How to Get the First Row of DataFrame Pandas
-
Get the First Row of a Pandas DataFrame Using the
pandas.DataFrame.iloc
Property -
Get the First Row of a Pandas DataFrame Using the
pandas.DataFrame.head()
Method - Get the First Row of a Pandas DataFrame Based on the Specified Condition
-
Get the First Row of a Pandas DataFrame Using the
query()
Method -
Get the First Row of a Pandas DataFrame Using the
pandas.DataFrame.take()
Method - Get the First Row of a Pandas DataFrame Using Slicing
- Conclusion
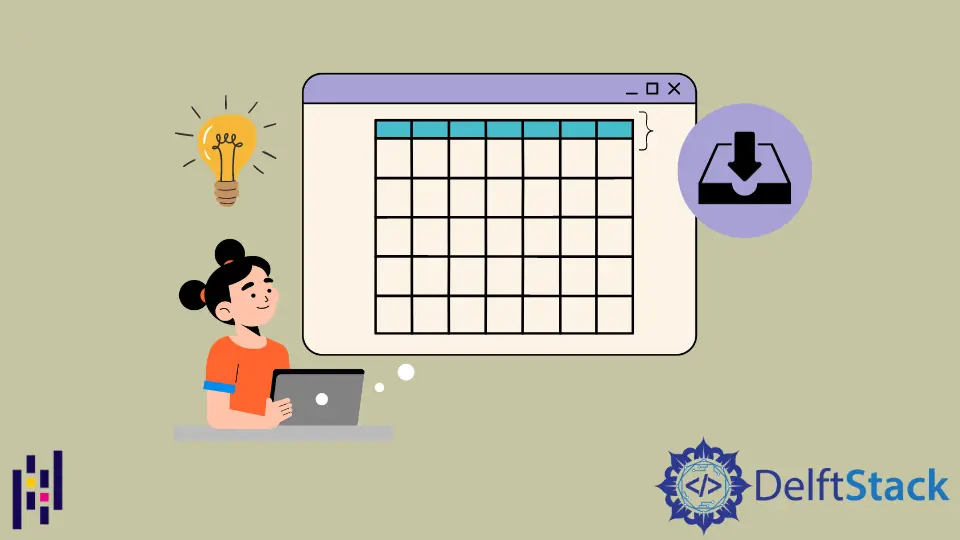
In this tutorial, we’ll discuss how we can get the first row from a Pandas DataFrame using the pandas.DataFrame.iloc
property, pandas.DataFrame.head()
method, and pandas.DataFrame.take()
Method. Additionally, we’ll also discuss getting the first row of a Pandas DataFrame based on specified conditions and using slicing.
We will use the DataFrame in the example below to explain how we can get the first row from a Pandas DataFrame.
import pandas as pd
df = pd.DataFrame(
{
"C_1": ["A", "B", "C", "D"],
"C_2": [40, 34, 38, 45],
"C_3": [430, 980, 200, 350],
}
)
print(df)
Output:
C_1 C_2 C_3
0 A 40 430
1 B 34 980
2 C 38 200
3 D 45 350
Get the First Row of a Pandas DataFrame Using the pandas.DataFrame.iloc
Property
The pandas.DataFrame.iloc
property is used to access specific rows and columns in a DataFrame using integer-based indexing. The iloc
property allows us to access rows and columns by their integer positions.
Basic Syntax:
data_frame.iloc[row_position, column_position]
Parameters:
row_position
: The integer position of the row we want to access.column_position
: The integer position of the column we want to access.
To get the first row, we would use the following:
first_row = data_frame.iloc[0]
In this case, 0
specifies the first row’s position, and there’s no need for additional parameters. Let’s give an example.
In the given code, we are working with a Pandas DataFrame df
. This DataFrame is constructed with three columns, "C_1"
, "C_2"
, and "C_3"
, and each column contains respective data.
