Pandas 中如何獲取特定列滿足給定條件的所有行的索引
Suraj Joshi
2023年1月30日
- 簡單的索引操作可獲取 Pandas 中特定列滿足給定條件的所有行的索引
-
np.where()
方法獲取特定列滿足給定條件的所有行的索引 -
pandas.DataFrame.query()
獲取特定列滿足給定條件的所有行的索引
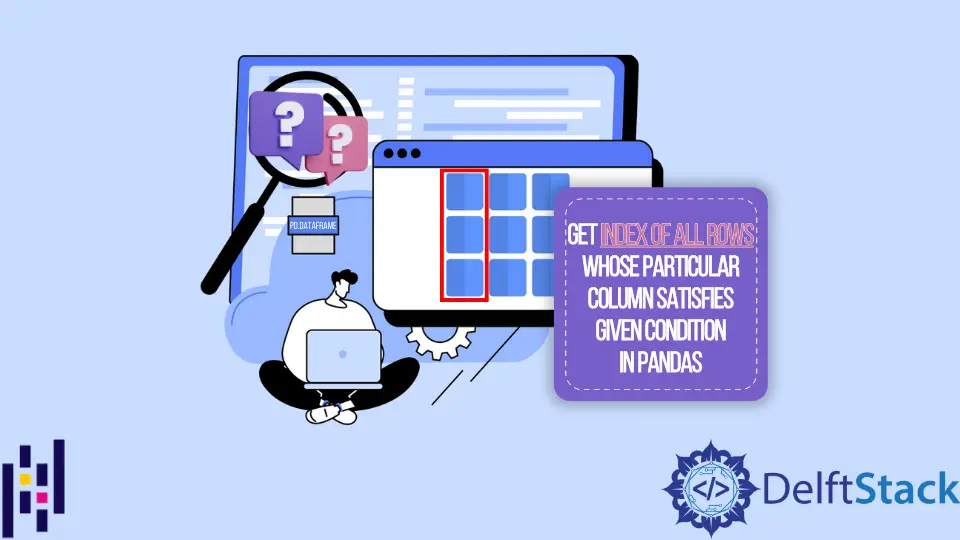
我們可以使用簡單的索引操作獲得特定列滿足給定條件的所有行的索引。我們還可以使用 NumPy 包中的 where()
方法和 DataFrame 物件的 query()
方法找到它們的索引。
簡單的索引操作可獲取 Pandas 中特定列滿足給定條件的所有行的索引
使用簡單的索引操作可以完成獲取特定列滿足給定條件的行的索引的任務。
import pandas as pd
import numpy as np
dates = ["April-10", "April-11", "April-12", "April-13", "April-14", "April-16"]
sales = [200, 300, 400, 200, 300, 300]
prices = [3, 1, 2, 4, 3, 2]
df = pd.DataFrame({"Date": dates, "Sales": sales, "Price": prices})
reqd_Index = df[df["Sales"] >= 300].index.tolist()
print(reqd_Index)
輸出:
[1, 2, 4, 5]
這裡,df['Sales']>=300
給出一系列布林值,如果其 Sales
列的值大於或等於 300,則其元素為 True。
我們可以通過使用 df[df['Sales']>=300].index
來檢索銷售值大於或等於 300 的行的索引。
最後,tolist()
方法將所有索引轉換為列表。
np.where()
方法獲取特定列滿足給定條件的所有行的索引
np.where()
將條件作為輸入,並返回滿足給定條件的元素的索引。因此,我們可以使用 np.where()
來獲取特定列滿足給定條件的所有行的索引。
import pandas as pd
import numpy as np
dates = ["April-10", "April-11", "April-12", "April-13", "April-14", "April-16"]
sales = [200, 300, 400, 200, 300, 300]
prices = [3, 1, 2, 4, 3, 2]
df = pd.DataFrame({"Date": dates, "Sales": sales, "Price": prices})
reqd_Index = list(np.where(df["Sales"] >= 300))
print(reqd_Index)
輸出:
[array([1, 2, 4, 5])]
這將輸出 Sales
列中的值大於或等於 300
的所有行的索引。
pandas.DataFrame.query()
獲取特定列滿足給定條件的所有行的索引
pandas.DataFrame.query()
返回由提供的查詢表示式產生的 DataFrame。現在,我們可以使用 DataFrame 的 index 屬性返回其特定列滿足給定條件的所有行的索引。
import pandas as pd
import numpy as np
dates = ["April-10", "April-11", "April-12", "April-13", "April-14", "April-16"]
sales = [200, 300, 400, 200, 300, 300]
prices = [3, 1, 2, 4, 3, 2]
df = pd.DataFrame({"Date": dates, "Sales": sales, "Price": prices})
reqd_index = df.query("Sales == 300").index.tolist()
print(reqd_index)
輸出:
[1, 4, 5]
它返回特定列滿足給定條件 Sales == 300
的所有行的索引列表。
作者: Suraj Joshi
Suraj Joshi is a backend software engineer at Matrice.ai.
LinkedIn相關文章 - Pandas DataFrame
- 如何將 Pandas DataFrame 列標題獲取為列表
- 如何刪除 Pandas DataFrame 列
- 如何在 Pandas 中將 DataFrame 列轉換為日期時間
- 如何在 Pandas DataFrame 中將浮點數轉換為整數
- 如何按一列的值對 Pandas DataFrame 進行排序
- 如何用 group-by 和 sum 獲得 Pandas 總和
相關文章 - Pandas DataFrame Row
- 如何獲取 Pandas DataFrame 的行數
- 如何對 Pandas 中的 DataFrame 行隨機排序
- 如何根據 Pandas 中的列值過濾 DataFrame 行
- 如何在 Pandas 中遍歷 DataFrame 的行
- Pandas DataFrame 刪除某行