How to Fix the TypeError: Can Only Join an Iterable in Python
-
What Is the
TypeError: can only join an iterable
in Python -
Use
join()
With Iterable Data Types to Fix theTypeError: can only join an iterable
in Python - Check the Data Type Before Joining
- Convert Non-Iterable Objects to Iterable
- Handle Edge Cases and Empty Data
- Debugging and Error Handling
- Conclusion
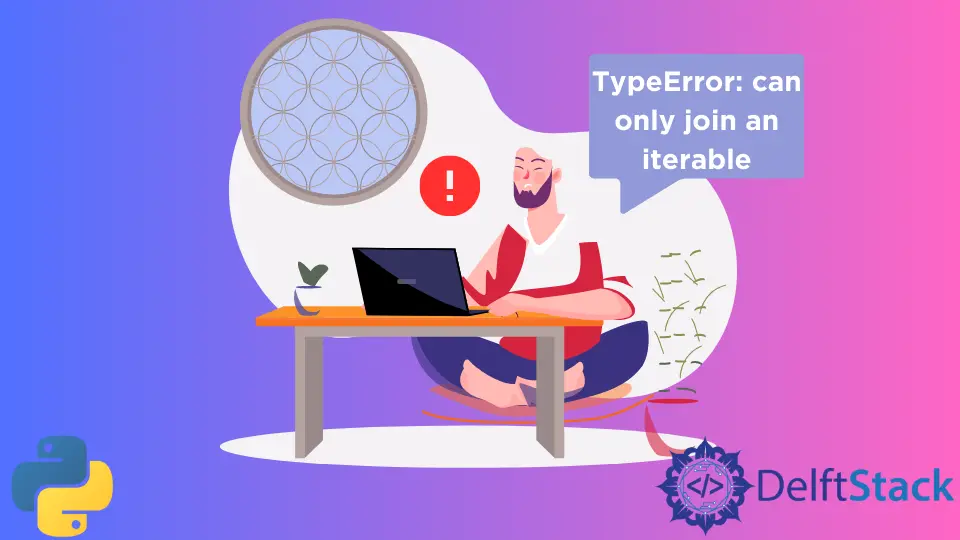
Strings, lists, tuples, and other similar objects are often referred to as iterables in Python. This is because they contain a finite number of elements that can be referred to using their indexes; we can loop over these objects using a simple for
loop.
A handy function that works with such iterables is the join()
function. This function can combine the elements of an iterable into a single string, and we can specify the separator of the elements in the string with the function.
This tutorial will discuss the TypeError: can only join an iterable
error in Python.
What Is the TypeError: can only join an iterable
in Python
Python’s TypeError: can only join an iterable
is a common error encountered when attempting to concatenate strings using the str.join()
method with a non-iterable object.
This error signifies that the join()
method expects an iterable (such as a list, tuple, or string) as its argument but receives a different type. Here, we’ll explore various methods to address and resolve this issue.
Since it is a TypeError
, we can conclude that an unsupported operation is being performed on a given object. This error is encountered when we try to combine the elements of an unsupported type of object using the join()
function.
Example:
a = 456
s = "".join(a)
print(s)
This code attempts to use the join()
method on an integer (a = 456
). However, the join()
method expects an iterable object (like a list, tuple, or string) as its argument.
Since integers are not iterable, this leads to a TypeError: can only join an iterable
.
Output:
TypeError: can only join an iterable
In the above example, we tried to use the join()
function with an integer and got this error.
Use join()
With Iterable Data Types to Fix the TypeError: can only join an iterable
in Python
In Python, the join()
method is used to concatenate elements within an iterable, such as a list, tuple, or string, using a specified separator. Its syntax is as follows:
string_separator.join(iterable)
Here’s a breakdown of the parameters:
string_separator
: This parameter specifies the separator that will be used to join the elements in the iterable. It could be an empty string (""
), a single character, or a substring.iterable
: The iterable object whose elements will be concatenated together. Common iterables include strings, lists, tuples, etc.
The fix to this error is simple: only use the valid data types that are iterable. A very simple example of the join()
function with an iterable is shown below.
a = ["4", "5", "6"]
s = "".join(a)
print(s)
Output:
456
In this scenario, since a
contains strings within the list, the join()
method concatenates these strings together without any separator, resulting in the string '456'
. Thus, when print(s)
is executed, it will display 456
.
Remember that since the join()
function returns a string, the elements of an iterable must also be a string; otherwise, a new error will be raised.
There are some cases where we get the can only join an iterable
error while working with iterables.
For example:
a = ["4", "5", "6"]
b = a.reverse()
s = "".join(b)
print(s)
Output:
TypeError: can only join an iterable
The above example raises the error because the reverse()
function reverses the order of elements in the original list. It does not create a new list.
