How to Fix AttributeError: Module Urllib Has No Attribute Request
-
the
AttributeError: module 'urllib' has no attribute 'request'
in Python -
Fix the
AttributeError: module 'urllib' has no attribute 'request'
in Python
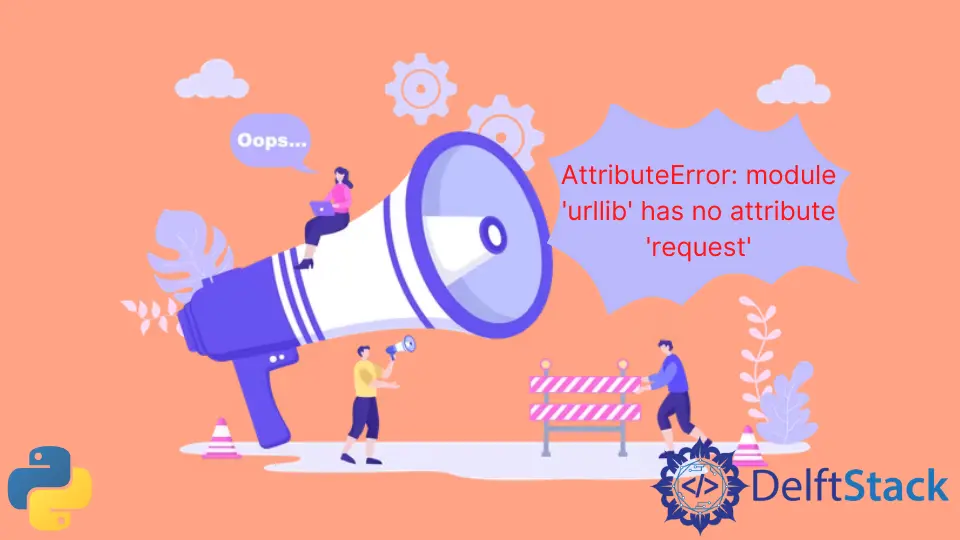
Since urllib.request
in Python is an independent module, urllib
cannot be implemented in this situation. If a module has several submodules, individually loading each could be expensive and lead to issues with items unrelated to your program.
Python will cache the import since the module you’re using imports to use for itself, making it a part of the object.
the AttributeError: module 'urllib' has no attribute 'request'
in Python
This error is a common exception in Python when you try to open URL links by importing URL libraries. To understand this error, let’s take an example.
Code example:
import urllib
request = urllib.request.Request("http://www.python.org")
Output:
AttributeError: module 'urllib' has no attribute 'request'
You may occasionally import the module you require when using packages like this. The urllib
module won’t have to load everything just because you just needed a small part of any url
.
The import url
is accessing the whole library; that’s why it’s showing an error.
Fix the AttributeError: module 'urllib' has no attribute 'request'
in Python
You can import like import urllib.request as urllib
or the other way around to use import urllib.request urllib.request.urlopen('http://www.python.org',timeout=1)
.
import urllib.request
with urllib.request.urlopen("http://www.python.org") as url:
s = url.read()
print(s)
Output:
<!doctype html>
<head>
<meta charset="utf-8">
'
'
'
'
from urllib.request import Request, urlopen
def get_page_content(url, head):
req = Request(url, headers=head)
return urlopen(req)
url = "https://example.com"
head = {
"User-Agent": "Mozilla/5.0 (Macintosh; Intel Mac OS X 10_14_6) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/99.0.4844.84 Safari/537.36",
"Accept": "text/html,application/xhtml+xml,application/xml;q=0.9,image/avif,image/webp,image/apng,*/*;q=0.8,application/signed-exchange;v=b3;q=0.9",
"Accept-Charset": "ISO-8859-1,utf-8;q=0.7,*;q=0.3",
"Accept-Encoding": "none",
"Accept-Language": "en-US,en;q=0.8",
"Connection": "keep-alive",
"refere": "https://example.com",
"cookie": """your cookie value ( you can get that from your web page) """,
}
data = get_page_content(url, head).read()
print(data)
Output:
<!doctype html>\n<html>\n<head>\n <title>Example Domain</title>\n\n <meta charset="utf-8" />\n
'
'
'
href="https://www.iana.org/domains/example">More information...</a></p>\n</div>\n</body>\n</html>\n'
Instead of fully importing the urllib
module and then attempting to access its submodule request as an attribute, you should import the submodule request from the parent module urllib
.
The import urllib print(urllib.request)
doesn’t work, but from urllib import request print(request)
and import urllib.request
both succeed.
Zeeshan is a detail oriented software engineer that helps companies and individuals make their lives and easier with software solutions.
LinkedInRelated Article - Python Error
- Can Only Concatenate List (Not Int) to List in Python
- How to Fix Value Error Need More Than One Value to Unpack in Python
- How to Fix ValueError Arrays Must All Be the Same Length in Python
- Invalid Syntax in Python
- How to Fix the TypeError: Object of Type 'Int64' Is Not JSON Serializable
- How to Fix the TypeError: 'float' Object Cannot Be Interpreted as an Integer in Python