceiling division. It clearly communicates the intent of rounding up, making your code more readable. This method is especially useful when you want to ensure that your results are always whole numbers, such as when calculating the number of pages needed for a document or the number of items per container.
Method 2: Using Integer Division and Conditional Logic
Another way to perform ceiling division in Python is by combining integer division with a simple conditional check. This method is a bit more manual but can be useful in scenarios where you want to avoid importing additional libraries. Here’s how it works:
def ceiling_division(a, b):
return (a + b - 1) // b
result = ceiling_division(5, 2)
print(result)
Output:
3
In this code snippet, we define the ceiling_division
function that takes two arguments, a
and b
. Instead of using the math.ceil()
function, we adjust a
by adding b - 1
before performing integer division with the //
operator. This adjustment effectively rounds up the result of the division if there is any remainder.
For example, when we call ceiling_division(5, 2)
, the calculation becomes (5 + 2 - 1) // 2
, which simplifies to 6 // 2
, resulting in 3. This method is particularly useful when you want a lightweight solution without relying on external libraries. It’s efficient and performs well in scenarios where you’re handling large datasets or need to optimize for speed.
Method 3: Using NumPy for Ceiling Division
If you’re working with arrays or large datasets, the numpy
library provides a powerful way to perform ceiling division efficiently. NumPy is optimized for numerical operations and can handle large arrays with ease. Here’s how to use it for ceiling division:
import numpy as np
def ceiling_division(a, b):
return np.ceil(a / b).astype(int)
result = ceiling_division(5, 2)
print(result)
Output:
3
In this example, we import the NumPy library and define the ceiling_division
function. The function divides a
by b
, applies np.ceil()
to round up the result, and then converts it to an integer using .astype(int)
. This is particularly useful when working with arrays, as NumPy can handle element-wise operations efficiently.
When you call ceiling_division(5, 2)
, it performs the division, rounds up, and converts the result to an integer, giving you 3. This method is ideal for data analysis tasks where you may need to perform ceiling division on multiple values at once, making it a versatile tool in your programming toolkit.
Conclusion
Ceiling division is a crucial operation in Python that can simplify many tasks, from resource allocation to data manipulation. Whether you choose to use the math.ceil()
function, a combination of integer division and conditional logic, or the powerful NumPy library, understanding how to implement ceiling division will enhance your programming skills. Each method has its own advantages, and the choice depends on your specific use case and the complexity of your project. By mastering ceiling division, you can ensure that your calculations are precise and effective.
FAQ
-
What is ceiling division?
Ceiling division is the mathematical operation that divides two numbers and rounds up the result to the nearest whole number. -
How do I perform ceiling division in Python?
You can use themath.ceil()
function, integer division with conditional logic, or the NumPy library to perform ceiling division in Python. -
Why would I use ceiling division?
Ceiling division is useful in scenarios where fractional results are not acceptable, such as when calculating the number of containers needed for items or pages in a document. -
Is there a performance difference between methods?
Yes, using NumPy can be more efficient for large datasets, while the other methods are simpler for smaller calculations. -
Can I use ceiling division with negative numbers?
Yes, ceiling division can be applied to negative numbers, but the results may not align with typical expectations due to the nature of rounding.