How to Append Multiple Elements to List in Python
-
Append a Single Element in the Python List Using the
append()
Function -
Use the
extend()
Function to Append Multiple Elements in the Python List - Use List Slicing to Append Multiple Elements in the Python List
- Use the Concatenation Method to Append Multiple Elements in the Python List
-
Use the
itertools.chain
Function to Append Multiple Elements in the Python List - Conclusion
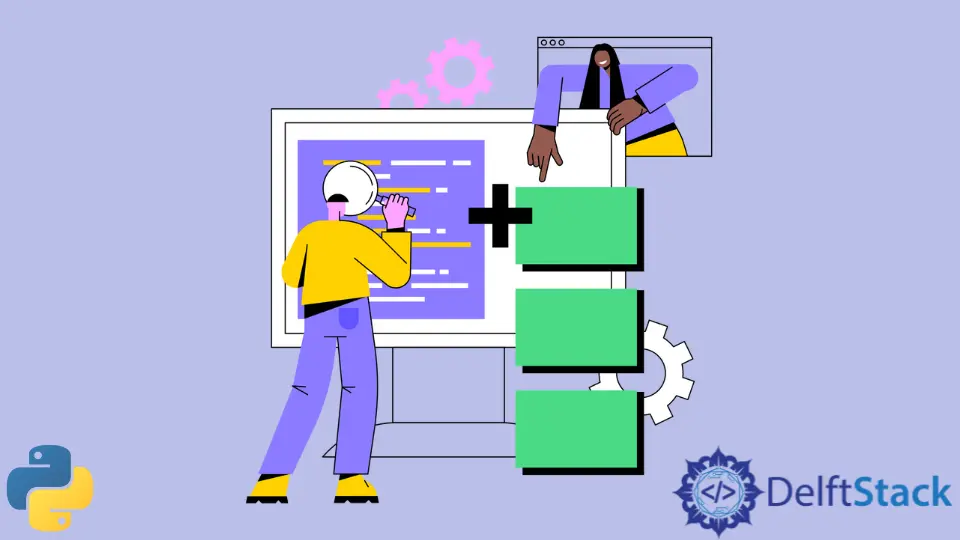
The list is a mutable data structure in Python. It could contain different types of values. Appending elements to a list is a common operation in Python.
While it’s straightforward and relatively quite easy to append just one element using the append()
method, there may be situations where you need to append several elements to a list efficiently.
In this tutorial, we will explore various approaches to append multiple elements to a list in Python, starting with an introduction to the append()
function to append a single element.
Append a Single Element in the Python List Using the append()
Function
Lists are sequences that can hold different data types and Python objects, and you can use the append()
method, which can be utilized to add a single value to the end of the list.
Consider the following example.
lst = [2, 4, 6, "python"]
lst.append(6)
print("The appended list is:", lst)
Output:
Similarly, to add one more new value, we will use another append()
method to add another new value after the value 6
in the list.
lst = [2, 4, 6, "python"]
lst.append(6)
lst.append(7)
print("The appended list is:", lst)
Output:
Use the extend()
Function to Append Multiple Elements in the Python List
The extend()
method will extend the list by adding all items to the iterable. We use the same example list created in the above code and add the new list elements.
The complete example code is given below.
lst = [2, 4, 6, "python"]
lst.extend([8, 9, 10])
print("The appended list is:", lst)
Output:
The extend()
function in this code adds all the elements mentioned in a new list created and uses the respective function at the end of the given list lst
.
Use List Slicing to Append Multiple Elements in the Python List
List slicing is originally utilized to manipulate a list to solve efficient problems. It can be done by specifying the start
, stop
, and step
values.
However, list slicing can also be used to append multiple elements in the Python list, provided the elements we add are part of another list.
The complete example code is given below.
lst = ["welcome", ".com"]
lst1 = ["to", "delftstack"]
lst[1:1] = lst1
print(lst)
Output:
The above code creates two lists, then uses list slicing on the first list and a simple assignment operation to add the items of the second list to the first.
Use the Concatenation Method to Append Multiple Elements in the Python List
In many cases, the +
symbol is used for concatenation and can combine the elements of two or more lists.
The complete example code is given below.
lst1 = [2, 4, 6, 8]
lst2 = ["python", "java"]
lst3 = lst1 + lst2
print("The Concatenated List is:", lst3)
Output:
The above code defines two lists and uses the concatenation(+
) operator to combine all the elements between them into a third list.
Note: We can make use of the concatenation operator along with list slicing as well.
Use the itertools.chain
Function to Append Multiple Elements in the Python List
The chain()
function is imported from the itertools
. The purpose of the chain
function is the same as the concatenation operator +
.
It will combine all the list’s elements into a new list. The performance of this method is much more efficient than other methods.
The complete example code is given below.
from itertools import chain
lst1 = [2, 4, 6, 8]
lst2 = ["python", "java"]
final_list = list(chain(lst1, lst2))
print("The Final List is:", final_list)
Output:
As the chain()
function must be imported from the itertools
library, we first import it. Then, the chain()
function takes up both lists as its arguments to create a third list that combines all the elements of the first two lists.
Conclusion
We can append multiple elements to a Python list with the help of various methods, including the extend()
method, the +
operator, list slicing, and the chain()
function from the itertools
library. Choosing an appropriate way depends on a particular programmer’s coding style and requirements.
Consider the complexity and performance implications that might be there in the background while appending more than a few elements in a Python list.
Related Article - Python List
- How to Convert a Dictionary to a List in Python
- How to Remove All the Occurrences of an Element From a List in Python
- How to Remove Duplicates From List in Python
- How to Get the Average of a List in Python
- What Is the Difference Between List Methods Append and Extend
- How to Convert a List to String in Python