So, in the above example, the object b
value is None
. That is why the error is raised.
In such cases, be mindful of the final object passed onto the join()
function. We can fix the above example using the reversed()
method, which returns a new list.
See the code below:
a = ["4", "5", "6"]
b = reversed(a)
s = "".join(b)
print(s)
Output:
654
Since the reversed()
method returns a new list, the error was avoided in the above example.
Check the Data Type Before Joining
When working with Python and joining data, it’s important to check the data types involved before attempting to use the join()
operation. This is done to prevent encountering the TypeError: can only join an iterable
.
By checking that the data types are suitable for joining (such as ensuring both are iterable), you can avoid encountering errors and ensure smooth execution of the join()
method in your Python code.
Thus, it is advised that we ensure that the object passed to the join()
method is iterable. If not, convert it to an appropriate iterable type.
Example:
# Checking data type before joining
my_string = "Hello"
if isinstance(my_string, str):
result = " ".join([my_string])
print(result)
else:
print("Object is not iterable")
The code checks if a variable, my_string
, is a string type using isinstance()
. If my_string
is a string, it uses the join()
method to concatenate it with a space as a separator.
The joined string is then printed. If the variable is not a string, it prints a message indicating that the object is not iterable.
Output:
Hello
This helps prevent errors when attempting to join non-iterable objects.
Convert Non-Iterable Objects to Iterable
For non-iterable objects, we need to convert them to an iterable type like a list or string to enable concatenation using join()
. To convert non-iterable objects to iterable ones in Python, you can use various techniques depending on the specific type of object.
Example:
# Converting non-iterable objects to iterable
my_integer = 123
if isinstance(my_integer, int):
result = "".join(str(my_integer))
print(result)
Output:
123
In this code, the integer 123
is converted to a string and then immediately joined. Although, in this case, joining a single string with an empty separator doesn’t change the string, this illustrates the process of converting a non-iterable object (like an integer) into an iterable object (a string, in this case) to make it compatible with the join()
method.
Handle Edge Cases and Empty Data
Handling edge cases and empty data is a practice in programming that involves incorporating specific code to address scenarios where unexpected or atypical conditions might occur. This is particularly relevant when dealing with iterable objects and joining operations in Python to avoid encountering errors like can only join an iterable
.
Therefore, it is advised that we ensure the object passed to join()
isn’t empty or contains invalid data, as it can cause the TypeError
.
Example:
# Handling edge cases and empty data
my_empty_list = []
if my_empty_list:
result = ",".join(my_empty_list)
print(result)
else:
print("List is empty")
Output:
List is empty
Since my_empty_list
is an empty list, the join()
method is not called on it within the if
block. Instead, the code proceeds to the else
block and prints the message indicating that the list is empty.
This code snippet demonstrates handling empty data or edge cases by checking whether a list is empty before performing operations like joining its elements. If the list is empty, it provides feedback or executes alternate instructions to handle the empty scenario.
Debugging and Error Handling
Debugging and error handling are crucial aspects of writing robust code in Python. To avoid the can only join an iterable
error when working with iterable operations, you can incorporate debugging techniques and implement proper error-handling mechanisms.
One way to do this is by implementing the try-except
blocks to catch errors and debug issues with the join()
method.
Example:
# Debugging with try-except block
my_dict = {"key": "value"}
try:
result = ",".join(my_dict)
print(result)
except TypeError as e:
print(f"Error: {e}")
Output:
key
In this code, the try-except
block is utilized for error handling. It attempts to execute the join()
method on a dictionary, knowing that it might raise a TypeError
.
If a TypeError
occurs during this operation, it’s caught in the except
block, displaying an error message specifying the nature of the error (TypeError
) and the error details.
Conclusion
The TypeError: can only join an iterable
error in Python arises when attempting to use the join()
method with non-iterable objects. By ensuring the correct usage of join()
with appropriate data types and handling edge cases, this error can be effectively resolved.
Debugging and error handling practices further aid in identifying and rectifying issues related to the join()
method.
Remember, understanding the nature of data being passed to join()
and verifying its iterability are key steps to avoid encountering this error in your Python programs.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedInRelated Article - Python Error
- Can Only Concatenate List (Not Int) to List in Python
- How to Fix Value Error Need More Than One Value to Unpack in Python
- How to Fix ValueError Arrays Must All Be the Same Length in Python
- Invalid Syntax in Python
- How to Fix the TypeError: Object of Type 'Int64' Is Not JSON Serializable
- How to Fix the TypeError: 'float' Object Cannot Be Interpreted as an Integer in Python