We use df.iloc[0]
to access the first row of the DataFrame. This means we are retrieving the row with an integer position of 0
, and The resulting row_1
contains the data from the first row of the DataFrame.
import pandas as pd
df = pd.DataFrame(
{
"C_1": ["A", "B", "C", "D"],
"C_2": [40, 34, 38, 45],
"C_3": [430, 980, 200, 350],
}
)
row_1 = df.iloc[0]
print("The DataFrame:")
print(df, "\n")
print("The First Row of the DataFrame:")
print(row_1)
Output:
The DataFrame:
C_1 C_2 C_3
0 A 40 430
1 B 34 980
2 C 38 200
3 D 45 350
The First Row of the DataFrame:
C_1 A
C_2 40
C_3 430
Name: 0, dtype: object
The output displayed to the console shows the original DataFrame and the first row we extracted. The DataFrame consists of four rows (indexed from 0
to 3
) and three columns, where each row represents different data.
The first row, which we obtained using iloc
, is displayed as a Series with column names as index labels and the corresponding values from the first row. So, in this case, "C_1"
has a value of "A"
, "C_2"
has a value of 40
, and "C_3"
has a value of 430
.
Get the First Row of a Pandas DataFrame Using the pandas.DataFrame.head()
Method
To get the first row of a Pandas DataFrame using the pandas.DataFrame.head()
method, we can use this method to return the first 'n'
rows of the DataFrame, where 'n'
is the number of rows we want to retrieve.
Basic Syntax:
data_frame.head(n)
Parameter:
n
: The number of rows we want to retrieve from the beginning of the DataFrame. To get the first row, we should setn
to1
.
To get the first row, we would use the following:
first_row = data_frame.head(1)
In this case, 1
indicates that you want to retrieve the first row, and the result is returned as a new DataFrame containing that first row. Let’s give an example.
In the given code, we are working with a Pandas DataFrame named df
. This DataFrame is created with three columns: "C_1"
, "C_2"
, and "C_3"
, each containing different data values.
To extract the first row of the DataFrame, we use the df.head(1)
method. We specify 1
as the argument to head()
to indicate that we want to retrieve the first row, and this first row is assigned to the variable row_1
.
The code then proceeds to display the DataFrame and the first row. The DataFrame consists of four rows and three columns, each containing distinct data.
The first row, obtained with head(1)
, is displayed as a DataFrame with the same column names and the values corresponding to the first row.
import pandas as pd
df = pd.DataFrame(
{
"C_1": ["A", "B", "C", "D"],
"C_2": [40, 34, 38, 45],
"C_3": [430, 980, 200, 350],
}
)
row_1 = df.head(1)
print("The DataFrame:")
print(df, "\n")
print("The First Row of the DataFrame:")
print(row_1)
Output:
The DataFrame:
C_1 C_2 C_3
0 A 40 430
1 B 34 980
2 C 38 200
3 D 45 350
The First Row of the DataFrame:
C_1 C_2 C_3
0 A 40 430
The output, as shown in the console, includes the DataFrame and the first row. The DataFrame is displayed with all of its rows and columns, while the "The First Row of the DataFrame"
section presents the first row of the DataFrame with its column names and associated values.
Get the First Row of a Pandas DataFrame Based on the Specified Condition
To extract the first row satisfying specified conditions from a DataFrame, we first filter the rows satisfying specified conditions and then select the first row from the filtered DataFrame using the methods discussed above.
In this code, we’re working with a Pandas DataFrame named df
. The DataFrame is constructed with three columns: "C_1"
, "C_2"
, and "C_3"
, each containing different data values.
We then apply a filtering condition, where we want to find rows where column "C_2"
has values less than 40
and column "C_3"
has values greater than 450
. We use this condition to create a filtered DataFrame called filtered_df
.
