Undefined Index With PHP Post
- Reproduce the Undefined Index Error
-
Use
isset()
to Check the Content of$_POST
-
Use
array_key_exists()
to Search the Keys in$_POST
-
Use
in_array
to Check if a Value Exists in$_POST
-
Use Null Coalescing Operator (
??
)
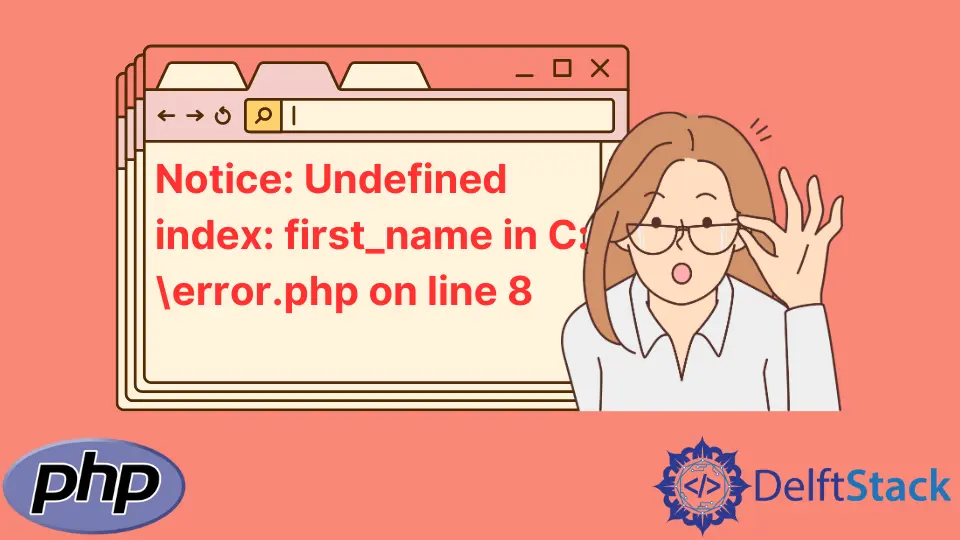
This article teaches four solutions to undefined index
in PHP $_POST
. The solutions will use isset()
, array_key_exists()
, in_array()
, and the Null coalescing operator.
First, we’ll reproduce the error before explaining the solutions. Meanwhile, in PHP 8
, undefined index
is the same as undefined array key
.
Reproduce the Undefined Index Error
To reproduce the error, download XAMPP
or WAMP
and create a working directory in htdocs
. Save the following HTML form in your working directory as sample-html-form.html
.
The form has two input fields that accept a user’s first and last names. You’ll also note that we’ve set the form’s action attribute to 0-reproduce-the-error.php
.
Code - sample-html-form.html
:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>Sample HTML Form</title>
<style type="text/css">
body { height: 100vh; display: grid; place-items: center; }
main { display: grid; outline: 3px solid #1a1a1a; width: 50%; padding: 0.5em; }
form { align-self: center; justify-self: center; width: 50%;}
input:not([type="submit"]) { width: 50%; }
input[type="submit"] { padding: 0.5em;}
.form-row { display: flex; justify-content: space-between; margin-bottom: 1rem; }
.form-row:last-child { justify-content: center; }
</style>
</head>
<body>
<main>
<!--
This is a sample form that demonstrates how
to fix the undefined index in post when using
PHP. You can replace the value of the "action"
attribute with any of the PHP code in
this article.
- DelftStack.com
-->
<form method="post" action="0-reproduce-the-error.php">
<div class="form-row">
<label id="first_name">First name</label>
<input name="first_name" type="text" required>
</div>
<div class="form-row">
<label id="last_name">Last name</label>
<input type="text" name="last_name" required>
</div>
<div class="form-row">
<input type="submit" name="submit_bio_data" value="Submit">
</div>
</form>
</main>
</body>
</html>
Code - 0-reproduce-the-error.php
:
<?php
// The purpose of this script is to reproduce
// the undefined index or undefined array
// key error messages. Also, it requires
// that the $_POST array does not contain
// the first_name and last_name indexes.
// DO NOT USE THIS CODE ON PRODUCTION SERVERS.
$first_name = $_POST['first_name'];
$last_name = $_POST['last_name'];
if ($first_name && $last_name) {
echo "Your first name is " . htmlspecialchars($first_name);
echo "Your last name is " . htmlspecialchars($last_name);
}
?>
Now, open the HTML form in your web browser. Then type empty strings into the input fields and hit the submit button.
In PHP 5
, you’ll get an output like the one shown in the following image. The image shows that undefined index
is a Notice
in PHP 5
.
Output:
If you use PHP 8
, undefined index
becomes undefined array key
. Also, unlike PHP 5
, undefined array key
is a Warning
in PHP 8
.
Output:
The next thing is to show you different ways that will solve it.
Use isset()
to Check the Content of $_POST
With isset()
, you can check $_POST
before using any of its contents. First, set up an if...else
statement that uses isset()
in its conditional check.
The code in the if
block should only execute if isset()
returns true
. By doing this, you’ll prevent the undefined index
error.
In the code below, we are checking if the name attributes of the HTML form exist in $_POST
. Also, we ensure the user has not entered empty strings.
If the check returns true
, we print the user-supplied data. Save the PHP code as 1-fix-with-isset.php
in your working directory and link it with the HTML form.
Code - 1-fix-with-isset.php
:
<?php
// It's customary to check that the user clicked
// the submit button.
if (isset($_POST['submit_bio_data']) && !empty($_POST)) {
// In the following "if" statement, we check for
// the following:
// 1. The existence of the first_name last_name
// in $_POST.
