用 Python 將多個元素新增到列表中
Azaz Farooq
2023年1月30日
Python
Python List
-
使用
append()
函式在 Python 列表中追加單個元素 -
使用
extend()
函式在 Python 列表中新增多個元素 - 在 Python 列表中使用連線方法追加多個元素
-
使用
itertools.chain
函式在 Python 列表中新增多個元素
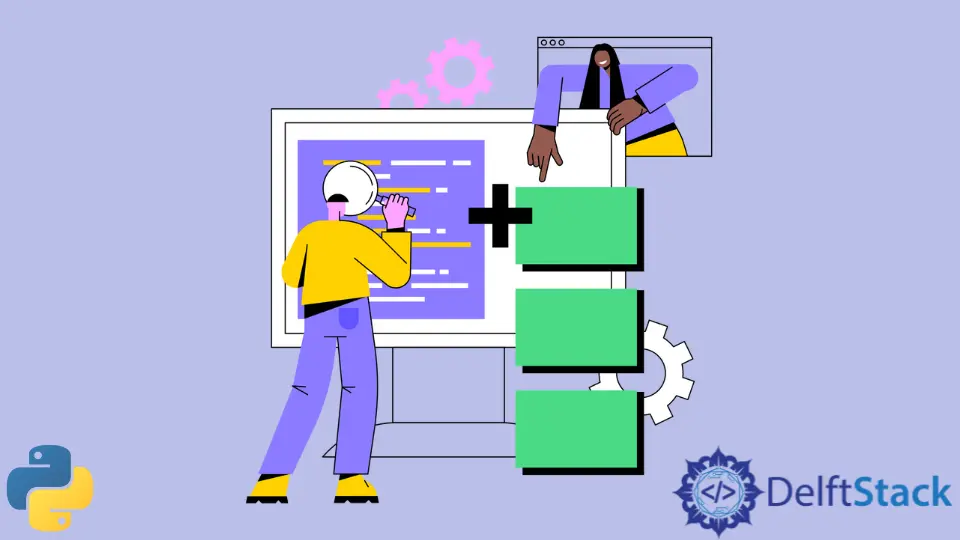
列表是 Python 中的一個可變資料結構。它可以包含不同型別的值。
本文將討論一些在 Python 列表中追加單個或多個元素的方法。
使用 append()
函式在 Python 列表中追加單個元素
append()
方法將單個值新增到列表的末尾。
完整的示例程式碼如下:
lst = [2, 4, 6, "python"]
lst.append(6)
print("The appended list is:", lst)
輸出:
The appended list is: [2, 4, 6, 'python', 6]
同樣,為了增加一個新的值,我們將使用另一個 append()
方法在列表中的值 6
之後增加一個新的值。
lst = [2, 4, 6, "python"]
lst.append(6)
lst.append(7)
print("The appended list is:", lst)
輸出:
The appended list is: [2, 4, 6, 'python', 6, 7]
使用 extend()
函式在 Python 列表中新增多個元素
這個方法將通過將所有專案新增到可迭代列表中來擴充套件列表。我們使用上述程式碼中建立的附加列表,並將新的列表元素新增到其中。
完整的示例程式碼如下:
lst = [2, 4, 6, "python"]
lst.extend([8, 9, 10])
print("The appended list is:", lst)
輸出:
The appended list is: [2, 4, 6, 'python', 8, 9, 10]
在 Python 列表中使用連線方法追加多個元素
+
符號用於連線和合並兩個列表。完整的示例程式碼在下面給出。
lst1 = [2, 4, 6, 8]
lst2 = ["python", "java"]
lst3 = lst1 + lst2
print("The Concatenated List is:", lst3)
輸出:
The Concatenated List is: [2, 4, 6, 8, 'python', 'java']
使用 itertools.chain
函式在 Python 列表中新增多個元素
chain()
函式是從 itertools
中匯入的。chain
函式的目的與連線操作符+
相同。它將把所有列表的元素合併成一個新的列表。這種方法的效能比其他方法高效得多。
完整的示例程式碼如下:
from itertools import chain
lst1 = [2, 4, 6, 8]
lst2 = ["python", "java"]
final_list = list(chain(lst1, lst2))
print("The Final List is:", final_list)
輸出:
The Final List is: [2, 4, 6, 8, 'python', 'java']
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe