如何獲取 Pandas DataFrame 的行數
Asad Riaz
2023年1月30日
Pandas
Pandas DataFrame Row
-
.shape
方法獲取資料DataFrame
的行數 -
.len(DataFrame.index)
獲取 Pandas 行數的最快方法 -
dataframe.apply()
計算滿足 Pandas 條件的行
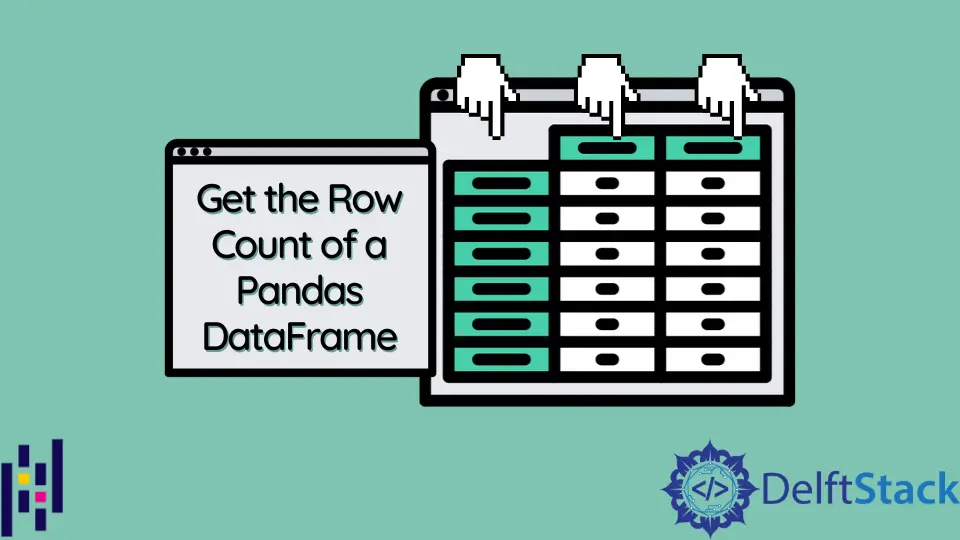
我們將介紹如何使用 shape
和 len(DataFrame.index)
之類的不同方法來獲取 Pandas DataFrame
的行數。我們發現,len(DataFrame.index)
是最快的,存在明顯的效能差異。
我們還將研究如何使用 dataframe.apply()
獲取行中有多少個元素滿足條件。
.shape
方法獲取資料 DataFrame
的行數
假設 df 是我們的 DataFrame
,對於計算行數來說,
# python 3.x
import pandas as pd
import numpy as np
df = pd.DataFrame(np.arange(15).reshape(3, 5))
print(df)
print("Row count is:", df.shape[0])
輸出:
0 1 2 3 4
0 0 1 2 3 4
1 5 6 7 8 9
2 10 11 12 13 14
Row count is: 3
對於列數,我們可以使用 df.shape[1]
。
.len(DataFrame.index)
獲取 Pandas 行數的最快方法
我們可以通過獲取成員變數的長度索引來計算 DataFrame
中的行:
# python 3.x
import pandas as pd
import numpy as np
df = pd.DataFrame(np.arange(15).reshape(3, 5))
print(df)
print("Row count is:", len(df.index))
輸出:
0 1 2 3 4
0 0 1 2 3 4
1 5 6 7 8 9
2 10 11 12 13 14
Row count is: 3
我們還可以傳遞 df.axes[0]
而不是 df.index
:
# python 3.x
import pandas as pd
import numpy as np
df = pd.DataFrame(np.arange(15).reshape(3, 5))
print(df)
print("Row count is:", len(df.axes[0]))
輸出:
0 1 2 3 4
0 0 1 2 3 4
1 5 6 7 8 9
2 10 11 12 13 14
Row count is: 3
對於列數,我們可以使用 df.axes[1]
。
dataframe.apply()
計算滿足 Pandas 條件的行
通過計算返回的 dataframe.apply()
結果序列中 True
的數目,我們可以得到滿足條件的 DataFrame
中的行的元素。
# python 3.x
import pandas as pd
import numpy as np
df = pd.DataFrame(np.arange(15).reshape(3, 5))
counterFunc = df.apply(lambda x: True if x[1] > 3 else False, axis=1)
numOfRows = len(counterFunc[counterFunc == True].index)
print(df)
print("Row count > 3 in column[1]is:", numOfRows)
輸出:
0 1 2 3 4
0 0 1 2 3 4
1 5 6 7 8 9
2 10 11 12 13 14
Row count > 3 in column[1]is: 2
我們得到行中 column[1] > 3
的行數。
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe