How to Append 2D Array in Python
- Using Nested Lists (Python Lists)
- Using List Comprehension
- Using NumPy Arrays
-
Using
numpy.hstack()
andnumpy.vstack()
-
Using
+=
Operator -
Using
extend()
to Append Multiple Rows -
Using
insert()
to Add a Row at a Specific Index - Using Nested Loops for Dynamic 2D Array Population
- Conclusion
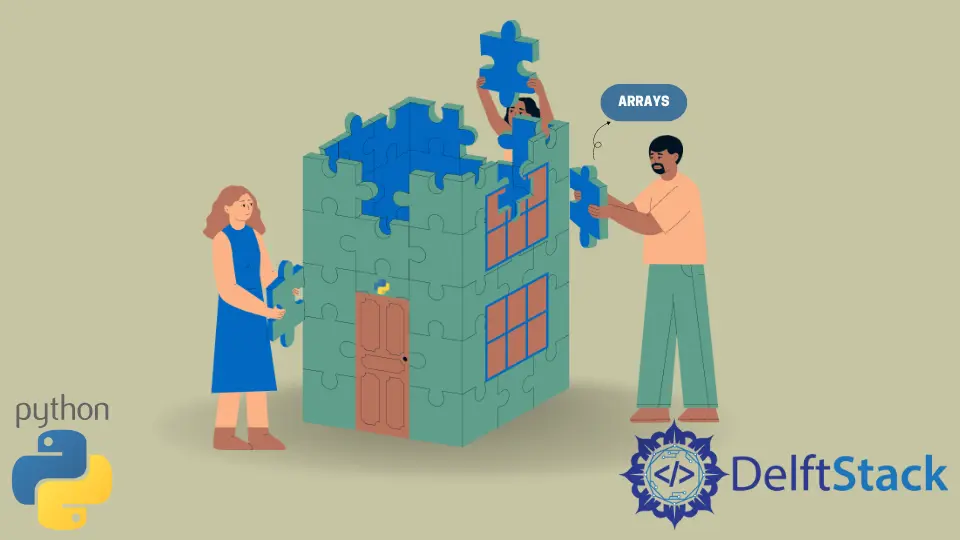
Appending values to a 2D array in Python is a common operation in data manipulation, numerical computing, and data science applications. Whether you are working with native Python lists or using NumPy for efficiency, there are multiple methods to append rows, columns, or individual values to a 2D array. This guide explores different techniques, their advantages, and use cases.
Using Nested Lists (Python Lists)
Python’s built-in lists can represent 2D arrays as lists of lists. The simplest way to append values is using the append()
method.
Appending a New Row
array = [[1, 2, 3], [4, 5, 6]]
array.append([7, 8, 9])
print(array)
Output:
[[1, 2, 3],
[4, 5, 6],
[7, 8, 9]]
This method adds an entirely new row to the existing list. The append()
method is useful when you want to expand a 2D list dynamically by adding more data over time. However, note that all rows may need to have the same length for structured data processing.
Appending a Value to an Existing Row
array[0].append(10)
print(array)
Output:
[[1, 2, 3, 10],
[4, 5, 6],
[7, 8, 9]]
This method modifies a specific row by appending a new element. Unlike adding a new row, this operation affects only the specified row, which can lead to an irregular column structure if not managed properly.
Using List Comprehension
List comprehensions provide an elegant way to append values across all rows in a 2D list.
array = [[1, 2], [3, 4]]
array = [row + [5] for row in array]
print(array)
Output:
[[1, 2, 5],
[3, 4, 5]]
This method efficiently adds a new column to each row. By iterating over each row and appending a value, list comprehension ensures that the new column is uniformly added across all rows.
Using NumPy Arrays
NumPy provides powerful methods for handling and appending values to 2D arrays efficiently. However, when dealing with large datasets with many empty values, using a sparse matrix can significantly reduce memory consumption. Learn more about [sparse matrices in Python]({{relref “/HowTo/Python/sparse matrix in python.en.md”}}) and how they optimize storage.
Using numpy.append()
import numpy as np
array = np.array([[1, 2], [3, 4]])
array = np.append(array, [[5, 6]], axis=0)
print(array)
Output:
[[1 2]
[3 4]
[5 6]]
This method appends a new row to a NumPy array. The numpy.append()
function is useful when dealing with large datasets, as it ensures efficient memory management compared to native Python lists.
To append a new column:
array = np.append(array, [[7], [8], [9]], axis=1)
print(array)
Output:
[[1 2 7]
[3 4 8]
[5 6 9]]
After appending values, you possibly need to save the modified array to a file for later use. You can refer to our guide on [writing arrays to a text file]({{relref “/HowTo/Python/write array to a textfile.en.md”}}) for details on storing NumPy arrays efficiently.
If you encounter indexing errors while modifying NumPy arrays, refer to our guide on fixing ‘Too Many Indices for Array’ errors to troubleshoot common mistakes.
Using numpy.hstack()
and numpy.vstack()
NumPy’s hstack()
and vstack()
functions are designed for appending columns and rows efficiently.
Using hstack()
to Append a Column
import numpy as np
array = np.array([[1, 2], [3, 4]])
new_column = np.array([[5], [6]])
result = np.hstack((array, new_column))
print(result)
Output:
[[1 2 5]
[3 4 6]]
Using vstack()
to Append a Row
new_row = np.array([[5, 6]])
result = np.vstack((array, new_row))
print(result)
Output:
[[1 2]
[3 4]
[5 6]]
These methods are efficient and recommended when working with large datasets. Unlike numpy.append()
, which may require restructuring the entire array, hstack()
and vstack()
are optimized for column-wise and row-wise extensions, respectively.
If you’re working with structured numerical data, you might also need to transpose matrices for reshaping data efficiently. Check out our article on transposing matrices in Python.
Using +=
Operator
array = [[1, 2], [3, 4]]
array += [[5, 6]]
print(array)
Output:
[[1, 2],
[3, 4],
[5, 6]]
This method is a shorthand for append()
but modifies the list in place. Using +=
ensures that new rows are directly added without creating a new reference, making it an efficient approach for handling small 2D lists.
Using extend()
to Append Multiple Rows
array = [[1, 2], [3, 4]]
array.extend([[5, 6], [7, 8]])
print(array)
Output:
[[1, 2],
[3, 4],
[5, 6],
[7, 8]]
This is useful when adding multiple rows at once. The extend()
method is particularly helpful when merging data from different sources into a single 2D list.
If you need to store the updated list as a CSV file, you can check our guide on writing lists to a CSV file in Python.
Using insert()
to Add a Row at a Specific Index
array = [[1, 2], [3, 4]]
array.insert(1, [5, 6])
print(array)
Output:
[[1, 2],
[5, 6],
[3, 4]]
The insert()
method allows precise row placement within the array. This is useful when working with ordered datasets where new entries need to be inserted at specific positions without disrupting the overall structure.
Using Nested Loops for Dynamic 2D Array Population
array = []
for i in range(3):
row = []
for j in range(2):
row.append(i * j)
array.append(row)
print(array)
Output:
[[0, 0],
[0, 1],
[0, 2]]
This method is useful for dynamically generating structured 2D arrays. Using nested loops provides full control over how data is populated, making it ideal for programmatically generating matrices and grid-based structures.
Conclusion
Python provides multiple ways to append values to a 2D array. If you’re working with standard lists, append()
, extend()
, and list comprehensions are effective choices. For high-performance numerical operations, NumPy’s append()
, hstack()
, and vstack()
provide optimized solutions. The right method depends on whether you’re handling small lists or large-scale numerical data.
Comparison of Methods
Method | Suitable For | Flexibility | Performance |
---|---|---|---|
append() |
Adding a row (Python list) | High | Moderate |
list comprehension |
Adding a column (Python list) | Moderate | High |
numpy.append() |
Appending rows/columns (NumPy) | High | High |
hstack() / vstack() |
Appending rows/columns efficiently (NumPy) | High | Very High |
+= Operator |
Quick row append (Python list) | Low | High |
extend() |
Appending multiple rows (Python list) | Moderate | High |
insert() |
Insert at a specific index (Python list) | Moderate | Moderate |
Nested loops | Dynamic data generation | High | Variable |