Pandas에서 DataFrame의 행을 반복하는 방법
-
Pandas DataFrame에서 행을 반복하는
index
속성 -
파이썬에서 DataFrame의 행을 반복하는
loc[]
메소드 -
파이썬에서 DataFrame의 행을 반복하는
iloc[]
메소드 -
pandas.DataFrame.iterrows()
는 행 Pandas를 반복합니다 -
pandas.DataFrame.itertuples
는 행 Pandas를 반복합니다 -
pandas.DataFrame.apply
는 행 Pandas를 반복합니다
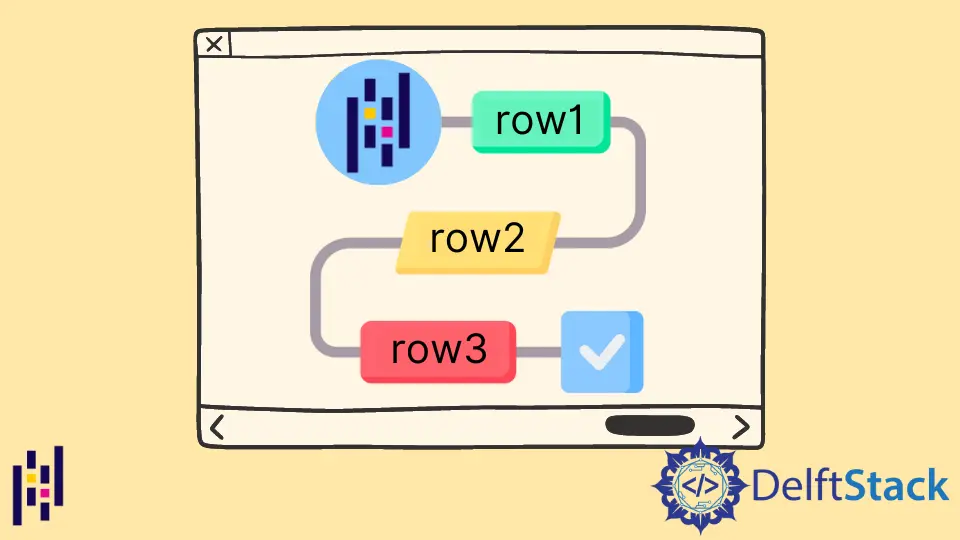
DataFrame의 index 속성을 사용하여 Pandas DataFrame의 행을 반복 할 수 있습니다. DataFrame 객체의loc()
,iloc()
,iterrows()
,itertuples()
,iteritems()
및apply()
메소드를 사용하여 DataFrame Pandas 행을 반복 할 수도 있습니다.
다음 섹션에서 아래 데이터 프레임을 예로 사용합니다.
import pandas as pd
dates = ["April-10", "April-11", "April-12", "April-13", "April-14", "April-16"]
income1 = [10, 20, 10, 15, 10, 12]
income2 = [20, 30, 10, 5, 40, 13]
df = pd.DataFrame({"Date": dates, "Income_1": income1, "Income_2": income2})
print(df)
출력:
Date Income_1 Income_2
0 April-10 10 20
1 April-11 20 30
2 April-12 10 10
3 April-13 15 5
4 April-14 10 40
5 April-16 12 13
Pandas DataFrame에서 행을 반복하는index
속성
Pandas DataFrame의 index
속성은 DataFrame의 맨 위 행에서 맨 아래 행까지 범위 객체를 제공합니다. 범위를 사용하여 Pandas의 행을 반복 할 수 있습니다.
import pandas as pd
dates = ["April-10", "April-11", "April-12", "April-13", "April-14", "April-16"]
income1 = [10, 20, 10, 15, 10, 12]
income2 = [20, 30, 10, 5, 40, 13]
df = pd.DataFrame({"Date": dates, "Income_1": income1, "Income_2": income2})
for i in df.index:
print(
"Total income in "
+ df["Date"][i]
+ " is:"
+ str(df["Income_1"][i] + df["Income_2"][i])
)
출력:
Total income in April-10 is:30
Total income in April-11 is:50
Total income in April-12 is:20
Total income in April-13 is:20
Total income in April-14 is:50
Total income in April-16 is:25
각 행에 Income_1
과 Income_2
를 더하고 총 수입을 인쇄합니다.
파이썬에서 DataFrame의 행을 반복하는loc[]
메소드
loc[]
메소드는 한 번에 한 행에 액세스하는 데 사용됩니다. DataFrame을 통한 루프 내에서loc[]
메소드를 사용하면 DataFrame의 행을 반복 할 수 있습니다.
import pandas as pd
dates = ["April-10", "April-11", "April-12", "April-13", "April-14", "April-16"]
income1 = [10, 20, 10, 15, 10, 12]
income2 = [20, 30, 10, 5, 40, 13]
df = pd.DataFrame({"Date": dates, "Income_1": income1, "Income_2": income2})
for i in range(len(df)):
print(
"Total income in "
+ df.loc[i, "Date"]
+ " is:"
+ str(df.loc[i, "Income_1"] + df.loc[i, "Income_2"])
)
출력:
Total income in April-10 is:30
Total income in April-11 is:50
Total income in April-12 is:20
Total income in April-13 is:20
Total income in April-14 is:50
Total income in April-16 is:25
여기서range(len(df))
는 DataFrame의 전체 행을 반복하는 범위 객체를 생성합니다.
