Pandas DataFrame의 행 수를 얻는 방법
Asad Riaz
2023년1월30일
-
.shape
메소드로DataFrame
의 행 개수를 얻습니다 -
Pandas에서 행 수를 얻는 가장 빠른 방법 인
.len(DataFrame.index)
-
Pandas의 조건을 만족하는 행을 계산하는
dataframe.apply()
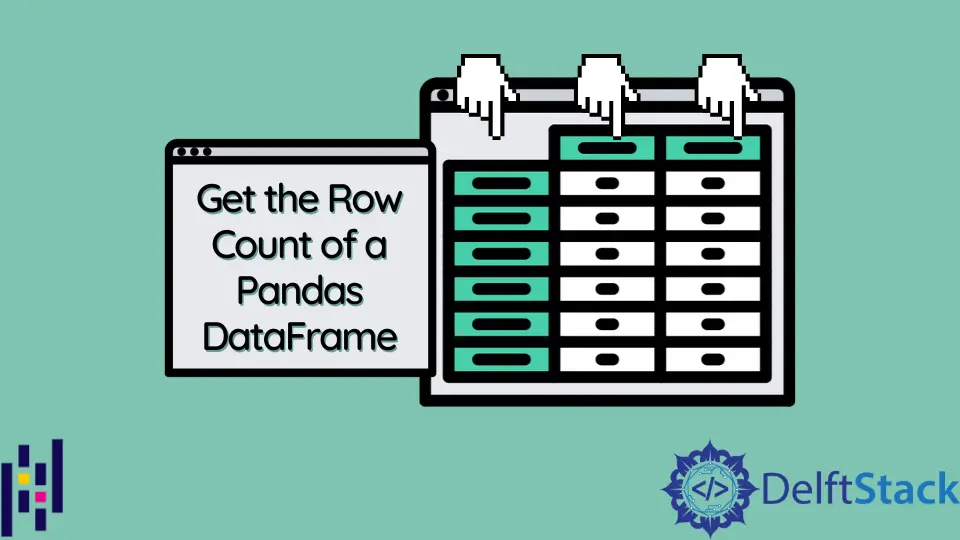
shape
와len(DataFrame.index)
와 같은 다른 방법으로 Pandas DataFrame
의 행 수를 얻는 방법을 소개합니다. len(DataFrame.index)
가 가장 빠르다는 점에서 주목할만한 성능 차이가 있습니다.
또한 dataframe.apply()
를 사용하여 조건을 만족시키는 행 요소 수를 얻는 방법을 살펴 봅니다.
.shape
메소드로DataFrame
의 행 개수를 얻습니다
df
가DataFrame
이라고 가정하고 행 개수를 계산합니다.
# python 3.x
import pandas as pd
import numpy as np
df = pd.DataFrame(np.arange(15).reshape(3, 5))
print(df)
print("Row count is:", df.shape[0])
출력:
0 1 2 3 4
0 0 1 2 3 4
1 5 6 7 8 9
2 10 11 12 13 14
Row count is: 3
열 개수에는df.shape[1]
을 사용할 수 있습니다.
Pandas에서 행 수를 얻는 가장 빠른 방법 인.len(DataFrame.index)
인덱스 행의 길이를 가져 와서 DataFrame
에서 행 수를 계산할 수 있습니다.
# python 3.x
import pandas as pd
import numpy as np
df = pd.DataFrame(np.arange(15).reshape(3, 5))
print(df)
print("Row count is:", len(df.index))
출력:
0 1 2 3 4
0 0 1 2 3 4
1 5 6 7 8 9
2 10 11 12 13 14
Row count is: 3
df.index
대신df.axes[0]
를 전달할 수도 있습니다:
# python 3.x
import pandas as pd
import numpy as np
df = pd.DataFrame(np.arange(15).reshape(3, 5))
print(df)
print("Row count is:", len(df.axes[0]))
출력:
0 1 2 3 4
0 0 1 2 3 4
1 5 6 7 8 9
2 10 11 12 13 14
Row count is: 3
열 수에 대해서는df.axes[1]
을 사용할 수 있습니다.
Pandas의 조건을 만족하는 행을 계산하는dataframe.apply()
반환 된dataframe.apply()
결과에서True
의 수를 세면 조건을 만족하는DataFrame
의 행 수를 얻을 수 있습니다.
# python 3.x
import pandas as pd
import numpy as np
df = pd.DataFrame(np.arange(15).reshape(3, 5))
counterFunc = df.apply(lambda x: True if x[1] > 3 else False, axis=1)
numOfRows = len(counterFunc[counterFunc == True].index)
print(df)
print("Row count > 3 in column[1]is:", numOfRows)
출력:
0 1 2 3 4
0 0 1 2 3 4
1 5 6 7 8 9
2 10 11 12 13 14
Row count > 3 in column[1]is: 2
column[1]
의 값이 3보다 큰 행의 개수를 얻습니다.