How to Extract the Filename From a Path Using PowerShell
-
Use the
Split-Path
Cmdlet to Extract the Filename From a Path in PowerShell - Use .NET’s Path Class Methods to Extract the Filename From a Path in PowerShell
-
Use the
Get-Item
andGet-ChildItem
Cmdlets to Extract the Filename From a Path in PowerShell - Use Regular Expressions to Extract the Filename From a Path in PowerShell
- Use String Manipulation to Extract the Filename From a Path in PowerShell
-
Use the
System.IO.FileInfo
Class to Extract the Filename From a Path in PowerShell - Conclusion
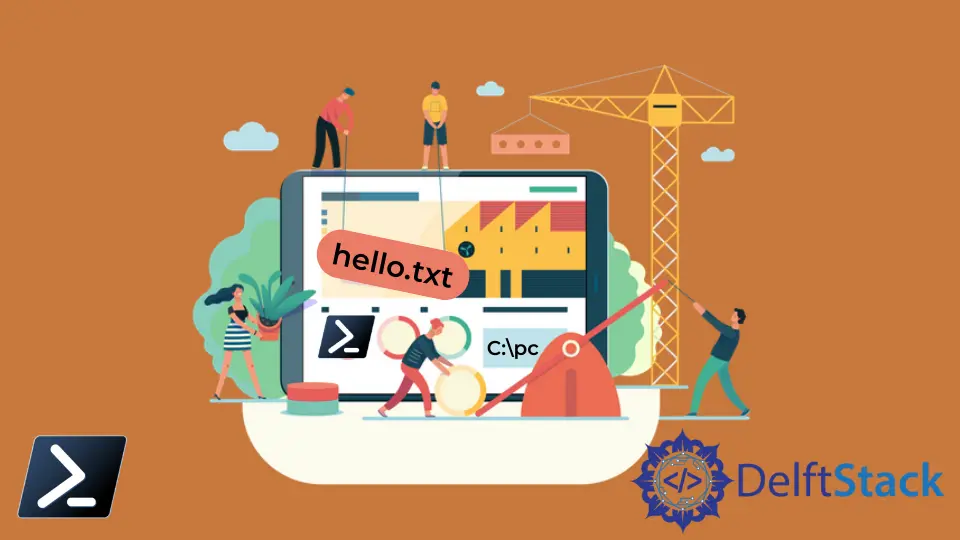
A file path tells the location of the file on the system. While working with files in PowerShell, you may need to get only the file name from a path.
There are multiple ways to get the path of the files in PowerShell. This tutorial will teach you to extract the filename from a file path with PowerShell.
Use the Split-Path
Cmdlet to Extract the Filename From a Path in PowerShell
In PowerShell, the Split-Path
cmdlet is a handy tool for isolating specific segments of a given path. Whether it’s the parent folder, subfolder, file name, or just the file extension, Split-Path
allows you to target and display these elements.
Extract the Filename With Extension
To capture the filename along with its extension from a path, you can employ the Split-Path
command with the -Leaf
parameter:
Split-Path C:\pc\test_folder\hello.txt -Leaf
Output:
hello.txt
Obtain the Filename Without an Extension
In cases where you need the filename without the extension, the -LeafBase
parameter comes in handy. However, it’s important to note that this parameter is available in PowerShell versions 6.0 and later:
Split-Path C:\pc\test_folder\hello.txt -LeafBase
Output:
hello
Use .NET’s Path Class Methods to Extract the Filename From a Path in PowerShell
In .NET, the Path
class offers useful methods to easily extract the filename and extension from a specified path. Let’s explore these capabilities with examples.
Extract Filename With Extension Using GetFileName
The GetFileName
method of the Path
class retrieves the file name along with its extension from a given path. Let’s demonstrate this with the path C:\pc\test_folder\hello.txt
:
[System.IO.Path]::GetFileName('C:\pc\test_folder\hello.txt')
Output:
hello.txt
Obtain Filename Without Extension Using GetFileNameWithoutExtension
To extract only the filename without the extension, you can use the GetFileNameWithoutExtension
method. This is particularly useful when you need the name of the file itself, excluding the file type:
[System.IO.Path]::GetFileNameWithoutExtension('C:\pc\test_folder\hello.txt')
Output:
hello
Use the Get-Item
and Get-ChildItem
Cmdlets to Extract the Filename From a Path in PowerShell
In PowerShell, the Get-Item
and Get-ChildItem
cmdlets are essential tools for working with files and directories, allowing you to extract specific information about the item at a given location.
Let’s see how to use these cmdlets to extract the filename from a given path, including variations to obtain the filename with or without the extension.