// 2. Prevent the user from supplying an empty
// string.
if (isset($_POST['first_name'], $_POST['last_name'])
&& !empty(trim($_POST['first_name']))
&& !empty(trim($_POST['last_name'])))
{
echo "Your first name is " . htmlspecialchars($_POST['first_name']);
echo "Your last name is " . htmlspecialchars($_POST['last_name']);
} else {
// If the user sees the following message, that
// means either first_name or last_name is not
// in the $_POST array.
echo "Please fill all the form fields";
}
} else {
echo "An unexpected error occurred. Please, try again.";
}
?>
Output (when the user supplies empty values):
Use array_key_exists()
to Search the Keys in $_POST
The array_key_exists()
as its name implies will search for a key in $_POST
. In our case, these keys are the name attributes of the HTML form inputs.
We’ll only process the form if array_key_exists()
find the name attributes in $_POST
. To be safe, we use the empty()
function to ensure the user entered some data.
You’ll find all these in the following code. To test the code, save it as 2-fix-with-array-key-exists.php
in your working directory, and link it with your HTML form by updating the value of the action
attribute.
Now, do the following steps.
- Open the HTML file in your code editor.
- Update the
name
attribute ofFirst name
input tofirst_nam
(we deleted the last “e”). - Switch to your Web browser and fill out the first and last names.
An error will occur when you fill the form with the correct data. That’s because there is a mismatch between first_nam
and first_name
.
The PHP script expected the latter, but it got the former. Without the error checking, you’ll get the undefined index
or undefined array key
.
Code - 2-fix-with-array-key-exists.php
:
<?php
// Ensure the user clicked the submit button.
if (isset($_POST['submit_bio_data']) && !empty($_POST)) {
// Use array_key_exists to check for the
// first_name and last_name fields. At the
// same time, prevent the user from supplying
// an empty string. You can also implement other
// validation checks depending on your use case.
if (array_key_exists('first_name', $_POST) && array_key_exists('last_name', $_POST)
&& !empty(trim($_POST['first_name']))
&& !empty(trim($_POST['last_name']))
)
{
echo "Your first name is " . htmlspecialchars($_POST['first_name']) . "<br />";
echo "Your last name is " . htmlspecialchars($_POST['last_name']);
} else {
echo "Please fill all the form fields";
}
} else {
echo "An unexpected error occurred. Please, try again.";
}
?>
Output (pay attention to the highlighted part in DevTools
):
Use in_array
to Check if a Value Exists in $_POST
PHP in_array()
function can search $_POST
for a specific value. Knowing this, we can use it to confirm if the user filled the HTML form.
Together with the empty()
function, we can ensure the user did not supply empty strings. Both the searching and emptiness check prevents undefined index
or undefined array key
.
In the example detailed below, we use in_array
and empty()
to prevent the undefined index
error. Save the PHP code as 3-fix-with-in-array.php
, then set the action
attribute of the HTML with the name of the PHP file.
Open the HTML form, fill the Last name
field, and leave the First name
blank. Submit the form, and you’ll get the custom error message because in_array
did not find the first name in $_POST
.
Code - 3-fix-with-in-array.php
:
<?php
// Ensure the user clicked the submit button.
if (isset($_POST['submit_bio_data']) && !empty($_POST)) {
// Use in_array to check for the first and last
// name fields. Also, check for empty submissions
// by the user. We advise that you implement other
// checks to suit your use case.
if (in_array('first_name', $_POST) && in_array('last_name', $_POST)
&& !empty(trim($_POST['first_name']))
&& !empty(trim($_POST['last_name']))
)
{
echo "Your first name is " . htmlspecialchars($_POST['first_name']) . "<br />";
echo "Your last name is " . htmlspecialchars($_POST['last_name']);
} else {
echo "Please fill all the form fields";
}
} else {
echo "An unexpected error occurred. Please, try again.";
}
?>
Output:
Use Null Coalescing Operator (??
)
The null coalescing operator (??
) will return the operand on its left side if it exists. Otherwise, it’ll return the operand on the right.
Armed with this knowledge, we can pass an input value to the Null coalescing operator. As a result, if the value exists, the operator will return it; otherwise, we’ll make it return another value.
In the code below, we’ll check if the Null coalescing operator returned this other value. If it does, that means one of the form inputs is not in $_POST
.
With this, we can show a custom error message other than undefined index
or undefined array key
. Save the code as 4-fix-with-null-coalescing-operator.php
and link it with your HTML form.
Then, open your web browser and fill out the form with the correct data. You’ll get no error messages when you submit the form.
Code - 4-fix-with-null-coalescing-operator.php
:
<?php
// It's customary to check that the user clicked
// the submit button.
if (isset($_POST['submit_bio_data']) && !empty($_POST)) {
// This works in PHP 7 and above. It's called
// the null coalescing operator. It'll return
// the value on the left if it exists or the
// value on the right if it does not. While
// By doing this, we ensure the user does not
// enter empty strings. What's more, you can
// implement other checks for your use case.
$first_name = !empty(trim($_POST['first_name'])) ?? 'Missing';
$last_name = !empty(trim($_POST['last_name'])) ?? 'Missing';
// if the null coalescing operator returns
// the string "Missing" for first_name
// or last_name, that means they are not valid
// indexes of the $_POST array.
if ($first_name && $last_name !== 'Missing') {
echo "Your first name is " . htmlspecialchars($_POST['first_name']) . "<br />";
echo "Your last name is " . htmlspecialchars($_POST['last_name']);
} else {
// If the user sees the following message, that
// means either first_name or last_name is not
// in the $_POST array.
echo "Please fill all the form fields";
}
} else {
echo "An unexpected error occurred. Please, try again.";
}
?>
Output:
Habdul Hazeez is a technical writer with amazing research skills. He can connect the dots, and make sense of data that are scattered across different media.
LinkedIn