Pandas の DataFrame の行を反復する方法
-
Pandas DataFrame の行を繰り返し処理する
index
属性 -
Python で DataFrame の行を繰り返し処理する
loc[]
メソッド -
Python で DataFrame の行を繰り返し処理する
iloc[]
メソッド -
行 Pandas を反復するための
pandas.DataFrame.iterrows()
-
行 Pandas を反復するための
pandas.DataFrame.itertuples
-
##行 Pandas を反復する
pandas.DataFrame.apply
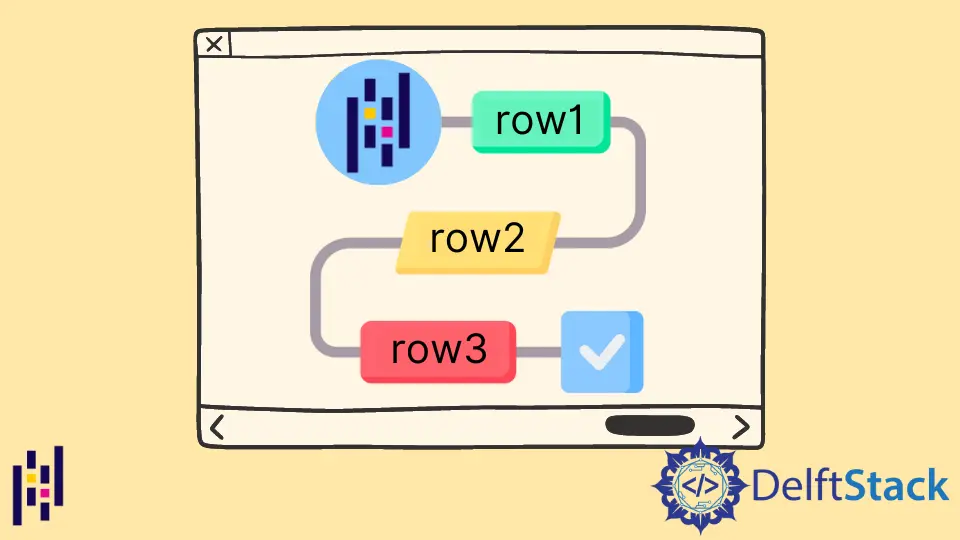
DataFrame の index
属性を使用して、Pandas DataFrame の行をループできます。DataFrame オブジェクトの loc()
、iloc()
、iterrows()
、itertuples()
、iteritems()
、および apply()
メソッドを使用して、Pandas DataFrame の行を繰り返し処理することもできます。
次のセクションでは、例として以下の DataFrame を使用します。
import pandas as pd
dates = ["April-10", "April-11", "April-12", "April-13", "April-14", "April-16"]
income1 = [10, 20, 10, 15, 10, 12]
income2 = [20, 30, 10, 5, 40, 13]
df = pd.DataFrame({"Date": dates, "Income_1": income1, "Income_2": income2})
print(df)
出力:
Date Income_1 Income_2
0 April-10 10 20
1 April-11 20 30
2 April-12 10 10
3 April-13 15 5
4 April-14 10 40
5 April-16 12 13
Pandas DataFrame の行を繰り返し処理する index
属性
Pandas DataFrame の index
属性は、DataFrame の最上行から最下行までの範囲オブジェクトを提供します。範囲を使用して、Pandas の行を反復できます。
import pandas as pd
dates = ["April-10", "April-11", "April-12", "April-13", "April-14", "April-16"]
income1 = [10, 20, 10, 15, 10, 12]
income2 = [20, 30, 10, 5, 40, 13]
df = pd.DataFrame({"Date": dates, "Income_1": income1, "Income_2": income2})
for i in df.index:
print(
"Total income in "
+ df["Date"][i]
+ " is:"
+ str(df["Income_1"][i] + df["Income_2"][i])
)
出力:
Total income in April-10 is:30
Total income in April-11 is:50
Total income in April-12 is:20
Total income in April-13 is:20
Total income in April-14 is:50
Total income in April-16 is:25
各行の Income_1
と Income_2
を追加し、総収入を出力します。
Python で DataFrame の行を繰り返し処理する loc[]
メソッド
loc[]
メソッドは、一度に 1つの行にアクセスするために使用されます。DataFrame のループ内で loc[]
メソッドを使用すると、DataFrame の行を繰り返し処理できます。
import pandas as pd
dates = ["April-10", "April-11", "April-12", "April-13", "April-14", "April-16"]
income1 = [10, 20, 10, 15, 10, 12]
income2 = [20, 30, 10, 5, 40, 13]
df = pd.DataFrame({"Date": dates, "Income_1": income1, "Income_2": income2})
for i in range(len(df)):
print(
"Total income in "
+ df.loc[i, "Date"]
+ " is:"
+ str(df.loc[i, "Income_1"] + df.loc[i, "Income_2"])
)
出力:
Total income in April-10 is:30
Total income in April-11 is:50
Total income in April-12 is:20
Total income in April-13 is:20
Total income in April-14 is:50
Total income in April-16 is:25
ここで、range(len(df))
は、DataFrame の行全体をループする範囲オブジェクトを生成します。
Python で DataFrame の行を繰り返し処理する iloc[]
メソッド
Pandas DataFrame の iloc
属性も loc
属性に非常に似ています。loc
と iloc
の唯一の違いは、loc
ではアクセスする行または列の名前を指定する必要があることですが、iloc
ではアクセスする行または列のインデックスを指定します。
import pandas as pd
dates = ["April-10", "April-11", "April-12", "April-13", "April-14", "April-16"]
income1 = [10, 20, 10, 15, 10, 12]
income2 = [20, 30, 10, 5, 40, 13]
df = pd.DataFrame({"Date": dates, "Income_1": income1, "Income_2": income2})
for i in range(len(df)):
print(
"Total income in " + df.iloc[i, 0] + " is:" + str(df.iloc[i, 1] + df.iloc[i, 2])
)
出力:
