How to Get Index of All Rows Whose Particular Column Satisfies Given Condition in Pandas
- Simple Indexing Operation to Get the Index of All Rows Whose Particular Column Satisfies Given Condition
-
np.where()
Method to Get Index of All Rows Whose Particular Column Satisfies Given Condition -
pandas.DataFrame.query()
to Get Indices of All Rows Whose Particular Column Satisfies Given Condition
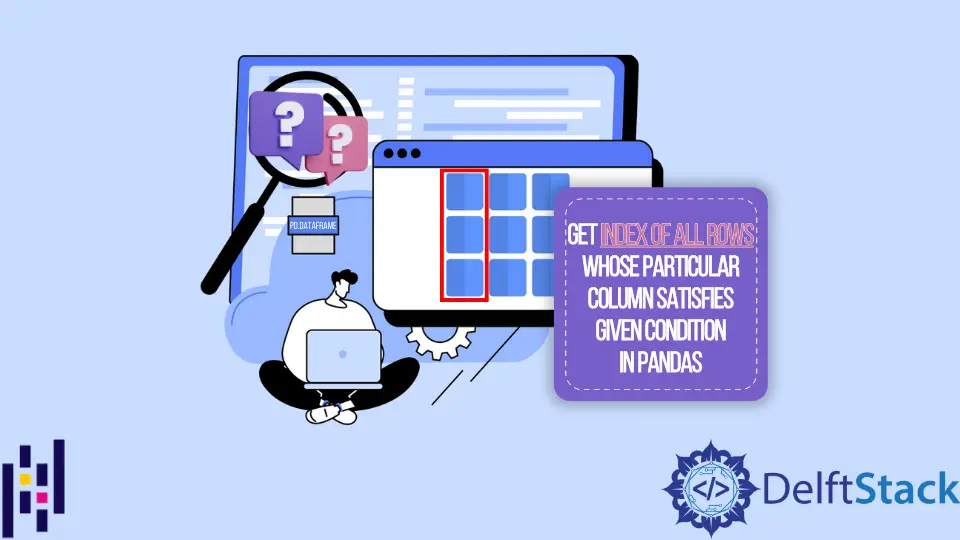
We can get the index of all rows whose particular column satisfies given condition in Pandas using simple indexing operation. We could also find their indices using the where()
method from NumPy package and query()
method of DataFrame object.
Simple Indexing Operation to Get the Index of All Rows Whose Particular Column Satisfies Given Condition
The use of simple indexing operation can accomplish the task of getting the index of rows whose particular column meets the given condition.
import pandas as pd
import numpy as np
dates = ["April-10", "April-11", "April-12", "April-13", "April-14", "April-16"]
sales = [200, 300, 400, 200, 300, 300]
prices = [3, 1, 2, 4, 3, 2]
df = pd.DataFrame({"Date": dates, "Sales": sales, "Price": prices})
reqd_Index = df[df["Sales"] >= 300].index.tolist()
print(reqd_Index)
Output:
[1, 2, 4, 5]
Here, df['Sales']>=300
gives series of boolean values whose elements are True
if their Sales
column has a value greater than or equal to 300.
We can retrieve the index of rows whose Sales
value is greater than or equal to 300 by using df[df['Sales']>=300].index
.
Finally, the tolist()
method converts all the indices to a list.
np.where()
Method to Get Index of All Rows Whose Particular Column Satisfies Given Condition
np.where()
takes condition as an input and returns the indices of elements that satisfy the given condition. Hence, we could use np.where()
to get indices of all rows whose particular column satisfies the given condition.
import pandas as pd
import numpy as np
dates = ["April-10", "April-11", "April-12", "April-13", "April-14", "April-16"]
sales = [200, 300, 400, 200, 300, 300]
prices = [3, 1, 2, 4, 3, 2]
df = pd.DataFrame({"Date": dates, "Sales": sales, "Price": prices})
reqd_Index = list(np.where(df["Sales"] >= 300))
print(reqd_Index)
Output:
[array([1, 2, 4, 5])]
This outputs indices of all the rows whose values in the Sales
column are greater than or equal to 300
.
pandas.DataFrame.query()
to Get Indices of All Rows Whose Particular Column Satisfies Given Condition
pandas.DataFrame.query()
returns DataFrame resulting from the provided query expression. Now, we can use the index
attribute of DataFrame to return indices of all the rows whose particular column satisfies the given condition.
import pandas as pd
import numpy as np
dates = ["April-10", "April-11", "April-12", "April-13", "April-14", "April-16"]
sales = [200, 300, 400, 200, 300, 300]
prices = [3, 1, 2, 4, 3, 2]
df = pd.DataFrame({"Date": dates, "Sales": sales, "Price": prices})
reqd_index = df.query("Sales == 300").index.tolist()
print(reqd_index)
Output:
[1, 4, 5]
It returns the list of indices of all rows whose particular column satisfies the given condition Sales == 300
.
Suraj Joshi is a backend software engineer at Matrice.ai.
LinkedInRelated Article - Pandas DataFrame
- How to Get Pandas DataFrame Column Headers as a List
- How to Delete Pandas DataFrame Column
- How to Convert Pandas Column to Datetime
- How to Convert a Float to an Integer in Pandas DataFrame
- How to Sort Pandas DataFrame by One Column's Values
- How to Get the Aggregate of Pandas Group-By and Sum
Related Article - Pandas DataFrame Row
- How to Get the Row Count of a Pandas DataFrame
- How to Randomly Shuffle DataFrame Rows in Pandas
- How to Filter Dataframe Rows Based on Column Values in Pandas
- How to Iterate Through Rows of a DataFrame in Pandas
- How to Find Duplicate Rows in a DataFrame Using Pandas