After filtering, we use filtered_df.head(1)
to extract the first row from the filtered DataFrame that meets the specified condition. This row is stored in the variable row_1_filtered
.
import pandas as pd
df = pd.DataFrame(
{
"C_1": ["A", "B", "C", "D"],
"C_2": [40, 34, 38, 45],
"C_3": [430, 980, 500, 350],
}
)
filtered_df = df[(df.C_2 < 40) & (df.C_3 > 450)]
row_1_filtered = filtered_df.head(1)
print("The DataFrame is:")
print(df, "\n")
print("The Filtered DataFrame is:")
print(filtered_df, "\n")
print("The First Row with C_2 less than 45 and C_3 greater than 450 is:")
print(row_1_filtered)
Output:
The DataFrame is:
C_1 C_2 C_3
0 A 40 430
1 B 34 980
2 C 38 500
3 D 45 350
The Filtered DataFrame is:
C_1 C_2 C_3
1 B 34 980
2 C 38 500
The First Row with C_2 less than 45 and C_3 greater than 450 is:
C_1 C_2 C_3
1 B 34 980
The output presented in the console includes the original DataFrame, the filtered DataFrame, and the first row that satisfies the condition. The filtered DataFrame only retains rows where "C_2"
is less than 40
and "C_3"
is greater than 450
.
Get the First Row of a Pandas DataFrame Using the query()
Method
We can also use the query()
method to filter the rows from the DataFrame. It allows you to write the condition as a string and, in a sense, provides a more expressive and readable way to filter data.
Basic Syntax:
filtered_df = data_frame.query("condition")
Parameters:
data_frame
: The name of your DataFrame.'condition'
: A string that represents the filtering condition using column names and operators.
Let’s give an example. In this code, we begin by creating a Pandas DataFrame called df
. This DataFrame has three columns: "C_1"
, "C_2"
, and "C_3"
, each with different data values.
We then use the query()
method to filter the rows that meet the specified conditions. The condition is defined as "C_2"
being less than 40
and "C_3"
being greater than 450
. We store the resulting filtered DataFrame in the variable filtered_df
.
To extract the first row from the filtered DataFrame that satisfies the condition, we use filtered_df.head(1)
and store it in the variable row_1_filtered
. The output displayed in the console includes the original DataFrame, the filtered DataFrame, and the first row that meets the condition.
The filtered DataFrame retains only the rows that satisfy the criteria, and the first row that satisfies the specified conditions is presented with its column names and corresponding values. In this specific case, the first row has "B"
, 34
, and 980
for "C_1"
, "C_2"
, and "C_3"
, respectively.
import pandas as pd
df = pd.DataFrame(
{
"C_1": ["A", "B", "C", "D"],
"C_2": [40, 34, 38, 45],
"C_3": [430, 980, 500, 350],
}
)
filtered_df = df.query("(C_2 < 40) & (C_3 > 450)")
row_1_filtered = filtered_df.head(1)
print("The DataFrame is:")
print(df, "\n")
print("The Filtered DataFrame is:")
print(filtered_df, "\n")
print("The First Row with C_2 less than 45 and C_3 greater than 450 is:")
print(row_1_filtered)
Output:
The DataFrame is:
C_1 C_2 C_3
0 A 40 430
1 B 34 980
2 C 38 500
3 D 45 350
The Filtered DataFrame is:
C_1 C_2 C_3
1 B 34 980
2 C 38 500
The First Row with C_2 less than 45 and C_3 greater than 450 is:
C_1 C_2 C_3
1 B 34 980
It will filter all the rows with the value of column C_2
less than 45
and the value of C_3
column greater than 450
using the query()
method and then select the first row from the filtered_df
using the head()
method.
The query()
method is especially useful when you want to filter rows based on complex conditions.
Get the First Row of a Pandas DataFrame Using the pandas.DataFrame.take()
Method
The pandas.DataFrame.take()
method is used to get specific rows from a Pandas DataFrame by providing a list of the row positions you want to select. To get the first row of a DataFrame using the take()
method, you would provide a list containing just the integer position 0
since it represents the first row.