Total income in April-10 is:30
Total income in April-11 is:50
Total income in April-12 is:20
Total income in April-13 is:20
Total income in April-14 is:50
Total income in April-16 is:25
ここで、インデックス 0
は DataFrame の最初の列、つまり Date
を表し、インデックス 1
は Income_1
列を表し、インデックス 2
は Income_2
列を表します。
行 Pandas を反復するための pandas.DataFrame.iterrows()
pandas.DataFrame.iterrows()
は、インデックスを返します行と行のデータ全体をシリーズ
として。したがって、この関数を使用して、Pandas DataFrame の行を繰り返し処理できます。
import pandas as pd
dates = ["April-10", "April-11", "April-12", "April-13", "April-14", "April-16"]
income1 = [10, 20, 10, 15, 10, 12]
income2 = [20, 30, 10, 5, 40, 13]
df = pd.DataFrame({"Date": dates, "Income_1": income1, "Income_2": income2})
for index, row in df.iterrows():
print(
"Total income in "
+ row["Date"]
+ " is:"
+ str(row["Income_1"] + row["Income_2"])
)
出力:
Total income in April-10 is:30
Total income in April-11 is:50
Total income in April-12 is:20
Total income in April-13 is:20
Total income in April-14 is:50
Total income in April-16 is:25
行 Pandas を反復するための pandas.DataFrame.itertuples
pandas.DataFrame.itertuples
はオブジェクトを返し、各行のタプルを繰り返し処理します最初のフィールドはインデックスとして、残りのフィールドは列値として。したがって、この関数を使用して Pandas DataFrame の行を繰り返し処理することもできます。
import pandas as pd
dates = ["April-10", "April-11", "April-12", "April-13", "April-14", "April-16"]
income1 = [10, 20, 10, 15, 10, 12]
income2 = [20, 30, 10, 5, 40, 13]
df = pd.DataFrame({"Date": dates, "Income_1": income1, "Income_2": income2})
for row in df.itertuples():
print("Total income in " + row.Date + " is:" + str(row.Income_1 + row.Income_2))
出力:
Total income in April-10 is:30
Total income in April-11 is:50
Total income in April-12 is:20
Total income in April-13 is:20
Total income in April-14 is:50
Total income in April-16 is:25
##行 Pandas を反復する pandas.DataFrame.apply
pandas.DataFrame.apply
は DataFrame を返します
DataFrame の特定の軸に沿って特定の関数を適用した結果として。
構文:
DataFrame.apply(self, func, axis=0, raw=False, result_type=None, args=(), **kwds)
ここで、func
は適用される関数を表し、axis
は関数が適用される軸を表します。axis = 1
または axis = 'columns'
を使用して、各行に関数を適用できます。
import pandas as pd
dates = ["April-10", "April-11", "April-12", "April-13", "April-14", "April-16"]
income1 = [10, 20, 10, 15, 10, 12]
income2 = [20, 30, 10, 5, 40, 13]
df = pd.DataFrame({"Date": dates, "Income_1": income1, "Income_2": income2})
print(
df.apply(
lambda row: "Total income in "
+ row["Date"]
+ " is:"
+ str(row["Income_1"] + row["Income_2"]),
axis=1,
)
)
出力:
0 Total income in April-10 is:30
1 Total income in April-11 is:50
2 Total income in April-12 is:20
3 Total income in April-13 is:20
4 Total income in April-14 is:50
5 Total income in April-16 is:25
dtype: object
ここでは、lambda
キーワードを使用して、各行に適用されるインライン関数を定義しています。
Suraj Joshi is a backend software engineer at Matrice.ai.
LinkedIn関連記事 - Pandas DataFrame
- Pandas DataFrame の列ヘッダーをリストとして取得する方法
- Pandas DataFrame 列を削除する方法
- Pandas で DataFrame 列を日時に変換する方法
- Pandas DataFrame で浮動小数点数 float を整数 int に変換する方法
- Pandas DataFrame を 1つの列の値で並べ替える方法
- Pandas group-by と Sum の集計を取得する方法