파이썬에서 DataFrame의 행을 반복하는iloc[]
메소드
Pandas DataFrame iloc
속성은loc
속성과 매우 유사합니다. loc
과iloc
의 유일한 차이점은loc
에서는 액세스 할 행 또는 열의 이름을 지정해야하고 iloc
에서는 액세스 할 행 또는 열의 인덱스를 지정해야한다는 것입니다.
import pandas as pd
dates = ["April-10", "April-11", "April-12", "April-13", "April-14", "April-16"]
income1 = [10, 20, 10, 15, 10, 12]
income2 = [20, 30, 10, 5, 40, 13]
df = pd.DataFrame({"Date": dates, "Income_1": income1, "Income_2": income2})
for i in range(len(df)):
print(
"Total income in " + df.iloc[i, 0] + " is:" + str(df.iloc[i, 1] + df.iloc[i, 2])
)
출력:
Total income in April-10 is:30
Total income in April-11 is:50
Total income in April-12 is:20
Total income in April-13 is:20
Total income in April-14 is:50
Total income in April-16 is:25
여기서 인덱스 0
은 DataFrame의 첫 번째 열, 즉 Date
를 나타내고, 인덱스 1
은 Income_1
열을, 인덱스 2
는 Income_2
열을 나타냅니다.
pandas.DataFrame.iterrows()
는 행 Pandas를 반복합니다
pandas.DataFrame.iterrows()
의 색인을 반환합니다 Series
로서 행과 행의 전체 데이터. 따라서이 함수를 사용하여 Pandas DataFrame의 행을 반복 할 수 있습니다.
import pandas as pd
dates = ["April-10", "April-11", "April-12", "April-13", "April-14", "April-16"]
income1 = [10, 20, 10, 15, 10, 12]
income2 = [20, 30, 10, 5, 40, 13]
df = pd.DataFrame({"Date": dates, "Income_1": income1, "Income_2": income2})
for index, row in df.iterrows():
print(
"Total income in "
+ row["Date"]
+ " is:"
+ str(row["Income_1"] + row["Income_2"])
)
출력:
Total income in April-10 is:30
Total income in April-11 is:50
Total income in April-12 is:20
Total income in April-13 is:20
Total income in April-14 is:50
Total income in April-16 is:25
pandas.DataFrame.itertuples
는 행 Pandas를 반복합니다
pandas.DataFrame.itertuples
는 각 행에 대한 튜플을 반복하는 객체를 반환합니다. 첫 번째 필드는 인덱스이고 나머지 필드는 열 값입니다. 따라서이 함수를 사용하여 Pandas DataFrame의 행을 반복 할 수도 있습니다.
import pandas as pd
dates = ["April-10", "April-11", "April-12", "April-13", "April-14", "April-16"]
income1 = [10, 20, 10, 15, 10, 12]
income2 = [20, 30, 10, 5, 40, 13]
df = pd.DataFrame({"Date": dates, "Income_1": income1, "Income_2": income2})
for row in df.itertuples():
print("Total income in " + row.Date + " is:" + str(row.Income_1 + row.Income_2))
출력:
Total income in April-10 is:30
Total income in April-11 is:50
Total income in April-12 is:20
Total income in April-13 is:20
Total income in April-14 is:50
Total income in April-16 is:25
pandas.DataFrame.apply
는 행 Pandas를 반복합니다
pandas.DataFrame.apply
는 DataFrame을 반환합니다.
DataFrame의 주어진 축을 따라 주어진 함수를 적용한 결과.
통사론:
DataFrame.apply(self, func, axis=0, raw=False, result_type=None, args=(), **kwds)
여기서 func
는 적용 할 함수를 나타내고 axis
는 함수가 적용되는 축을 나타냅니다. axis=1
또는axis = 'columns'
를 사용하여 각 행에 함수를 적용 할 수 있습니다.
import pandas as pd
dates = ["April-10", "April-11", "April-12", "April-13", "April-14", "April-16"]
income1 = [10, 20, 10, 15, 10, 12]
income2 = [20, 30, 10, 5, 40, 13]
df = pd.DataFrame({"Date": dates, "Income_1": income1, "Income_2": income2})
print(
df.apply(
lambda row: "Total income in "
+ row["Date"]
+ " is:"
+ str(row["Income_1"] + row["Income_2"]),
axis=1,
)
)
출력:
0 Total income in April-10 is:30
1 Total income in April-11 is:50
2 Total income in April-12 is:20
3 Total income in April-13 is:20
4 Total income in April-14 is:50
5 Total income in April-16 is:25
dtype: object
여기서 lambda
키워드는 각 행에 적용되는 인라인 함수를 정의하는 데 사용됩니다.
Suraj Joshi is a backend software engineer at Matrice.ai.
LinkedIn관련 문장 - Pandas DataFrame
- Pandas DataFrame 열 헤더를 목록으로 가져 오는 방법
- Pandas DataFrame 열을 삭제하는 방법
- Pandas 에서 DataFrame 열을 Datetime 으로 변환하는 방법
- Pandas DataFrame에서 float를 정수로 변환하는 방법
- 한 열의 값으로 Pandas DataFrame 을 정렬하는 방법
- Pandas 그룹 및 합계를 집계하는 방법