Basic Syntax:
selected_rows = data_frame.take(indices)
Parameters:
data_frame
: The name of your DataFrame.indices
: A list of integer positions that specify which rows you want to select.
To get the first row, we would use the following:
first_row = data_frame.take([0])
This will select the first row of the DataFrame and return it as a new DataFrame. Let’s give an example.
In this code, we begin by importing the Pandas library as pd
and create a Pandas DataFrame named df
. This DataFrame is composed of three columns: "C_1"
, "C_2"
, and "C_3"
, each containing distinct data values.
To extract the first row of the DataFrame, we utilize the df.take([0])
method. Here, the take()
method allows us to specify the row positions we want to select.
In this case, we provide a list containing just the integer position 0
, which corresponds to the first row in the DataFrame.
import pandas as pd
df = pd.DataFrame(
{
"C_1": ["A", "B", "C", "D"],
"C_2": [40, 34, 38, 45],
"C_3": [430, 980, 200, 350],
}
)
first_row_take = df.take([0])
print(first_row_take)
Output:
C_1 C_2 C_3
0 A 40 430
The output displayed in the console showcases the result of this operation. It presents the first row as a DataFrame with the same column names and the values associated with the first row.
In this specific example, the first row contains the values "A"
, 40
, and 430
for the "C_1"
, "C_2"
, and "C_3"
columns, respectively.
Get the First Row of a Pandas DataFrame Using Slicing
To get the first row of a Pandas DataFrame using slicing, you can use standard Python slicing notation. You would slice the DataFrame to include only the first row like this:
Syntax:
first_row = data_frame[:1]
The slicing method used to get the first row of a Pandas DataFrame doesn’t accept explicit parameters. Instead, you specify the range of rows you want by using Python slicing notation.
Specifically, you use [:1]
to indicate that you want to retrieve the first row. Let’s give an example.
In this code, we begin by importing the Pandas library as pd
and create a Pandas DataFrame named df
. This DataFrame is composed of three columns: "C_1"
, "C_2"
, and "C_3"
, each containing distinct data values.
To retrieve the first row of the DataFrame, we employ slicing. We use df[:1]
to specify that we want all rows up to, but not including, the row with index 1
, which essentially captures the first row(index 0
).
The resulting slice is stored in the first_row_slicing
variable.
import pandas as pd
df = pd.DataFrame(
{
"C_1": ["A", "B", "C", "D"],
"C_2": [40, 34, 38, 45],
"C_3": [430, 980, 200, 350],
}
)
first_row_slicing = df[:1]
print(first_row_slicing)
Output:
C_1 C_2 C_3
0 A 40 430
The output displayed in the console presents the result of this operation. It shows the first row of the DataFrame as a DataFrame itself, maintaining the original column names and the values associated with the first row.
In this specific example, the first row contains the values "A"
, 40
, and 430
for the "C_1"
, "C_2"
, and "C_3"
columns, respectively.
Conclusion
In conclusion, this tutorial has introduced various techniques for extracting the first row from a Pandas DataFrame. We explored methods such as pandas.DataFrame.iloc
, pandas.DataFrame.head()
, pandas.DataFrame.take()
, slicing and filtering based on specific conditions.
These methods offer diverse approaches to accessing and manipulating data, empowering data analysts to efficiently retrieve the initial row of a DataFrame while catering to different data analysis requirements. Pandas provides a versatile toolkit for data exploration and manipulation, making it an essential resource in the field of data analysis.
Suraj Joshi is a backend software engineer at Matrice.ai.
LinkedInRelated Article - Pandas DataFrame Row
- How to Get the Row Count of a Pandas DataFrame
- How to Randomly Shuffle DataFrame Rows in Pandas
- How to Filter Dataframe Rows Based on Column Values in Pandas
- How to Iterate Through Rows of a DataFrame in Pandas
- How to Get Index of All Rows Whose Particular Column Satisfies Given Condition in Pandas
- How to Find Duplicate Rows in a DataFrame Using Pandas