Extract Filename With Extension Using Get-Item
The Get-Item
cmdlet retrieves detailed information about the specified item, including its directory, mode, last write time, length, and name. To extract the filename with the extension, you can use the .Name
property in conjunction with Get-Item
.
Let’s demonstrate this with a specific path, C:\pc\test_folder\hello.txt
:
(Get-Item C:\pc\test_folder\hello.txt).Name
Output:
hello.txt
Obtain Filename Without Extension Using Get-Item
If you need to extract only the filename without the extension, you can utilize the .BaseName
property in combination with Get-Item
. This allows you to isolate just the filename from the provided path:
(Get-Item C:\pc\test_folder\hello.txt).BaseName
Output:
hello
Apply the Method With Get-ChildItem
This approach is also applicable when using the Get-ChildItem
cmdlet, which retrieves information about the items in a specified location. Here’s how you can use it to extract the filename with or without the extension:
(Get-ChildItem C:\pc\test_folder\hello.txt).Name
(Get-ChildItem C:\pc\test_folder\hello.txt).BaseName
Output:
hello.txt
hello
Use Regular Expressions to Extract the Filename From a Path in PowerShell
Regular expressions can also be utilized to extract the filename from a path in PowerShell. The Regex
class in .NET provides powerful tools for pattern matching and manipulation.
Let’s see how this works:
# Define the path
$path = "C:\pc\test_folder\hello.txt"
# Use regular expression to extract the filename
$filename = [System.Text.RegularExpressions.Regex]::Match($path, '\\([^\\]+)$').Groups[1].Value
$filename
Output:
hello.txt
In this example, we utilize a regular expression to capture the text after the last backslash, which represents the filename along with its extension.
The regular expression '\\([^\\]+)$'
can be broken down as follows:
\\
: Matches a backslash in the path.([^\\]+)
: Captures one or more characters that are not a backslash (representing the filename with extension) within a capturing group.$
: Specifies that the match should occur at the end of the string.
By using the Match
method from the Regex
class, we apply the regular expression to the path.
The resulting match contains the filename with its extension. We access this through Groups[1].Value
to extract the desired filename.
Use String Manipulation to Extract the Filename From a Path in PowerShell
String manipulation techniques can also be employed to extract the filename from a path. For instance, you can use the LastIndexOf
method to find the last occurrence of the backslash and extract the filename accordingly.
# Define the path
$path = "C:\pc\test_folder\hello.txt"
# Find the index of the last backslash
$lastBackslashIndex = $path.LastIndexOf("\") + 1
# Extract the filename
$filename = $path.Substring($lastBackslashIndex)
$filename
Output:
hello.txt
Here, we utilize the LastIndexOf
method to determine the index of the last occurrence of a backslash (\
) in the path, essentially identifying the position where the filename begins.
$lastBackslashIndex = $path.LastIndexOf("\") + 1
: This line calculates the index of the last backslash and adds1
to ensure we start extracting characters after the backslash.
Next, we utilize the Substring
method to extract the filename, starting from the index after the last backslash and continuing to the end of the string.
$filename = $path.Substring($lastBackslashIndex)
: Here, we extract the filename by utilizingSubstring
with the calculated index.
Use the System.IO.FileInfo
Class to Extract the Filename From a Path in PowerShell
The System.IO.FileInfo
class provides properties to access file information, including the file name. We can create a FileInfo
object and then access the Name
property to obtain the filename.
# Define the path
$path = "C:\pc\test_folder\hello.txt"
# Create a FileInfo object
$fileInfo = New-Object System.IO.FileInfo $path
# Extract the filename
$filename = $fileInfo.Name
$filename
Output:
hello.txt
In this example, we create a FileInfo
object using New-Object
and the System.IO.FileInfo
class, allowing us to access file-related properties and methods.
$fileInfo = New-Object System.IO.FileInfo $path
: This line of code constructs aFileInfo
object based on the provided path.
Next, we utilize the Name
property of the FileInfo
object to extract the filename.
$filename = $fileInfo.Name
: Here, we retrieve the filename by accessing theName
property of theFileInfo
object.
Conclusion
In this tutorial, we explored various methods to extract file names from paths in PowerShell. From using built-in cmdlets like Split-Path
to employing regular expressions and string manipulation, you have a range of options to choose from based on your specific requirements.
Understanding these techniques will enhance your proficiency in handling file paths and extracting essential file information in PowerShell. Whether you prefer the simplicity of built-in cmdlets or the versatility of regular expressions, PowerShell offers a robust set of tools for effective file manipulation.