How to Fix the SyntaxError: 'break' Outside Loop Error in Python
- Understanding the SyntaxError: ‘break’ Outside Loop
- Correct Placement of the Break Statement
- Using Flags Instead of Break
- Refactoring Code for Clarity
- Conclusion
- FAQ
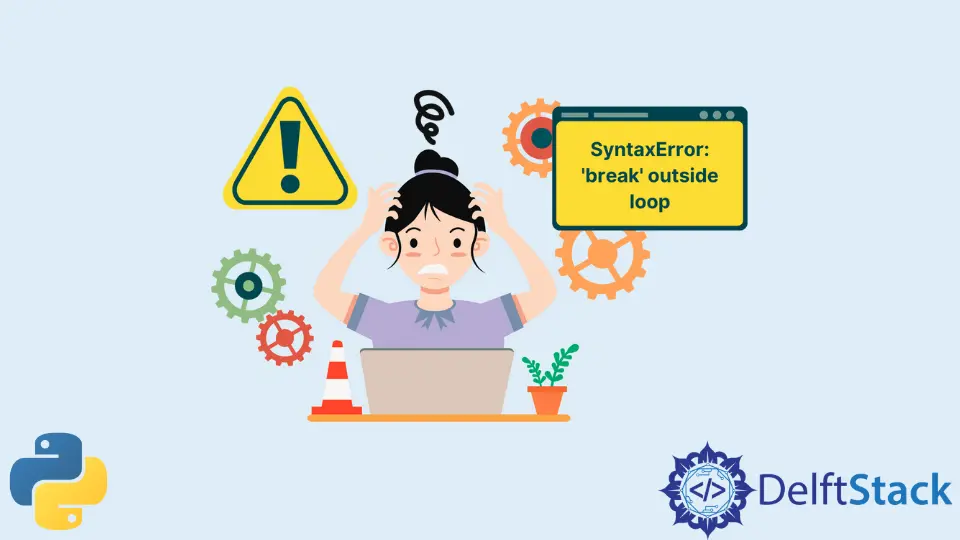
When you’re coding in Python, encountering errors can be frustrating, especially when they seem cryptic. One such common error is the SyntaxError: ‘break’ Outside Loop. This error usually arises when you mistakenly use the break statement outside of a loop. The break statement is designed to exit a loop prematurely, but if it’s placed incorrectly, Python raises this error, halting your program.
In this tutorial, we will explore how to fix this error effectively, providing clear examples and explanations. Whether you’re a beginner or a seasoned programmer, understanding how to resolve this issue is crucial for writing clean and functional code. Let’s dive into the solutions!
Understanding the SyntaxError: ‘break’ Outside Loop
Before we jump into fixing the error, it’s essential to understand why it occurs. The break statement is meant to be used within loops—either for or while
loops. When Python encounters a break statement outside of any loop, it doesn’t know what to do with it, hence the SyntaxError.
Consider the following example:
if condition:
break
In this scenario, the break statement is incorrectly placed inside an if statement instead of a loop. To resolve this error, you need to ensure that the break statement is nested within an appropriate loop structure.
Correct Placement of the Break Statement
One of the most straightforward ways to fix the SyntaxError is to ensure that your break statement is properly placed within a loop. Here’s how you can do it.
for i in range(5):
if i == 3:
break
print(i)
Output:
0
1
2
In this example, we have a for loop that iterates through numbers 0 to 4. The break statement is used to exit the loop when the value of i
equals 3. As a result, the loop prints 0, 1, and 2 before breaking. This placement of the break statement within the loop avoids the SyntaxError and allows the program to run smoothly.
To avoid the SyntaxError, always double-check that your break statements are nested inside loops. If you find one outside, consider restructuring your code.
Using Flags Instead of Break
Sometimes, using a flag variable can be an effective alternative to the break statement. Instead of prematurely exiting a loop, you can set a flag to indicate when to stop further processing. Here’s an example of how to implement this approach.
stop_loop = False
for i in range(5):
if stop_loop:
break
if i == 3:
stop_loop = True
print(i)
Output:
0
1
2
In this example, we introduced a flag variable called stop_loop. When i
equals 3, we set stop_loop to True, which causes the loop to break in the next iteration. This approach allows you to maintain clarity in your code while avoiding the SyntaxError.
Using flags can be particularly useful in more complex scenarios where multiple conditions might determine when to exit a loop. It also makes your intentions clearer to anyone reading your code.
Refactoring Code for Clarity
Another effective method to fix the SyntaxError is to refactor your code for better clarity and structure. Sometimes, the placement of the break statement might be a symptom of a larger structural issue in your code. By refactoring, you can create a more logical flow that naturally avoids such errors.
Consider the following code that leads to the error:
if condition:
break
for i in range(5):
print(i)
To refactor this, you could restructure it as follows:
for i in range(5):
if condition:
break
print(i)
Output:
0
1
2
In this refactored version, the break statement is now correctly placed within the loop. This not only resolves the SyntaxError but also improves the overall readability of your code. Refactoring can help you spot logical errors and ensure that your code is maintainable in the long run.
Conclusion
The SyntaxError: ‘break’ Outside Loop can be a common stumbling block for Python programmers. However, by understanding the correct placement of the break statement, using flags as alternatives, and refactoring your code for clarity, you can easily resolve this issue. Remember, coding is as much about structure and readability as it is about functionality. By following these strategies, you’ll not only fix errors but also improve your coding skills over time. Happy coding!
FAQ
-
What does the SyntaxError: ‘break’ Outside Loop mean?
This error indicates that a break statement is used outside of a loop, which is not allowed in Python. -
How can I avoid the ‘break’ Outside Loop error?
Ensure that your break statements are placed inside loops, such as for orwhile
loops. -
Can I use a flag variable instead of a break statement?
Yes, using a flag variable can help indicate when to stop processing without using break. -
What are some best practices for using loops in Python?
Always maintain clarity in your loop structures, avoid deep nesting, and ensure that your break statements are properly placed. -
Is refactoring my code necessary to fix this error?
While not always necessary, refactoring can improve your code’s clarity and help avoid logical errors, including the break statement issue.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedInRelated Article - Python Error
- Can Only Concatenate List (Not Int) to List in Python
- How to Fix Value Error Need More Than One Value to Unpack in Python
- How to Fix ValueError Arrays Must All Be the Same Length in Python
- Invalid Syntax in Python
- How to Fix the TypeError: Object of Type 'Int64' Is Not JSON Serializable
- How to Fix the TypeError: 'float' Object Cannot Be Interpreted as an Integer in Python