Pandas DataFrame の最初の行を取得する
-
pandas.DataFrame.iloc
プロパティを使用して Pandas DataFrame の最初の行を取得する - 指定した条件に基づいて Pandas の DataFrame から最初の行を取得する
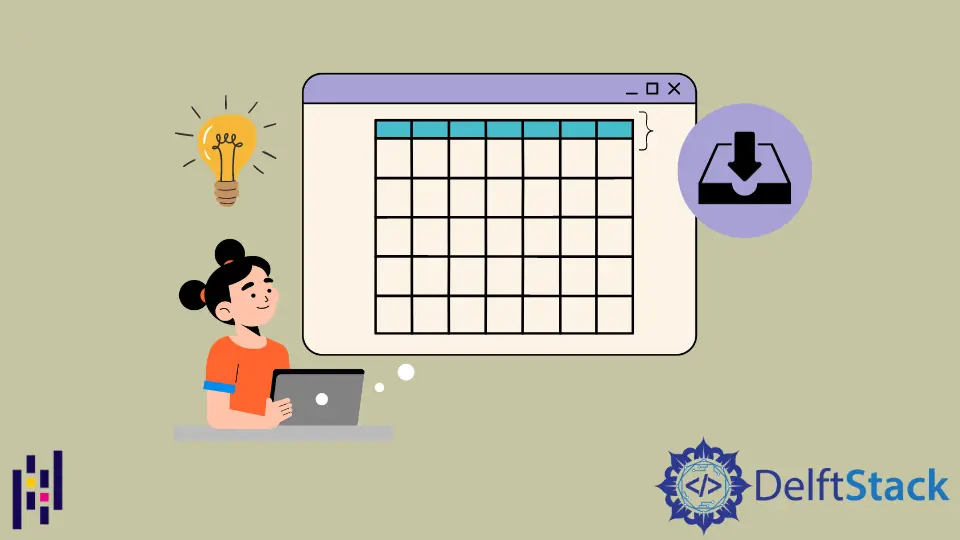
このチュートリアルでは、pandas.DataFrame.iloc
プロパティと pandas.DataFrame.head()
メソッドを使用して、Pandas DataFrame から最初の行を取得する方法を説明します。
以下の例では、Pandas の DataFrame から最初の行を取得する方法を説明します。
import pandas as pd
df = pd.DataFrame(
{
"C_1": ["A", "B", "C", "D"],
"C_2": [40, 34, 38, 45],
"C_3": [430, 980, 200, 350],
}
)
print(df)
出力:
C_1 C_2 C_3
0 A 40 430
1 B 34 980
2 C 38 200
3 D 45 350
pandas.DataFrame.iloc
プロパティを使用して Pandas DataFrame の最初の行を取得する
import pandas as pd
df = pd.DataFrame(
{
"C_1": ["A", "B", "C", "D"],
"C_2": [40, 34, 38, 45],
"C_3": [430, 980, 200, 350],
}
)
row_1 = df.iloc[0]
print("The DataFrame is:")
print(df, "\n")
print("The First Row of the DataFrame is:")
print(row_1)
出力:
The DataFrame is:
C_1 C_2 C_3
0 A 40 430
1 B 34 980
2 C 38 200
3 D 45 350
The First Row of the DataFrame is:
C_1 A
C_2 40
C_3 430
Name: 0, dtype: object
DataFrame df
の最初の行を表示します。最初の行を選択するには、DataFrame の iloc
プロパティで最初の行のデフォルトインデックス 0
を使用します。
pandas.DataFrame.head()
メソッドを使って Pandas DataFrame から最初の行を取得する
pandas.DataFrame.head()
メソッドは、最上位 5 行の DataFrame を返します。また、pandas.DataFrame.head()
メソッドの引数に最上段の行数を表す数値を渡すこともできます。pandas.DataFrame.head()
メソッドの引数に 1 を渡すと、DataFrame の最初の行のみを選択することができます。
import pandas as pd
df = pd.DataFrame(
{
"C_1": ["A", "B", "C", "D"],
"C_2": [40, 34, 38, 45],
"C_3": [430, 980, 200, 350],
}
)
row_1 = df.head(1)
print("The DataFrame is:")
print(df, "\n")
print("The First Row of the DataFrame is:")
print(row_1)
出力:
The DataFrame is:
C_1 C_2 C_3
0 A 40 430
1 B 34 980
2 C 38 200
3 D 45 350
The First Row of the DataFrame is:
C_1 C_2 C_3
0 A 40 430
指定した条件に基づいて Pandas の DataFrame から最初の行を取得する
DataFrame から指定された条件を満たす最初の行を抽出するには、まず指定された条件を満たす行をフィルタリングし、フィルタリングされた DataFrame から上述の方法で最初の行を選択します。
import pandas as pd
df = pd.DataFrame(
{
"C_1": ["A", "B", "C", "D"],
"C_2": [40, 34, 38, 45],
"C_3": [430, 980, 500, 350],
}
)
filtered_df = df[(df.C_2 < 40) & (df.C_3 > 450)]
row_1_filtered = filtered_df.head(1)
print("The DataFrame is:")
print(df, "\n")
print("The Filtered DataFrame is:")
print(filtered_df, "\n")
print("The First Row with C_2 less than 45 and C_3 greater than 450 is:")
print(row_1_filtered)
出力:
The DataFrame is:
C_1 C_2 C_3
0 A 40 430
1 B 34 980
2 C 38 500
3 D 45 350
The Filtered DataFrame is:
C_1 C_2 C_3
1 B 34 980
2 C 38 500
The First Row with C_2 less than 45 and C_3 greater than 450 is:
C_1 C_2 C_3
1 B 34 980
カラム C_2
の値が 45 以下でカラム C_3
の値が 450 以上の最初の行が表示されています。
また、query()
メソッドを使って DataFrame から行をフィルタリングすることもできます。
import pandas as pd
df = pd.DataFrame(
{
"C_1": ["A", "B", "C", "D"],
"C_2": [40, 34, 38, 45],
"C_3": [430, 980, 500, 350],
}
)
filtered_df = df.query("(C_2 < 40) & (C_3 > 450)")
row_1_filtered = filtered_df.head(1)
print("The DataFrame is:")
print(df, "\n")
print("The Filtered DataFrame is:")
print(filtered_df, "\n")
print("The First Row with C_2 less than 45 and C_3 greater than 450 is:")
print(row_1_filtered)
出力:
The DataFrame is:
C_1 C_2 C_3
0 A 40 430
1 B 34 980
2 C 38 500
3 D 45 350
The Filtered DataFrame is:
C_1 C_2 C_3
1 B 34 980
2 C 38 500
The First Row with C_2 less than 45 and C_3 greater than 450 is:
C_1 C_2 C_3
1 B 34 980
query()
メソッドを用いてカラム C_2
の値が 45 未満でカラム C_3
の値が 450 より大きい行をフィルタリングし、head()
メソッドを用いて filtered_df
から最初の行を選択します。
Suraj Joshi is a backend software engineer at Matrice.ai.
LinkedIn